mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-14 05:58:19 +00:00
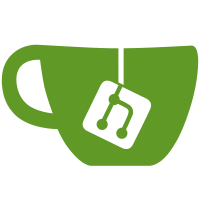
* Add database migrations, still need to update the API usage... * gofmt goimports * progress * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * use slot instead of index * rename LastArchivedIndex to LastArchivedSlot * rename LastArchivedIndexRoot to LastArchivedRoot * remove unused HighestSlotStates method * deprecate old key, include in migration * deprecate old key, include in migration * remove blocks index in migration * rename bucket variable * fix code to pass tests * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * gofmt, goimports * fix * Add state slot index * progress * lint * fix build * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration * kafka * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * remove SaveArchivedPointRoot, a few other big changes * Merge branch 'index-migration' of github.com:prysmaticlabs/prysm into index-migration * fix tests and lint * lint again * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * block migration, some renaming * gaz, gofmt * add tests * change index to uint bytes * Merge branch 'index-migration' of github.com:prysmaticlabs/prysm into index-migration * rm method notes * stop if the bucket doesn't exist * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * @rauljordan pr feedback * Simplify * Merge refs/heads/master into index-migration * Remove unused method, add roundtrip test * gofmt * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge refs/heads/master into index-migration * Merge branch 'master' of github.com:prysmaticlabs/prysm into index-migration
81 lines
1.5 KiB
Go
81 lines
1.5 KiB
Go
package kv
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
)
|
|
|
|
func TestArchivedPointIndexRoot_CanSaveRetrieve(t *testing.T) {
|
|
db := setupDB(t)
|
|
ctx := context.Background()
|
|
i1 := uint64(100)
|
|
r1 := [32]byte{'A'}
|
|
|
|
received := db.ArchivedPointRoot(ctx, i1)
|
|
if r1 == received {
|
|
t.Fatal("Should not have been saved")
|
|
}
|
|
st := testutil.NewBeaconState()
|
|
if err := st.SetSlot(i1); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if err := db.SaveState(ctx, st, r1); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
received = db.ArchivedPointRoot(ctx, i1)
|
|
if r1 != received {
|
|
t.Error("Should have been saved")
|
|
}
|
|
}
|
|
|
|
func TestLastArchivedPoint_CanRetrieve(t *testing.T) {
|
|
db := setupDB(t)
|
|
ctx := context.Background()
|
|
i, err := db.LastArchivedSlot(ctx)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if i != 0 {
|
|
t.Error("Did not get correct index")
|
|
}
|
|
|
|
st := testutil.NewBeaconState()
|
|
if err := db.SaveState(ctx, st, [32]byte{'A'}); err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
if db.LastArchivedRoot(ctx) != [32]byte{'A'} {
|
|
t.Error("Did not get wanted root")
|
|
}
|
|
|
|
if err := st.SetSlot(2); err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
if err := db.SaveState(ctx, st, [32]byte{'B'}); err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
if db.LastArchivedRoot(ctx) != [32]byte{'B'} {
|
|
t.Error("Did not get wanted root")
|
|
}
|
|
|
|
if err := st.SetSlot(3); err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
if err := db.SaveState(ctx, st, [32]byte{'C'}); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
i, err = db.LastArchivedSlot(ctx)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if i != 3 {
|
|
t.Error("Did not get correct index")
|
|
}
|
|
}
|