mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
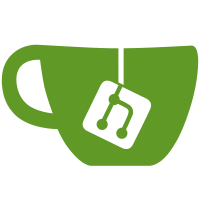
* extract creating DefaultErrorJson objects * do not use default http client for proxying Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
57 lines
1.3 KiB
Go
57 lines
1.3 KiB
Go
package gateway
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// ---------------
|
|
// Error handling.
|
|
// ---------------
|
|
|
|
// ErrorJson describes common functionality of all JSON error representations.
|
|
type ErrorJson interface {
|
|
StatusCode() int
|
|
SetCode(code int)
|
|
Msg() string
|
|
}
|
|
|
|
// DefaultErrorJson is a JSON representation of a simple error value, containing only a message and an error code.
|
|
type DefaultErrorJson struct {
|
|
Message string `json:"message"`
|
|
Code int `json:"code"`
|
|
}
|
|
|
|
// InternalServerErrorWithMessage returns a DefaultErrorJson with 500 code and a custom message.
|
|
func InternalServerErrorWithMessage(err error, message string) *DefaultErrorJson {
|
|
e := errors.Wrapf(err, message)
|
|
return &DefaultErrorJson{
|
|
Message: e.Error(),
|
|
Code: http.StatusInternalServerError,
|
|
}
|
|
}
|
|
|
|
// InternalServerError returns a DefaultErrorJson with 500 code.
|
|
func InternalServerError(err error) *DefaultErrorJson {
|
|
return &DefaultErrorJson{
|
|
Message: err.Error(),
|
|
Code: http.StatusInternalServerError,
|
|
}
|
|
}
|
|
|
|
// StatusCode returns the error's underlying error code.
|
|
func (e *DefaultErrorJson) StatusCode() int {
|
|
return e.Code
|
|
}
|
|
|
|
// Msg returns the error's underlying message.
|
|
func (e *DefaultErrorJson) Msg() string {
|
|
return e.Message
|
|
}
|
|
|
|
// SetCode sets the error's underlying error code.
|
|
func (e *DefaultErrorJson) SetCode(code int) {
|
|
e.Code = code
|
|
}
|