mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
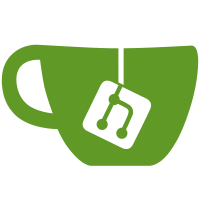
* Fix rename issue * nishant feedback * add test * Update shared/fileutil/fileutil.go Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com> * preston feedback Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
82 lines
2.3 KiB
Go
82 lines
2.3 KiB
Go
// Copyright 2015 The go-ethereum Authors
|
|
// This file is part of go-ethereum.
|
|
//
|
|
// go-ethereum is free software: you can redistribute it and/or modify
|
|
// it under the terms of the GNU General Public License as published by
|
|
// the Free Software Foundation, either version 3 of the License, or
|
|
// (at your option) any later version.
|
|
//
|
|
// go-ethereum is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
// GNU General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with go-ethereum. If not, see <http://www.gnu.org/licenses/>.
|
|
package fileutil_test
|
|
|
|
import (
|
|
"bufio"
|
|
"bytes"
|
|
"io/ioutil"
|
|
"os"
|
|
"os/user"
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/fileutil"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
)
|
|
|
|
func TestPathExpansion(t *testing.T) {
|
|
user, err := user.Current()
|
|
require.NoError(t, err)
|
|
tests := map[string]string{
|
|
"/home/someuser/tmp": "/home/someuser/tmp",
|
|
"~/tmp": user.HomeDir + "/tmp",
|
|
"$DDDXXX/a/b": "/tmp/a/b",
|
|
"/a/b/": "/a/b",
|
|
}
|
|
require.NoError(t, os.Setenv("DDDXXX", "/tmp"))
|
|
for test, expected := range tests {
|
|
expanded, err := fileutil.ExpandPath(test)
|
|
require.NoError(t, err)
|
|
assert.Equal(t, expected, expanded)
|
|
}
|
|
}
|
|
|
|
func TestCopyFile(t *testing.T) {
|
|
fName := testutil.TempDir() + "testfile"
|
|
err := ioutil.WriteFile(fName, []byte{1, 2, 3}, params.BeaconIoConfig().ReadWritePermissions)
|
|
require.NoError(t, err)
|
|
defer func() {
|
|
assert.NoError(t, os.Remove(fName))
|
|
}()
|
|
|
|
err = fileutil.CopyFile(fName, fName+"copy")
|
|
assert.NoError(t, err)
|
|
defer func() {
|
|
assert.NoError(t, os.Remove(fName+"copy"))
|
|
}()
|
|
|
|
assert.Equal(t, true, deepCompare(t, fName, fName+"copy"))
|
|
}
|
|
|
|
func deepCompare(t *testing.T, file1, file2 string) bool {
|
|
sf, err := os.Open(file1)
|
|
assert.NoError(t, err)
|
|
df, err := os.Open(file2)
|
|
assert.NoError(t, err)
|
|
sscan := bufio.NewScanner(sf)
|
|
dscan := bufio.NewScanner(df)
|
|
|
|
for sscan.Scan() && dscan.Scan() {
|
|
if !bytes.Equal(sscan.Bytes(), dscan.Bytes()) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|