mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
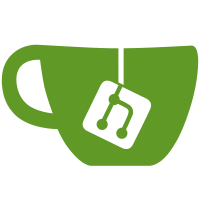
* add REST endpoint for SubmitSignedAggregateSelectionProof * fix linter action * update with context * fix context import Co-authored-by: Radosław Kapka <rkapka@wp.pl>
30 lines
1.1 KiB
Go
30 lines
1.1 KiB
Go
package beacon_api
|
|
|
|
import (
|
|
"bytes"
|
|
"context"
|
|
"encoding/json"
|
|
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/rpc/apimiddleware"
|
|
ethpb "github.com/prysmaticlabs/prysm/v3/proto/prysm/v1alpha1"
|
|
)
|
|
|
|
func (c *beaconApiValidatorClient) submitSignedAggregateSelectionProof(ctx context.Context, in *ethpb.SignedAggregateSubmitRequest) (*ethpb.SignedAggregateSubmitResponse, error) {
|
|
body, err := json.Marshal([]*apimiddleware.SignedAggregateAttestationAndProofJson{jsonifySignedAggregateAndProof(in.SignedAggregateAndProof)})
|
|
if err != nil {
|
|
return nil, errors.Wrap(err, "failed to marshal SignedAggregateAttestationAndProof")
|
|
}
|
|
|
|
if _, err := c.jsonRestHandler.PostRestJson(ctx, "/eth/v1/validator/aggregate_and_proofs", nil, bytes.NewBuffer(body), nil); err != nil {
|
|
return nil, errors.Wrap(err, "failed to send POST data to REST endpoint")
|
|
}
|
|
|
|
attestationDataRoot, err := in.SignedAggregateAndProof.Message.Aggregate.Data.HashTreeRoot()
|
|
if err != nil {
|
|
return nil, errors.Wrap(err, "failed to compute attestation data root")
|
|
}
|
|
|
|
return ðpb.SignedAggregateSubmitResponse{AttestationDataRoot: attestationDataRoot[:]}, nil
|
|
}
|