mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
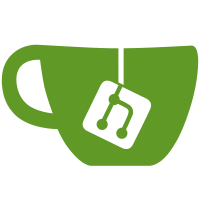
* panic in SizeSSZ * moving slowly * adapt old code to new interfaces * return interfaces from factory functions * replace the rest of WrappedSignedBeaconBlock * WrappedBeaconBlock * WrappedBeaconBlockBody * miscellaneous * Test_BeaconBlockIsNil * replace usages of BeaconBlockIsNil * replace usages of mutator * fix all build errors * fix some more issues * mutator changes * relax assertions when initializing * revert changes in object_mapping.go * allow calling Proto on nil * Revert "allow calling Proto on nil" This reverts commit ecc84e455381b03d24aec2fa0fa17bddbec71705. * modify Copy and Proto methods * remove unused var * fix block batch tests * correct BUILD file * Error when initializing nil objects * one more error fix * add missing comma * rename alias to blocktest * add logging * error when SignedBeaconBlock is nil * fix last test * import fix * broken * working * test fixes * reduce complexity of processPendingBlocks * simplified
140 lines
4.8 KiB
Go
140 lines
4.8 KiB
Go
package validator
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/transition/interop"
|
|
"github.com/prysmaticlabs/prysm/config/params"
|
|
"github.com/prysmaticlabs/prysm/consensus-types/blocks"
|
|
types "github.com/prysmaticlabs/prysm/consensus-types/primitives"
|
|
"github.com/prysmaticlabs/prysm/crypto/bls"
|
|
"github.com/prysmaticlabs/prysm/encoding/bytesutil"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
synccontribution "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1/attestation/aggregation/sync_contribution"
|
|
"go.opencensus.io/trace"
|
|
)
|
|
|
|
func (vs *Server) buildAltairBeaconBlock(ctx context.Context, req *ethpb.BlockRequest) (*ethpb.BeaconBlockAltair, error) {
|
|
ctx, span := trace.StartSpan(ctx, "ProposerServer.buildAltairBeaconBlock")
|
|
defer span.End()
|
|
blkData, err := vs.buildPhase0BlockData(ctx, req)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("could not build block data: %v", err)
|
|
}
|
|
|
|
// Use zero hash as stub for state root to compute later.
|
|
stateRoot := params.BeaconConfig().ZeroHash[:]
|
|
|
|
// No need for safe sub as req.Slot cannot be 0 if requesting Altair blocks. If 0, we will be throwing
|
|
// an error in the first validity check of this endpoint.
|
|
syncAggregate, err := vs.getSyncAggregate(ctx, req.Slot-1, bytesutil.ToBytes32(blkData.ParentRoot))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return ðpb.BeaconBlockAltair{
|
|
Slot: req.Slot,
|
|
ParentRoot: blkData.ParentRoot,
|
|
StateRoot: stateRoot,
|
|
ProposerIndex: blkData.ProposerIdx,
|
|
Body: ðpb.BeaconBlockBodyAltair{
|
|
Eth1Data: blkData.Eth1Data,
|
|
Deposits: blkData.Deposits,
|
|
Attestations: blkData.Attestations,
|
|
RandaoReveal: req.RandaoReveal,
|
|
ProposerSlashings: blkData.ProposerSlashings,
|
|
AttesterSlashings: blkData.AttesterSlashings,
|
|
VoluntaryExits: blkData.VoluntaryExits,
|
|
Graffiti: blkData.Graffiti[:],
|
|
SyncAggregate: syncAggregate,
|
|
},
|
|
}, nil
|
|
}
|
|
|
|
func (vs *Server) getAltairBeaconBlock(ctx context.Context, req *ethpb.BlockRequest) (*ethpb.BeaconBlockAltair, error) {
|
|
ctx, span := trace.StartSpan(ctx, "ProposerServer.getAltairBeaconBlock")
|
|
defer span.End()
|
|
blk, err := vs.buildAltairBeaconBlock(ctx, req)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("could not build block data: %v", err)
|
|
}
|
|
// Compute state root with the newly constructed block.
|
|
wsb, err := blocks.NewSignedBeaconBlock(
|
|
ðpb.SignedBeaconBlockAltair{Block: blk, Signature: make([]byte, 96)},
|
|
)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
stateRoot, err := vs.computeStateRoot(ctx, wsb)
|
|
if err != nil {
|
|
interop.WriteBlockToDisk(wsb, true /*failed*/)
|
|
return nil, fmt.Errorf("could not compute state root: %v", err)
|
|
}
|
|
blk.StateRoot = stateRoot
|
|
return blk, nil
|
|
}
|
|
|
|
// getSyncAggregate retrieves the sync contributions from the pool to construct the sync aggregate object.
|
|
// The contributions are filtered based on matching of the input root and slot then profitability.
|
|
func (vs *Server) getSyncAggregate(ctx context.Context, slot types.Slot, root [32]byte) (*ethpb.SyncAggregate, error) {
|
|
ctx, span := trace.StartSpan(ctx, "ProposerServer.getSyncAggregate")
|
|
defer span.End()
|
|
|
|
// Contributions have to match the input root
|
|
contributions, err := vs.SyncCommitteePool.SyncCommitteeContributions(slot)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
proposerContributions := proposerSyncContributions(contributions).filterByBlockRoot(root)
|
|
|
|
// Each sync subcommittee is 128 bits and the sync committee is 512 bits for mainnet.
|
|
var bitsHolder [][]byte
|
|
for i := uint64(0); i < params.BeaconConfig().SyncCommitteeSubnetCount; i++ {
|
|
bitsHolder = append(bitsHolder, ethpb.NewSyncCommitteeAggregationBits())
|
|
}
|
|
sigsHolder := make([]bls.Signature, 0, params.BeaconConfig().SyncCommitteeSize/params.BeaconConfig().SyncCommitteeSubnetCount)
|
|
|
|
for i := uint64(0); i < params.BeaconConfig().SyncCommitteeSubnetCount; i++ {
|
|
cs := proposerContributions.filterBySubIndex(i)
|
|
aggregates, err := synccontribution.Aggregate(cs)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
// Retrieve the most profitable contribution
|
|
deduped, err := proposerSyncContributions(aggregates).dedup()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
c := deduped.mostProfitable()
|
|
if c == nil {
|
|
continue
|
|
}
|
|
bitsHolder[i] = c.AggregationBits
|
|
sig, err := bls.SignatureFromBytes(c.Signature)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
sigsHolder = append(sigsHolder, sig)
|
|
}
|
|
|
|
// Aggregate all the contribution bits and signatures.
|
|
var syncBits []byte
|
|
for _, b := range bitsHolder {
|
|
syncBits = append(syncBits, b...)
|
|
}
|
|
syncSig := bls.AggregateSignatures(sigsHolder)
|
|
var syncSigBytes [96]byte
|
|
if syncSig == nil {
|
|
syncSigBytes = [96]byte{0xC0} // Infinity signature if itself is nil.
|
|
} else {
|
|
syncSigBytes = bytesutil.ToBytes96(syncSig.Marshal())
|
|
}
|
|
|
|
return ðpb.SyncAggregate{
|
|
SyncCommitteeBits: syncBits,
|
|
SyncCommitteeSignature: syncSigBytes[:],
|
|
}, nil
|
|
}
|