mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
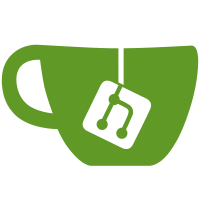
* add context to beacon APIs * add TODO to merge GET and POST methods * fix linter action Co-authored-by: kasey <489222+kasey@users.noreply.github.com> Co-authored-by: james-prysm <90280386+james-prysm@users.noreply.github.com>
120 lines
3.3 KiB
Go
120 lines
3.3 KiB
Go
package beacon_api
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"net/http"
|
|
"testing"
|
|
|
|
"github.com/golang/mock/gomock"
|
|
"github.com/prysmaticlabs/prysm/v3/api/gateway/apimiddleware"
|
|
ethpb "github.com/prysmaticlabs/prysm/v3/proto/prysm/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/v3/testing/assert"
|
|
"github.com/prysmaticlabs/prysm/v3/validator/client/beacon-api/mock"
|
|
)
|
|
|
|
func TestProposeBeaconBlock_Error(t *testing.T) {
|
|
testSuites := []struct {
|
|
name string
|
|
expectedErrorMessage string
|
|
expectedHttpError *apimiddleware.DefaultErrorJson
|
|
}{
|
|
{
|
|
name: "error 202",
|
|
expectedErrorMessage: "block was successfully broadcasted but failed validation",
|
|
expectedHttpError: &apimiddleware.DefaultErrorJson{
|
|
Code: http.StatusAccepted,
|
|
Message: "202 error",
|
|
},
|
|
},
|
|
{
|
|
name: "request failed",
|
|
expectedErrorMessage: "failed to send POST data to REST endpoint",
|
|
expectedHttpError: nil,
|
|
},
|
|
}
|
|
|
|
testCases := []struct {
|
|
name string
|
|
consensusVersion string
|
|
endpoint string
|
|
block *ethpb.GenericSignedBeaconBlock
|
|
}{
|
|
{
|
|
name: "phase0",
|
|
consensusVersion: "phase0",
|
|
endpoint: "/eth/v1/beacon/blocks",
|
|
block: ðpb.GenericSignedBeaconBlock{
|
|
Block: generateSignedPhase0Block(),
|
|
},
|
|
},
|
|
{
|
|
name: "altair",
|
|
consensusVersion: "altair",
|
|
endpoint: "/eth/v1/beacon/blocks",
|
|
block: ðpb.GenericSignedBeaconBlock{
|
|
Block: generateSignedAltairBlock(),
|
|
},
|
|
},
|
|
{
|
|
name: "bellatrix",
|
|
consensusVersion: "bellatrix",
|
|
endpoint: "/eth/v1/beacon/blocks",
|
|
block: ðpb.GenericSignedBeaconBlock{
|
|
Block: generateSignedBellatrixBlock(),
|
|
},
|
|
},
|
|
{
|
|
name: "blinded bellatrix",
|
|
consensusVersion: "bellatrix",
|
|
endpoint: "/eth/v1/beacon/blinded_blocks",
|
|
block: ðpb.GenericSignedBeaconBlock{
|
|
Block: generateSignedBlindedBellatrixBlock(),
|
|
},
|
|
},
|
|
{
|
|
name: "blinded capella",
|
|
consensusVersion: "capella",
|
|
endpoint: "/eth/v1/beacon/blinded_blocks",
|
|
block: ðpb.GenericSignedBeaconBlock{
|
|
Block: generateSignedBlindedCapellaBlock(),
|
|
},
|
|
},
|
|
}
|
|
|
|
for _, testSuite := range testSuites {
|
|
for _, testCase := range testCases {
|
|
t.Run(testSuite.name+"/"+testCase.name, func(t *testing.T) {
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
|
|
ctx := context.Background()
|
|
jsonRestHandler := mock.NewMockjsonRestHandler(ctrl)
|
|
|
|
headers := map[string]string{"Eth-Consensus-Version": testCase.consensusVersion}
|
|
jsonRestHandler.EXPECT().PostRestJson(
|
|
ctx,
|
|
testCase.endpoint,
|
|
headers,
|
|
gomock.Any(),
|
|
nil,
|
|
).Return(
|
|
testSuite.expectedHttpError,
|
|
errors.New("foo error"),
|
|
).Times(1)
|
|
|
|
validatorClient := &beaconApiValidatorClient{jsonRestHandler: jsonRestHandler}
|
|
_, err := validatorClient.proposeBeaconBlock(ctx, testCase.block)
|
|
assert.ErrorContains(t, testSuite.expectedErrorMessage, err)
|
|
assert.ErrorContains(t, "foo error", err)
|
|
})
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestProposeBeaconBlock_UnsupportedBlockType(t *testing.T) {
|
|
validatorClient := &beaconApiValidatorClient{}
|
|
_, err := validatorClient.proposeBeaconBlock(context.Background(), ðpb.GenericSignedBeaconBlock{})
|
|
assert.ErrorContains(t, "unsupported block type", err)
|
|
}
|