mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
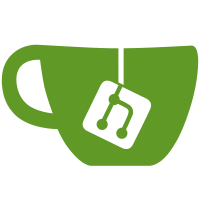
* Merge variable declaration and assignment * Use result of type assertion to simplify cases * Replace call to bytes.Compare with bytes.Equal * Drop unnecessary use of the blank identifier * Replace x.Sub(time.Now()) with time.Until(x) * Function literal can be simplified * Use a single append to concatenate two slices * Replace time.Now().Sub(x) with time.Since(x) * Omit comparison with boolean constant * Omit redundant nil check on slices * Nested if can be replaced with else-if * Function call can be replaced with helper function * Omit redundant control flow * Use plain channel send or receive * Simplify returning boolean expression * Merge branch 'origin-master' into fix-antipatterns * Merge branch 'master' into fix-antipatterns
43 lines
1.1 KiB
Go
43 lines
1.1 KiB
Go
package cmd
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/urfave/cli/v2"
|
|
"github.com/urfave/cli/v2/altsrc"
|
|
)
|
|
|
|
// WrapFlags so that they can be loaded from alternative sources.
|
|
func WrapFlags(flags []cli.Flag) []cli.Flag {
|
|
wrapped := make([]cli.Flag, 0, len(flags))
|
|
for _, f := range flags {
|
|
switch t := f.(type) {
|
|
case *cli.BoolFlag:
|
|
f = altsrc.NewBoolFlag(t)
|
|
case *cli.DurationFlag:
|
|
f = altsrc.NewDurationFlag(t)
|
|
case *cli.GenericFlag:
|
|
f = altsrc.NewGenericFlag(t)
|
|
case *cli.Float64Flag:
|
|
f = altsrc.NewFloat64Flag(t)
|
|
case *cli.IntFlag:
|
|
f = altsrc.NewIntFlag(t)
|
|
case *cli.StringFlag:
|
|
f = altsrc.NewStringFlag(t)
|
|
case *cli.StringSliceFlag:
|
|
f = altsrc.NewStringSliceFlag(t)
|
|
case *cli.Uint64Flag:
|
|
f = altsrc.NewUint64Flag(t)
|
|
case *cli.UintFlag:
|
|
f = altsrc.NewUintFlag(t)
|
|
case *cli.Int64Flag:
|
|
// Int64Flag does not work. See https://github.com/prysmaticlabs/prysm/issues/6478
|
|
panic(fmt.Sprintf("unsupported flag type type %T", f))
|
|
default:
|
|
panic(fmt.Sprintf("cannot convert type %T", f))
|
|
}
|
|
wrapped = append(wrapped, f)
|
|
}
|
|
return wrapped
|
|
}
|