mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
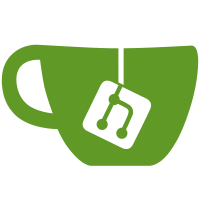
* Add http endpoint for GetValidatorPerformance * Add tests * fix up client usage * Revert changes * refactor to reuse code * Move endpoint + move ComputeValidatorPerformance * Radek's comment change * Add Bazel file * Change endpoint path * Add server for http endpoints * Fix server * Create core package * Gaz * Add correct error code * Fix error code in test * Adding errors * Fix errors * Fix default GRPC error * Change http errors to core ones * Use error status without helper * Fix * Capitalize GRPC error messages --------- Co-authored-by: Raul Jordan <raul@prysmaticlabs.com>
50 lines
876 B
Go
50 lines
876 B
Go
package core
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"google.golang.org/grpc/codes"
|
|
)
|
|
|
|
type ErrorReason uint8
|
|
|
|
const (
|
|
Internal = iota
|
|
Unavailable
|
|
BadRequest
|
|
// Add more errors as needed
|
|
)
|
|
|
|
type RpcError struct {
|
|
Err error
|
|
Reason ErrorReason
|
|
}
|
|
|
|
func ErrorReasonToGRPC(reason ErrorReason) codes.Code {
|
|
switch reason {
|
|
case Internal:
|
|
return codes.Internal
|
|
case Unavailable:
|
|
return codes.Unavailable
|
|
case BadRequest:
|
|
return codes.InvalidArgument
|
|
// Add more cases for other error reasons as needed
|
|
default:
|
|
return codes.Internal
|
|
}
|
|
}
|
|
|
|
func ErrorReasonToHTTP(reason ErrorReason) int {
|
|
switch reason {
|
|
case Internal:
|
|
return http.StatusInternalServerError
|
|
case Unavailable:
|
|
return http.StatusServiceUnavailable
|
|
case BadRequest:
|
|
return http.StatusBadRequest
|
|
// Add more cases for other error reasons as needed
|
|
default:
|
|
return http.StatusInternalServerError
|
|
}
|
|
}
|