mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
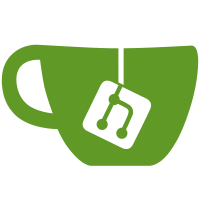
* Update seed domains (#3872) * Remove Transfers (#3870) * Remove active index roots and compact committee roots (#3869) * Update inclusion reward (#3886) * Alter proposer selection logic (#3884) * Fix early committee bias (#3888) * Remove shards and committees (#3896) * Epoch spec tests v0.9 (#3907) * Block spec test v0.9 (#3905) * rm'ed in protobuf * build proto * build proto * build proto * fix core package * Gazelle * Fixed all the tests * Fixed static test * Comment out spec test for now * One more skip * fix-roundRobinSync (#3862) * Starting but need new seed function * Revert initial sync * Updated Proposer Slashing * Fixed all tests * Lint * Update inclusion reward * Fill randao mixes with eth1 data hash * Test * Fixing test part1 * All tests passing * One last test * Updated config * Build proto * Proper skip message * Conflict and fmt * Removed crosslinks and shards. Built * Format and gazelle * Fixed all the block package tests * Fixed all the helper tests * All epoch package tests pass * All core package tests pass * Fixed operation tests * Started fixing rpc test * RPC tests passed! * Fixed all init sync tests * All tests pass * Fixed blockchain tests * Lint * Lint * Preston's feedback * Starting * Remove container * Fixed block spec tests * All passing except for block_processing test * Failing block processing test * Starting * Add AggregateAndProof * All mainnet test passes * Update deposit contract (#3906) * Proto spec tests v0.9 (#3908) * Starting * Add AggregateAndProof * Unskip block util tests (#3910) * rm'ed in protobuf * build proto * build proto * build proto * fix core package * Gazelle * Fixed all the tests * Fixed static test * Comment out spec test for now * One more skip * fix-roundRobinSync (#3862) * Starting but need new seed function * Revert initial sync * Updated Proposer Slashing * Fixed all tests * Lint * Update inclusion reward * Fill randao mixes with eth1 data hash * Test * Fixing test part1 * All tests passing * One last test * Updated config * Build proto * Proper skip message * Conflict and fmt * Removed crosslinks and shards. Built * Format and gazelle * Fixed all the block package tests * Fixed all the helper tests * All epoch package tests pass * All core package tests pass * Fixed operation tests * Started fixing rpc test * RPC tests passed! * Fixed all init sync tests * All tests pass * Fixed blockchain tests * Lint * Lint * Preston's feedback * Starting * Remove container * Fixed block spec tests * All passing except for block_processing test * Failing block processing test * Starting * Add AggregateAndProof * All mainnet test passes * Unskip block util tests * Slot processing spec test V0.9 (#3912) * Starting * Add AggregateAndProof * Unskip slot processing mainnet test * Unskip minimal spec test for finalization (#3920) * Remove outdated interop tests (#3922) * Rm outdated interop tests * Rm test runner * Gazelle * Update validator to use proposer slot (#3919) * Fix committee assignment (#3931) * Replace shard with committee index (#3930) * Conflict * Clean up (#3933) * Remove shard filter in db (#3936) * Remove lightouse compatibility test (#3939) * Update Committee Cache for v0.9 (#3948) * Updated committee cache * Removed shuffled indices cache * Started testing run time * Lint * Fixed test * Safeguard against nil head state * address edge case * add test * Fixed TestRoundRobinSync by doubling the epochs * Unskip TestProtoCompatability (#3958) * Unskip TestProtoCompatability * Update WORKSPACE * Fix minimal config (#3959) * fix minimal configs * fix hardcoded value in test * Simplify verify att time (#3961) * update readme for deposit contract, regen bindings for vyper 0.1.0b12 (#3963) * update readme for deposit contract, regen bindings * medium * Check nil base state (#3964) * Copy Block When Receiving it From Sync (#3966) * copy block * clone for other service methods too * Change logging of Bitfield (#3956) * change logging of bits * preston's review * Unskip Beacon Server Test (#3962) * run test till the end * fix up proto message types * fmt * resolve broken tests * better error handling * fixing new logic to use archived proposer info * fix up logic * clip using the max effective balance * broken build fix with num arg mismatch * amend archive * archival logic changed * rename test * archive both proposer and attester seeds * page size 100 * further experiments * further experimentation, archivedProposerIndex seems wrong * test passes * rem log * fix broken test * fix test * gaz * fix imports * ethapis
410 lines
12 KiB
Go
410 lines
12 KiB
Go
package testutil
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/golang/protobuf/proto"
|
|
"github.com/prysmaticlabs/go-bitfield"
|
|
"github.com/prysmaticlabs/go-ssz"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/blocks"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/state"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/bls"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
)
|
|
|
|
// BlockGenConfig is used to define the requested conditions
|
|
// for block generation.
|
|
type BlockGenConfig struct {
|
|
MaxProposerSlashings uint64
|
|
MaxAttesterSlashings uint64
|
|
MaxAttestations uint64
|
|
MaxDeposits uint64
|
|
MaxVoluntaryExits uint64
|
|
}
|
|
|
|
// DefaultBlockGenConfig returns the block config that utilizes the
|
|
// current params in the beacon config.
|
|
func DefaultBlockGenConfig() *BlockGenConfig {
|
|
return &BlockGenConfig{
|
|
MaxProposerSlashings: params.BeaconConfig().MaxProposerSlashings,
|
|
MaxAttesterSlashings: params.BeaconConfig().MaxAttesterSlashings,
|
|
MaxAttestations: params.BeaconConfig().MaxAttestations,
|
|
MaxDeposits: params.BeaconConfig().MaxDeposits,
|
|
MaxVoluntaryExits: params.BeaconConfig().MaxVoluntaryExits,
|
|
}
|
|
}
|
|
|
|
// GenerateFullBlock generates a fully valid block with the requested parameters.
|
|
// Use BlockGenConfig to declare the conditions you would like the block generated under.
|
|
func GenerateFullBlock(
|
|
t testing.TB,
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
conf *BlockGenConfig,
|
|
slot uint64,
|
|
) *ethpb.BeaconBlock {
|
|
|
|
currentSlot := bState.Slot
|
|
if currentSlot > slot {
|
|
t.Fatalf("Current slot in state is larger than given slot. %d > %d", currentSlot, slot)
|
|
}
|
|
|
|
pSlashings := []*ethpb.ProposerSlashing{}
|
|
if conf.MaxProposerSlashings > 0 {
|
|
pSlashings = generateProposerSlashings(t, bState, privs, conf.MaxProposerSlashings)
|
|
}
|
|
|
|
aSlashings := []*ethpb.AttesterSlashing{}
|
|
if conf.MaxAttesterSlashings > 0 {
|
|
aSlashings = generateAttesterSlashings(t, bState, privs, conf.MaxAttesterSlashings)
|
|
}
|
|
|
|
atts := []*ethpb.Attestation{}
|
|
if conf.MaxAttestations > 0 {
|
|
atts = generateAttestations(t, bState, privs, conf.MaxAttestations)
|
|
}
|
|
|
|
newDeposits, eth1Data := []*ethpb.Deposit{}, bState.Eth1Data
|
|
if conf.MaxDeposits > 0 {
|
|
newDeposits, eth1Data = generateDepositsAndEth1Data(t, bState, conf.MaxDeposits)
|
|
}
|
|
|
|
exits := []*ethpb.VoluntaryExit{}
|
|
if conf.MaxVoluntaryExits > 0 {
|
|
exits = generateVoluntaryExits(t, bState, privs, conf.MaxVoluntaryExits)
|
|
}
|
|
|
|
newHeader := proto.Clone(bState.LatestBlockHeader).(*ethpb.BeaconBlockHeader)
|
|
prevStateRoot, err := ssz.HashTreeRoot(bState)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
newHeader.StateRoot = prevStateRoot[:]
|
|
parentRoot, err := ssz.SigningRoot(newHeader)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
// Temporarily incrementing the beacon state slot here since BeaconProposerIndex is a
|
|
// function deterministic on beacon state slot.
|
|
bState.Slot = slot
|
|
reveal, err := CreateRandaoReveal(bState, helpers.CurrentEpoch(bState), privs)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
bState.Slot = currentSlot
|
|
|
|
block := ðpb.BeaconBlock{
|
|
Slot: slot,
|
|
ParentRoot: parentRoot[:],
|
|
Body: ðpb.BeaconBlockBody{
|
|
Eth1Data: eth1Data,
|
|
RandaoReveal: reveal,
|
|
ProposerSlashings: pSlashings,
|
|
AttesterSlashings: aSlashings,
|
|
Attestations: atts,
|
|
VoluntaryExits: exits,
|
|
Deposits: newDeposits,
|
|
},
|
|
}
|
|
|
|
s, err := state.CalculateStateRoot(context.Background(), bState, block)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
root, err := ssz.HashTreeRoot(s)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
block.StateRoot = root[:]
|
|
blockRoot, err := ssz.SigningRoot(block)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
// Temporarily incrementing the beacon state slot here since BeaconProposerIndex is a
|
|
// function deterministic on beacon state slot.
|
|
bState.Slot = slot
|
|
proposerIdx, err := helpers.BeaconProposerIndex(bState)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
bState.Slot = currentSlot
|
|
domain := helpers.Domain(bState.Fork, helpers.CurrentEpoch(bState), params.BeaconConfig().DomainBeaconProposer)
|
|
block.Signature = privs[proposerIdx].Sign(blockRoot[:], domain).Marshal()
|
|
|
|
return block
|
|
}
|
|
|
|
func generateProposerSlashings(
|
|
t testing.TB,
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
maxSlashings uint64,
|
|
) []*ethpb.ProposerSlashing {
|
|
currentSlot := bState.Slot
|
|
currentEpoch := helpers.CurrentEpoch(bState)
|
|
slotsPerEpoch := params.BeaconConfig().SlotsPerEpoch
|
|
|
|
validatorCount, err := helpers.ActiveValidatorCount(bState, currentEpoch)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
proposerSlashings := make([]*ethpb.ProposerSlashing, maxSlashings)
|
|
for i := uint64(0); i < maxSlashings; i++ {
|
|
proposerIndex := i + uint64(validatorCount/4)
|
|
header1 := ðpb.BeaconBlockHeader{
|
|
Slot: currentSlot - (i % slotsPerEpoch),
|
|
BodyRoot: []byte{0, 1, 0},
|
|
}
|
|
root, err := ssz.SigningRoot(header1)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
domain := helpers.Domain(bState.Fork, currentEpoch, params.BeaconConfig().DomainBeaconProposer)
|
|
header1.Signature = privs[proposerIndex].Sign(root[:], domain).Marshal()
|
|
|
|
header2 := ðpb.BeaconBlockHeader{
|
|
Slot: currentSlot - (i % slotsPerEpoch),
|
|
BodyRoot: []byte{0, 2, 0},
|
|
}
|
|
root, err = ssz.SigningRoot(header2)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
header2.Signature = privs[proposerIndex].Sign(root[:], domain).Marshal()
|
|
|
|
slashing := ðpb.ProposerSlashing{
|
|
ProposerIndex: proposerIndex,
|
|
Header_1: header1,
|
|
Header_2: header2,
|
|
}
|
|
proposerSlashings[i] = slashing
|
|
}
|
|
return proposerSlashings
|
|
}
|
|
|
|
func generateAttesterSlashings(
|
|
t testing.TB,
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
maxSlashings uint64,
|
|
) []*ethpb.AttesterSlashing {
|
|
attesterSlashings := make([]*ethpb.AttesterSlashing, maxSlashings)
|
|
for i := uint64(0); i < maxSlashings; i++ {
|
|
committee, err := helpers.BeaconCommittee(bState, i, i%params.BeaconConfig().MaxCommitteesPerSlot)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
committeeSize := uint64(len(committee))
|
|
attData1 := ðpb.AttestationData{
|
|
Target: ðpb.Checkpoint{
|
|
Epoch: i,
|
|
Root: params.BeaconConfig().ZeroHash[:],
|
|
},
|
|
Source: ðpb.Checkpoint{
|
|
Epoch: i + 1,
|
|
Root: params.BeaconConfig().ZeroHash[:],
|
|
},
|
|
}
|
|
aggregationBits := bitfield.NewBitlist(committeeSize)
|
|
aggregationBits.SetBitAt(i, true)
|
|
custodyBits := bitfield.NewBitlist(committeeSize)
|
|
att1 := ðpb.Attestation{
|
|
Data: attData1,
|
|
CustodyBits: custodyBits,
|
|
AggregationBits: aggregationBits,
|
|
}
|
|
dataRoot, err := ssz.HashTreeRoot(&pb.AttestationDataAndCustodyBit{
|
|
Data: att1.Data,
|
|
CustodyBit: false,
|
|
})
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
domain := helpers.Domain(bState.Fork, i, params.BeaconConfig().DomainBeaconAttester)
|
|
sig := privs[committee[i]].Sign(dataRoot[:], domain)
|
|
att1.Signature = bls.AggregateSignatures([]*bls.Signature{sig}).Marshal()
|
|
|
|
attData2 := ðpb.AttestationData{
|
|
Target: ðpb.Checkpoint{
|
|
Epoch: i,
|
|
Root: params.BeaconConfig().ZeroHash[:],
|
|
},
|
|
Source: ðpb.Checkpoint{
|
|
Epoch: i,
|
|
Root: params.BeaconConfig().ZeroHash[:],
|
|
},
|
|
}
|
|
att2 := ðpb.Attestation{
|
|
Data: attData2,
|
|
CustodyBits: custodyBits,
|
|
AggregationBits: aggregationBits,
|
|
}
|
|
dataRoot, err = ssz.HashTreeRoot(&pb.AttestationDataAndCustodyBit{
|
|
Data: att2.Data,
|
|
CustodyBit: false,
|
|
})
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
sig = privs[committee[i]].Sign(dataRoot[:], domain)
|
|
att2.Signature = bls.AggregateSignatures([]*bls.Signature{sig}).Marshal()
|
|
|
|
indexedAtt1, err := blocks.ConvertToIndexed(context.Background(), bState, att1)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
indexedAtt2, err := blocks.ConvertToIndexed(context.Background(), bState, att2)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
slashing := ðpb.AttesterSlashing{
|
|
Attestation_1: indexedAtt1,
|
|
Attestation_2: indexedAtt2,
|
|
}
|
|
attesterSlashings[i] = slashing
|
|
}
|
|
return attesterSlashings
|
|
}
|
|
|
|
// generateAttestations creates attestations that are entirely valid, for the current state slot.
|
|
// This function always returns all validators participating, if maxAttestations is 1, then it will
|
|
// return 1 attestation with all validators aggregated into it. If maxAttestations is set to 4, then
|
|
// it will return 4 attestations for the same data with their aggregation bits split uniformly.
|
|
func generateAttestations(
|
|
t testing.TB,
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
maxAttestations uint64,
|
|
) []*ethpb.Attestation {
|
|
headState := proto.Clone(bState).(*pb.BeaconState)
|
|
headState, err := state.ProcessSlots(context.Background(), headState, bState.Slot+1)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
currentEpoch := helpers.CurrentEpoch(bState)
|
|
attestations := make([]*ethpb.Attestation, maxAttestations)
|
|
|
|
committee, err := helpers.BeaconCommittee(bState, currentEpoch, 0)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
headRoot, err := helpers.BlockRootAtSlot(headState, bState.Slot)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
targetRoot := make([]byte, 32)
|
|
epochStartSlot := helpers.StartSlot(currentEpoch)
|
|
if epochStartSlot == headState.Slot {
|
|
targetRoot = headRoot[:]
|
|
} else {
|
|
targetRoot, err = helpers.BlockRootAtSlot(headState, epochStartSlot)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
}
|
|
|
|
committeeSize := uint64(len(committee))
|
|
custodyBits := bitfield.NewBitlist(committeeSize)
|
|
attestingSlot := uint64(0)
|
|
if bState.Slot > 0 {
|
|
attestingSlot = bState.Slot - 1
|
|
}
|
|
|
|
att := ðpb.Attestation{
|
|
Data: ðpb.AttestationData{
|
|
Slot: attestingSlot,
|
|
BeaconBlockRoot: headRoot,
|
|
Source: bState.CurrentJustifiedCheckpoint,
|
|
Target: ðpb.Checkpoint{
|
|
Epoch: currentEpoch,
|
|
Root: targetRoot,
|
|
},
|
|
},
|
|
CustodyBits: custodyBits,
|
|
}
|
|
|
|
dataRoot, err := ssz.HashTreeRoot(&pb.AttestationDataAndCustodyBit{
|
|
Data: att.Data,
|
|
CustodyBit: false,
|
|
})
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
if maxAttestations > committeeSize {
|
|
t.Fatalf(
|
|
"requested %d attestations per block but there are only %d committee members",
|
|
maxAttestations,
|
|
len(committee),
|
|
)
|
|
}
|
|
|
|
bitsPerAtt := committeeSize / maxAttestations
|
|
domain := helpers.Domain(bState.Fork, currentEpoch, params.BeaconConfig().DomainBeaconAttester)
|
|
for i := uint64(0); i < committeeSize; i += bitsPerAtt {
|
|
aggregationBits := bitfield.NewBitlist(committeeSize)
|
|
sigs := []*bls.Signature{}
|
|
for b := i; b < i+bitsPerAtt; b++ {
|
|
aggregationBits.SetBitAt(b, true)
|
|
sigs = append(sigs, privs[committee[b]].Sign(dataRoot[:], domain))
|
|
}
|
|
att.AggregationBits = aggregationBits
|
|
|
|
att.Signature = bls.AggregateSignatures(sigs).Marshal()
|
|
attestations[i/bitsPerAtt] = att
|
|
}
|
|
return attestations
|
|
}
|
|
|
|
func generateDepositsAndEth1Data(
|
|
t testing.TB,
|
|
bState *pb.BeaconState,
|
|
maxDeposits uint64,
|
|
) (
|
|
[]*ethpb.Deposit,
|
|
*ethpb.Eth1Data,
|
|
) {
|
|
previousDepsLen := bState.Eth1DepositIndex
|
|
currentDeposits, _, _ := SetupInitialDeposits(t, previousDepsLen+maxDeposits)
|
|
eth1Data := GenerateEth1Data(t, currentDeposits)
|
|
return currentDeposits[previousDepsLen:], eth1Data
|
|
}
|
|
|
|
func generateVoluntaryExits(
|
|
t testing.TB,
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
maxExits uint64,
|
|
) []*ethpb.VoluntaryExit {
|
|
currentEpoch := helpers.CurrentEpoch(bState)
|
|
validatorCount, err := helpers.ActiveValidatorCount(bState, currentEpoch)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
voluntaryExits := make([]*ethpb.VoluntaryExit, maxExits)
|
|
for i := 0; i < len(voluntaryExits); i++ {
|
|
valIndex := float64(validatorCount)*(2.0/3.0) + float64(i)
|
|
exit := ðpb.VoluntaryExit{
|
|
Epoch: helpers.PrevEpoch(bState),
|
|
ValidatorIndex: uint64(valIndex),
|
|
}
|
|
root, err := ssz.SigningRoot(exit)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
domain := helpers.Domain(bState.Fork, currentEpoch, params.BeaconConfig().DomainVoluntaryExit)
|
|
exit.Signature = privs[uint64(valIndex)].Sign(root[:], domain).Marshal()
|
|
voluntaryExits[i] = exit
|
|
}
|
|
return voluntaryExits
|
|
}
|