mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
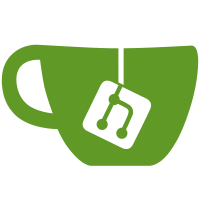
* panic in SizeSSZ * moving slowly * adapt old code to new interfaces * return interfaces from factory functions * replace the rest of WrappedSignedBeaconBlock * WrappedBeaconBlock * WrappedBeaconBlockBody * miscellaneous * Test_BeaconBlockIsNil * replace usages of BeaconBlockIsNil * replace usages of mutator * fix all build errors * fix some more issues * mutator changes * relax assertions when initializing * revert changes in object_mapping.go * allow calling Proto on nil * Revert "allow calling Proto on nil" This reverts commit ecc84e455381b03d24aec2fa0fa17bddbec71705. * modify Copy and Proto methods * remove unused var * fix block batch tests * correct BUILD file * Error when initializing nil objects * one more error fix * add missing comma * rename alias to blocktest * add logging * error when SignedBeaconBlock is nil * fix last test * import fix * broken * working * test fixes * reduce complexity of processPendingBlocks * simplified
214 lines
8.6 KiB
Go
214 lines
8.6 KiB
Go
package validator
|
|
|
|
import (
|
|
"context"
|
|
"encoding/hex"
|
|
"fmt"
|
|
"time"
|
|
|
|
"github.com/ethereum/go-ethereum/common"
|
|
"github.com/ethereum/go-ethereum/common/hexutil"
|
|
emptypb "github.com/golang/protobuf/ptypes/empty"
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/feed"
|
|
blockfeed "github.com/prysmaticlabs/prysm/beacon-chain/core/feed/block"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/transition"
|
|
"github.com/prysmaticlabs/prysm/config/params"
|
|
"github.com/prysmaticlabs/prysm/consensus-types/blocks"
|
|
"github.com/prysmaticlabs/prysm/consensus-types/interfaces"
|
|
types "github.com/prysmaticlabs/prysm/consensus-types/primitives"
|
|
"github.com/prysmaticlabs/prysm/encoding/bytesutil"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/time/slots"
|
|
"github.com/sirupsen/logrus"
|
|
"go.opencensus.io/trace"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
)
|
|
|
|
// eth1DataNotification is a latch to stop flooding logs with the same warning.
|
|
var eth1DataNotification bool
|
|
|
|
const eth1dataTimeout = 2 * time.Second
|
|
|
|
// GetBeaconBlock is called by a proposer during its assigned slot to request a block to sign
|
|
// by passing in the slot and the signed randao reveal of the slot. Returns phase0 beacon blocks
|
|
// before the Altair fork epoch and Altair blocks post-fork epoch.
|
|
func (vs *Server) GetBeaconBlock(ctx context.Context, req *ethpb.BlockRequest) (*ethpb.GenericBeaconBlock, error) {
|
|
ctx, span := trace.StartSpan(ctx, "ProposerServer.GetBeaconBlock")
|
|
defer span.End()
|
|
span.AddAttributes(trace.Int64Attribute("slot", int64(req.Slot)))
|
|
if slots.ToEpoch(req.Slot) < params.BeaconConfig().AltairForkEpoch {
|
|
blk, err := vs.getPhase0BeaconBlock(ctx, req)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not fetch phase0 beacon block: %v", err)
|
|
}
|
|
return ðpb.GenericBeaconBlock{Block: ðpb.GenericBeaconBlock_Phase0{Phase0: blk}}, nil
|
|
} else if slots.ToEpoch(req.Slot) < params.BeaconConfig().BellatrixForkEpoch {
|
|
blk, err := vs.getAltairBeaconBlock(ctx, req)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not fetch Altair beacon block: %v", err)
|
|
}
|
|
return ðpb.GenericBeaconBlock{Block: ðpb.GenericBeaconBlock_Altair{Altair: blk}}, nil
|
|
}
|
|
|
|
// An optimistic validator MUST NOT produce a block (i.e., sign across the DOMAIN_BEACON_PROPOSER domain).
|
|
if err := vs.optimisticStatus(ctx); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return vs.getBellatrixBeaconBlock(ctx, req)
|
|
}
|
|
|
|
// GetBlock is called by a proposer during its assigned slot to request a block to sign
|
|
// by passing in the slot and the signed randao reveal of the slot.
|
|
//
|
|
// DEPRECATED: Use GetBeaconBlock instead to handle blocks pre and post-Altair hard fork. This endpoint
|
|
// cannot handle blocks after the Altair fork epoch. If requesting a block after Altair, nothing will
|
|
// be returned.
|
|
func (vs *Server) GetBlock(ctx context.Context, req *ethpb.BlockRequest) (*ethpb.BeaconBlock, error) {
|
|
ctx, span := trace.StartSpan(ctx, "ProposerServer.GetBlock")
|
|
defer span.End()
|
|
span.AddAttributes(trace.Int64Attribute("slot", int64(req.Slot)))
|
|
blk, err := vs.GetBeaconBlock(ctx, req)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return blk.GetPhase0(), nil
|
|
}
|
|
|
|
// ProposeBeaconBlock is called by a proposer during its assigned slot to create a block in an attempt
|
|
// to get it processed by the beacon node as the canonical head.
|
|
func (vs *Server) ProposeBeaconBlock(ctx context.Context, req *ethpb.GenericSignedBeaconBlock) (*ethpb.ProposeResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "ProposerServer.ProposeBeaconBlock")
|
|
defer span.End()
|
|
blk, err := blocks.NewSignedBeaconBlock(req.Block)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.InvalidArgument, "Could not decode block: %v", err)
|
|
}
|
|
return vs.proposeGenericBeaconBlock(ctx, blk)
|
|
}
|
|
|
|
// ProposeBlock is called by a proposer during its assigned slot to create a block in an attempt
|
|
// to get it processed by the beacon node as the canonical head.
|
|
//
|
|
// DEPRECATED: Use ProposeBeaconBlock instead.
|
|
func (vs *Server) ProposeBlock(ctx context.Context, rBlk *ethpb.SignedBeaconBlock) (*ethpb.ProposeResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "ProposerServer.ProposeBlock")
|
|
defer span.End()
|
|
blk, err := blocks.NewSignedBeaconBlock(rBlk)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.InvalidArgument, "Could not decode block: %v", err)
|
|
}
|
|
return vs.proposeGenericBeaconBlock(ctx, blk)
|
|
}
|
|
|
|
// PrepareBeaconProposer caches and updates the fee recipient for the given proposer.
|
|
func (vs *Server) PrepareBeaconProposer(
|
|
ctx context.Context, request *ethpb.PrepareBeaconProposerRequest,
|
|
) (*emptypb.Empty, error) {
|
|
ctx, span := trace.StartSpan(ctx, "validator.PrepareBeaconProposer")
|
|
defer span.End()
|
|
var feeRecipients []common.Address
|
|
var validatorIndices []types.ValidatorIndex
|
|
for _, recipientContainer := range request.Recipients {
|
|
recipient := hexutil.Encode(recipientContainer.FeeRecipient)
|
|
if !common.IsHexAddress(recipient) {
|
|
return nil, status.Errorf(codes.InvalidArgument, fmt.Sprintf("Invalid fee recipient address: %v", recipient))
|
|
}
|
|
feeRecipients = append(feeRecipients, common.BytesToAddress(recipientContainer.FeeRecipient))
|
|
validatorIndices = append(validatorIndices, recipientContainer.ValidatorIndex)
|
|
}
|
|
if err := vs.BeaconDB.SaveFeeRecipientsByValidatorIDs(ctx, validatorIndices, feeRecipients); err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not save fee recipients: %v", err)
|
|
}
|
|
log.WithFields(logrus.Fields{
|
|
"validatorIndices": validatorIndices,
|
|
}).Info("Updated fee recipient addresses for validator indices")
|
|
return &emptypb.Empty{}, nil
|
|
}
|
|
|
|
func (vs *Server) proposeGenericBeaconBlock(ctx context.Context, blk interfaces.SignedBeaconBlock) (*ethpb.ProposeResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "ProposerServer.proposeGenericBeaconBlock")
|
|
defer span.End()
|
|
root, err := blk.Block().HashTreeRoot()
|
|
if err != nil {
|
|
return nil, fmt.Errorf("could not tree hash block: %v", err)
|
|
}
|
|
|
|
blk, err = vs.unblindBuilderBlock(ctx, blk)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
// Do not block proposal critical path with debug logging or block feed updates.
|
|
defer func() {
|
|
log.WithField("blockRoot", fmt.Sprintf("%#x", bytesutil.Trunc(root[:]))).Debugf(
|
|
"Block proposal received via RPC")
|
|
vs.BlockNotifier.BlockFeed().Send(&feed.Event{
|
|
Type: blockfeed.ReceivedBlock,
|
|
Data: &blockfeed.ReceivedBlockData{SignedBlock: blk},
|
|
})
|
|
}()
|
|
|
|
// Broadcast the new block to the network.
|
|
blkPb, err := blk.Proto()
|
|
if err != nil {
|
|
return nil, errors.Wrap(err, "could not get protobuf block")
|
|
}
|
|
if err := vs.P2P.Broadcast(ctx, blkPb); err != nil {
|
|
return nil, fmt.Errorf("could not broadcast block: %v", err)
|
|
}
|
|
log.WithFields(logrus.Fields{
|
|
"blockRoot": hex.EncodeToString(root[:]),
|
|
}).Debug("Broadcasting block")
|
|
|
|
if err := vs.BlockReceiver.ReceiveBlock(ctx, blk, root); err != nil {
|
|
return nil, fmt.Errorf("could not process beacon block: %v", err)
|
|
}
|
|
|
|
return ðpb.ProposeResponse{
|
|
BlockRoot: root[:],
|
|
}, nil
|
|
}
|
|
|
|
// computeStateRoot computes the state root after a block has been processed through a state transition and
|
|
// returns it to the validator client.
|
|
func (vs *Server) computeStateRoot(ctx context.Context, block interfaces.SignedBeaconBlock) ([]byte, error) {
|
|
beaconState, err := vs.StateGen.StateByRoot(ctx, bytesutil.ToBytes32(block.Block().ParentRoot()))
|
|
if err != nil {
|
|
return nil, errors.Wrap(err, "could not retrieve beacon state")
|
|
}
|
|
root, err := transition.CalculateStateRoot(
|
|
ctx,
|
|
beaconState,
|
|
block,
|
|
)
|
|
if err != nil {
|
|
return nil, errors.Wrapf(err, "could not calculate state root at slot %d", beaconState.Slot())
|
|
}
|
|
|
|
log.WithField("beaconStateRoot", fmt.Sprintf("%#x", root)).Debugf("Computed state root")
|
|
return root[:], nil
|
|
}
|
|
|
|
// SubmitValidatorRegistration submits validator registration.
|
|
// Deprecated: Use SubmitValidatorRegistrations instead.
|
|
func (vs *Server) SubmitValidatorRegistration(ctx context.Context, reg *ethpb.SignedValidatorRegistrationV1) (*emptypb.Empty, error) {
|
|
return vs.SubmitValidatorRegistrations(ctx, ðpb.SignedValidatorRegistrationsV1{Messages: []*ethpb.SignedValidatorRegistrationV1{reg}})
|
|
}
|
|
|
|
// SubmitValidatorRegistrations submits validator registrations.
|
|
func (vs *Server) SubmitValidatorRegistrations(ctx context.Context, reg *ethpb.SignedValidatorRegistrationsV1) (*emptypb.Empty, error) {
|
|
// No-op is the builder is nil / not configured. The node should still function without a builder.
|
|
if vs.BlockBuilder == nil || !vs.BlockBuilder.Configured() {
|
|
return &emptypb.Empty{}, nil
|
|
}
|
|
|
|
if err := vs.BlockBuilder.RegisterValidator(ctx, reg.Messages); err != nil {
|
|
return nil, status.Errorf(codes.InvalidArgument, "Could not register block builder: %v", err)
|
|
}
|
|
|
|
return &emptypb.Empty{}, nil
|
|
}
|