mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
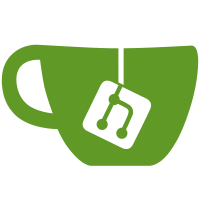
* beacon-chain/core/blocks/spectest tests updated * beacon-chain/core/blocks tests updated * beacon-chain/core/epoch tests updated * Merge branch 'master' into apply-testutils-assertions-to-core * beacon-chain/core/helpers tests updated * beacon-chain/core/validators tests updated * beacon-chain/core/state/spectest tests updated * beacon-chain/core/state tests updated * Merge refs/heads/master into apply-testutils-assertions-to-core * Merge refs/heads/master into apply-testutils-assertions-to-core * Merge refs/heads/master into apply-testutils-assertions-to-core * Merge refs/heads/master into apply-testutils-assertions-to-core * Merge refs/heads/master into apply-testutils-assertions-to-core
113 lines
2.7 KiB
Go
113 lines
2.7 KiB
Go
package helpers_test
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
beaconstate "github.com/prysmaticlabs/prysm/beacon-chain/state"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
)
|
|
|
|
func TestBlockRootAtSlot_CorrectBlockRoot(t *testing.T) {
|
|
var blockRoots [][]byte
|
|
|
|
for i := uint64(0); i < params.BeaconConfig().SlotsPerHistoricalRoot; i++ {
|
|
blockRoots = append(blockRoots, []byte{byte(i)})
|
|
}
|
|
s := &pb.BeaconState{
|
|
BlockRoots: blockRoots,
|
|
}
|
|
|
|
tests := []struct {
|
|
slot uint64
|
|
stateSlot uint64
|
|
expectedRoot [32]byte
|
|
}{
|
|
{
|
|
slot: 0,
|
|
stateSlot: 1,
|
|
expectedRoot: [32]byte{0},
|
|
},
|
|
{
|
|
slot: 2,
|
|
stateSlot: 5,
|
|
expectedRoot: [32]byte{2},
|
|
},
|
|
{
|
|
slot: 64,
|
|
stateSlot: 128,
|
|
expectedRoot: [32]byte{64},
|
|
}, {
|
|
slot: 2999,
|
|
stateSlot: 3000,
|
|
expectedRoot: [32]byte{183},
|
|
}, {
|
|
slot: 2873,
|
|
stateSlot: 3000,
|
|
expectedRoot: [32]byte{57},
|
|
},
|
|
{
|
|
slot: 0,
|
|
stateSlot: params.BeaconConfig().SlotsPerHistoricalRoot,
|
|
expectedRoot: [32]byte{},
|
|
},
|
|
}
|
|
for i, tt := range tests {
|
|
t.Run(fmt.Sprintf("%d", i), func(t *testing.T) {
|
|
s.Slot = tt.stateSlot
|
|
state, err := beaconstate.InitializeFromProto(s)
|
|
require.NoError(t, err)
|
|
wantedSlot := tt.slot
|
|
result, err := helpers.BlockRootAtSlot(state, wantedSlot)
|
|
require.NoError(t, err, "Failed to get block root at slot %d", wantedSlot)
|
|
assert.DeepEqual(t, tt.expectedRoot[:], result, "Result block root was an unexpected value")
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestBlockRootAtSlot_OutOfBounds(t *testing.T) {
|
|
var blockRoots [][]byte
|
|
|
|
for i := uint64(0); i < params.BeaconConfig().SlotsPerHistoricalRoot; i++ {
|
|
blockRoots = append(blockRoots, []byte{byte(i)})
|
|
}
|
|
state := &pb.BeaconState{
|
|
BlockRoots: blockRoots,
|
|
}
|
|
|
|
tests := []struct {
|
|
slot uint64
|
|
stateSlot uint64
|
|
expectedErr string
|
|
}{
|
|
{
|
|
slot: 1000,
|
|
stateSlot: 500,
|
|
expectedErr: "slot 1000 out of bounds",
|
|
},
|
|
{
|
|
slot: 3000,
|
|
stateSlot: 3000,
|
|
expectedErr: "slot 3000 out of bounds",
|
|
},
|
|
{
|
|
// Edge case where stateSlot is over slots per historical root and
|
|
// slot is not within (stateSlot - HistoricalRootsLimit, statSlot]
|
|
slot: 1,
|
|
stateSlot: params.BeaconConfig().SlotsPerHistoricalRoot + 2,
|
|
expectedErr: "slot 1 out of bounds",
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
state.Slot = tt.stateSlot
|
|
s, err := beaconstate.InitializeFromProto(state)
|
|
require.NoError(t, err)
|
|
_, err = helpers.BlockRootAtSlot(s, tt.slot)
|
|
assert.ErrorContains(t, tt.expectedErr, err)
|
|
}
|
|
}
|