mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
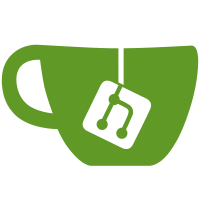
* Update seed domains (#3872) * Remove Transfers (#3870) * Remove active index roots and compact committee roots (#3869) * Update inclusion reward (#3886) * Alter proposer selection logic (#3884) * Fix early committee bias (#3888) * Remove shards and committees (#3896) * Epoch spec tests v0.9 (#3907) * Block spec test v0.9 (#3905) * rm'ed in protobuf * build proto * build proto * build proto * fix core package * Gazelle * Fixed all the tests * Fixed static test * Comment out spec test for now * One more skip * fix-roundRobinSync (#3862) * Starting but need new seed function * Revert initial sync * Updated Proposer Slashing * Fixed all tests * Lint * Update inclusion reward * Fill randao mixes with eth1 data hash * Test * Fixing test part1 * All tests passing * One last test * Updated config * Build proto * Proper skip message * Conflict and fmt * Removed crosslinks and shards. Built * Format and gazelle * Fixed all the block package tests * Fixed all the helper tests * All epoch package tests pass * All core package tests pass * Fixed operation tests * Started fixing rpc test * RPC tests passed! * Fixed all init sync tests * All tests pass * Fixed blockchain tests * Lint * Lint * Preston's feedback * Starting * Remove container * Fixed block spec tests * All passing except for block_processing test * Failing block processing test * Starting * Add AggregateAndProof * All mainnet test passes * Update deposit contract (#3906) * Proto spec tests v0.9 (#3908) * Starting * Add AggregateAndProof * Unskip block util tests (#3910) * rm'ed in protobuf * build proto * build proto * build proto * fix core package * Gazelle * Fixed all the tests * Fixed static test * Comment out spec test for now * One more skip * fix-roundRobinSync (#3862) * Starting but need new seed function * Revert initial sync * Updated Proposer Slashing * Fixed all tests * Lint * Update inclusion reward * Fill randao mixes with eth1 data hash * Test * Fixing test part1 * All tests passing * One last test * Updated config * Build proto * Proper skip message * Conflict and fmt * Removed crosslinks and shards. Built * Format and gazelle * Fixed all the block package tests * Fixed all the helper tests * All epoch package tests pass * All core package tests pass * Fixed operation tests * Started fixing rpc test * RPC tests passed! * Fixed all init sync tests * All tests pass * Fixed blockchain tests * Lint * Lint * Preston's feedback * Starting * Remove container * Fixed block spec tests * All passing except for block_processing test * Failing block processing test * Starting * Add AggregateAndProof * All mainnet test passes * Unskip block util tests * Slot processing spec test V0.9 (#3912) * Starting * Add AggregateAndProof * Unskip slot processing mainnet test * Unskip minimal spec test for finalization (#3920) * Remove outdated interop tests (#3922) * Rm outdated interop tests * Rm test runner * Gazelle * Update validator to use proposer slot (#3919) * Fix committee assignment (#3931) * Replace shard with committee index (#3930) * Conflict * Clean up (#3933) * Remove shard filter in db (#3936) * Remove lightouse compatibility test (#3939) * Update Committee Cache for v0.9 (#3948) * Updated committee cache * Removed shuffled indices cache * Started testing run time * Lint * Fixed test * Safeguard against nil head state * address edge case * add test * Fixed TestRoundRobinSync by doubling the epochs * Unskip TestProtoCompatability (#3958) * Unskip TestProtoCompatability * Update WORKSPACE * Fix minimal config (#3959) * fix minimal configs * fix hardcoded value in test * Simplify verify att time (#3961) * update readme for deposit contract, regen bindings for vyper 0.1.0b12 (#3963) * update readme for deposit contract, regen bindings * medium * Check nil base state (#3964) * Copy Block When Receiving it From Sync (#3966) * copy block * clone for other service methods too * Change logging of Bitfield (#3956) * change logging of bits * preston's review * Unskip Beacon Server Test (#3962) * run test till the end * fix up proto message types * fmt * resolve broken tests * better error handling * fixing new logic to use archived proposer info * fix up logic * clip using the max effective balance * broken build fix with num arg mismatch * amend archive * archival logic changed * rename test * archive both proposer and attester seeds * page size 100 * further experiments * further experimentation, archivedProposerIndex seems wrong * test passes * rem log * fix broken test * fix test * gaz * fix imports * ethapis
456 lines
14 KiB
Go
456 lines
14 KiB
Go
package powchain
|
|
|
|
import (
|
|
"bytes"
|
|
"context"
|
|
"encoding/binary"
|
|
"io/ioutil"
|
|
"math/big"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/ethereum/go-ethereum"
|
|
"github.com/ethereum/go-ethereum/common"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/cache/depositcache"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/db/kv"
|
|
contracts "github.com/prysmaticlabs/prysm/contracts/deposit-contract"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
"github.com/sirupsen/logrus"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
func init() {
|
|
logrus.SetLevel(logrus.DebugLevel)
|
|
logrus.SetOutput(ioutil.Discard)
|
|
}
|
|
|
|
func TestProcessDepositLog_OK(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
testutil.ResetCache()
|
|
testAcc, err := contracts.Setup()
|
|
if err != nil {
|
|
t.Fatalf("Unable to set up simulated backend %v", err)
|
|
}
|
|
web3Service, err := NewService(context.Background(), &Web3ServiceConfig{
|
|
ETH1Endpoint: endpoint,
|
|
DepositContract: testAcc.ContractAddr,
|
|
BeaconDB: &kv.Store{},
|
|
DepositCache: depositcache.NewDepositCache(),
|
|
})
|
|
if err != nil {
|
|
t.Fatalf("unable to setup web3 ETH1.0 chain service: %v", err)
|
|
}
|
|
web3Service = setDefaultMocks(web3Service)
|
|
web3Service.depositContractCaller, err = contracts.NewDepositContractCaller(testAcc.ContractAddr, testAcc.Backend)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
testAcc.Backend.Commit()
|
|
deposits, depositRoots, _ := testutil.SetupInitialDeposits(t, 1)
|
|
data := deposits[0].Data
|
|
|
|
testAcc.TxOpts.Value = contracts.Amount32Eth()
|
|
testAcc.TxOpts.GasLimit = 1000000
|
|
if _, err := testAcc.Contract.Deposit(testAcc.TxOpts, data.PublicKey, data.WithdrawalCredentials, data.Signature, depositRoots[0]); err != nil {
|
|
t.Fatalf("Could not deposit to deposit contract %v", err)
|
|
}
|
|
|
|
testAcc.Backend.Commit()
|
|
|
|
query := ethereum.FilterQuery{
|
|
Addresses: []common.Address{
|
|
web3Service.depositContractAddress,
|
|
},
|
|
}
|
|
|
|
logs, err := testAcc.Backend.FilterLogs(web3Service.ctx, query)
|
|
if err != nil {
|
|
t.Fatalf("Unable to retrieve logs %v", err)
|
|
}
|
|
if len(logs) == 0 {
|
|
t.Fatal("no logs")
|
|
}
|
|
|
|
web3Service.ProcessLog(context.Background(), logs[0])
|
|
|
|
testutil.AssertLogsDoNotContain(t, hook, "Could not unpack log")
|
|
testutil.AssertLogsDoNotContain(t, hook, "Could not save in trie")
|
|
testutil.AssertLogsDoNotContain(t, hook, "could not deserialize validator public key")
|
|
testutil.AssertLogsDoNotContain(t, hook, "could not convert bytes to signature")
|
|
testutil.AssertLogsDoNotContain(t, hook, "could not sign root for deposit data")
|
|
testutil.AssertLogsDoNotContain(t, hook, "deposit signature did not verify")
|
|
testutil.AssertLogsDoNotContain(t, hook, "could not tree hash deposit data")
|
|
testutil.AssertLogsDoNotContain(t, hook, "deposit merkle branch of deposit root did not verify for root")
|
|
testutil.AssertLogsContain(t, hook, "Deposit registered from deposit contract")
|
|
|
|
hook.Reset()
|
|
}
|
|
|
|
func TestProcessDepositLog_InsertsPendingDeposit(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
testAcc, err := contracts.Setup()
|
|
if err != nil {
|
|
t.Fatalf("Unable to set up simulated backend %v", err)
|
|
}
|
|
web3Service, err := NewService(context.Background(), &Web3ServiceConfig{
|
|
ETH1Endpoint: endpoint,
|
|
DepositContract: testAcc.ContractAddr,
|
|
BeaconDB: &kv.Store{},
|
|
DepositCache: depositcache.NewDepositCache(),
|
|
})
|
|
if err != nil {
|
|
t.Fatalf("unable to setup web3 ETH1.0 chain service: %v", err)
|
|
}
|
|
web3Service = setDefaultMocks(web3Service)
|
|
web3Service.depositContractCaller, err = contracts.NewDepositContractCaller(testAcc.ContractAddr, testAcc.Backend)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
testAcc.Backend.Commit()
|
|
|
|
deposits, depositRoots, _ := testutil.SetupInitialDeposits(t, 1)
|
|
data := deposits[0].Data
|
|
|
|
testAcc.TxOpts.Value = contracts.Amount32Eth()
|
|
testAcc.TxOpts.GasLimit = 1000000
|
|
|
|
if _, err := testAcc.Contract.Deposit(testAcc.TxOpts, data.PublicKey, data.WithdrawalCredentials, data.Signature, depositRoots[0]); err != nil {
|
|
t.Fatalf("Could not deposit to deposit contract %v", err)
|
|
}
|
|
|
|
if _, err := testAcc.Contract.Deposit(testAcc.TxOpts, data.PublicKey, data.WithdrawalCredentials, data.Signature, depositRoots[0]); err != nil {
|
|
t.Fatalf("Could not deposit to deposit contract %v", err)
|
|
}
|
|
|
|
testAcc.Backend.Commit()
|
|
|
|
query := ethereum.FilterQuery{
|
|
Addresses: []common.Address{
|
|
web3Service.depositContractAddress,
|
|
},
|
|
}
|
|
|
|
logs, err := testAcc.Backend.FilterLogs(web3Service.ctx, query)
|
|
if err != nil {
|
|
t.Fatalf("Unable to retrieve logs %v", err)
|
|
}
|
|
|
|
web3Service.chainStarted = true
|
|
|
|
web3Service.ProcessDepositLog(context.Background(), logs[0])
|
|
web3Service.ProcessDepositLog(context.Background(), logs[1])
|
|
pendingDeposits := web3Service.depositCache.PendingDeposits(context.Background(), nil /*blockNum*/)
|
|
if len(pendingDeposits) != 2 {
|
|
t.Errorf("Unexpected number of deposits. Wanted 2 deposit, got %+v", pendingDeposits)
|
|
}
|
|
hook.Reset()
|
|
}
|
|
|
|
func TestUnpackDepositLogData_OK(t *testing.T) {
|
|
testAcc, err := contracts.Setup()
|
|
if err != nil {
|
|
t.Fatalf("Unable to set up simulated backend %v", err)
|
|
}
|
|
web3Service, err := NewService(context.Background(), &Web3ServiceConfig{
|
|
ETH1Endpoint: endpoint,
|
|
DepositContract: testAcc.ContractAddr,
|
|
})
|
|
if err != nil {
|
|
t.Fatalf("unable to setup web3 ETH1.0 chain service: %v", err)
|
|
}
|
|
web3Service = setDefaultMocks(web3Service)
|
|
web3Service.depositContractCaller, err = contracts.NewDepositContractCaller(testAcc.ContractAddr, testAcc.Backend)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
testAcc.Backend.Commit()
|
|
|
|
if err := web3Service.initDataFromContract(); err != nil {
|
|
t.Fatalf("Could not init from contract: %v", err)
|
|
}
|
|
|
|
deposits, depositRoots, _ := testutil.SetupInitialDeposits(t, 1)
|
|
data := deposits[0].Data
|
|
|
|
testAcc.TxOpts.Value = contracts.Amount32Eth()
|
|
testAcc.TxOpts.GasLimit = 1000000
|
|
if _, err := testAcc.Contract.Deposit(testAcc.TxOpts, data.PublicKey, data.WithdrawalCredentials, data.Signature, depositRoots[0]); err != nil {
|
|
t.Fatalf("Could not deposit to deposit contract %v", err)
|
|
}
|
|
testAcc.Backend.Commit()
|
|
|
|
query := ethereum.FilterQuery{
|
|
Addresses: []common.Address{
|
|
web3Service.depositContractAddress,
|
|
},
|
|
}
|
|
|
|
logz, err := testAcc.Backend.FilterLogs(web3Service.ctx, query)
|
|
if err != nil {
|
|
t.Fatalf("Unable to retrieve logs %v", err)
|
|
}
|
|
|
|
loggedPubkey, withCreds, _, loggedSig, index, err := contracts.UnpackDepositLogData(logz[0].Data)
|
|
if err != nil {
|
|
t.Fatalf("Unable to unpack logs %v", err)
|
|
}
|
|
|
|
if binary.LittleEndian.Uint64(index) != 0 {
|
|
t.Errorf("Retrieved merkle tree index is incorrect %d", index)
|
|
}
|
|
|
|
if !bytes.Equal(loggedPubkey, data.PublicKey) {
|
|
t.Errorf("Pubkey is not the same as the data that was put in %v", loggedPubkey)
|
|
}
|
|
|
|
if !bytes.Equal(loggedSig, data.Signature) {
|
|
t.Errorf("Proof of Possession is not the same as the data that was put in %v", loggedSig)
|
|
}
|
|
|
|
if !bytes.Equal(withCreds, data.WithdrawalCredentials) {
|
|
t.Errorf("Withdrawal Credentials is not the same as the data that was put in %v", withCreds)
|
|
}
|
|
|
|
}
|
|
|
|
func TestProcessETH2GenesisLog_8DuplicatePubkeys(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
testAcc, err := contracts.Setup()
|
|
if err != nil {
|
|
t.Fatalf("Unable to set up simulated backend %v", err)
|
|
}
|
|
web3Service, err := NewService(context.Background(), &Web3ServiceConfig{
|
|
ETH1Endpoint: endpoint,
|
|
DepositContract: testAcc.ContractAddr,
|
|
BeaconDB: &kv.Store{},
|
|
DepositCache: depositcache.NewDepositCache(),
|
|
})
|
|
if err != nil {
|
|
t.Fatalf("unable to setup web3 ETH1.0 chain service: %v", err)
|
|
}
|
|
web3Service = setDefaultMocks(web3Service)
|
|
web3Service.depositContractCaller, err = contracts.NewDepositContractCaller(testAcc.ContractAddr, testAcc.Backend)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
bConfig := params.MinimalSpecConfig()
|
|
bConfig.MinGenesisTime = 0
|
|
params.OverrideBeaconConfig(bConfig)
|
|
|
|
testAcc.Backend.Commit()
|
|
testAcc.Backend.AdjustTime(time.Duration(int64(time.Now().Nanosecond())))
|
|
|
|
deposits, depositRoots, _ := testutil.SetupInitialDeposits(t, 1)
|
|
data := deposits[0].Data
|
|
|
|
testAcc.TxOpts.Value = contracts.Amount32Eth()
|
|
testAcc.TxOpts.GasLimit = 1000000
|
|
|
|
// 64 Validators are used as size required for beacon-chain to start. This number
|
|
// is defined in the deposit contract as the number required for the testnet. The actual number
|
|
// is 2**14
|
|
for i := 0; i < depositsReqForChainStart; i++ {
|
|
testAcc.TxOpts.Value = contracts.Amount32Eth()
|
|
if _, err := testAcc.Contract.Deposit(testAcc.TxOpts, data.PublicKey, data.WithdrawalCredentials, data.Signature, depositRoots[0]); err != nil {
|
|
t.Fatalf("Could not deposit to deposit contract %v", err)
|
|
}
|
|
|
|
testAcc.Backend.Commit()
|
|
}
|
|
|
|
query := ethereum.FilterQuery{
|
|
Addresses: []common.Address{
|
|
web3Service.depositContractAddress,
|
|
},
|
|
}
|
|
|
|
logs, err := testAcc.Backend.FilterLogs(web3Service.ctx, query)
|
|
if err != nil {
|
|
t.Fatalf("Unable to retrieve logs %v", err)
|
|
}
|
|
|
|
for _, log := range logs {
|
|
web3Service.ProcessLog(context.Background(), log)
|
|
}
|
|
|
|
if web3Service.chainStarted {
|
|
t.Error("Genesis has been triggered despite being 8 duplicate keys")
|
|
}
|
|
|
|
testutil.AssertLogsDoNotContain(t, hook, "Minimum number of validators reached for beacon-chain to start")
|
|
hook.Reset()
|
|
}
|
|
|
|
func TestProcessETH2GenesisLog(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
testAcc, err := contracts.Setup()
|
|
if err != nil {
|
|
t.Fatalf("Unable to set up simulated backend %v", err)
|
|
}
|
|
web3Service, err := NewService(context.Background(), &Web3ServiceConfig{
|
|
ETH1Endpoint: endpoint,
|
|
DepositContract: testAcc.ContractAddr,
|
|
BeaconDB: &kv.Store{},
|
|
DepositCache: depositcache.NewDepositCache(),
|
|
})
|
|
if err != nil {
|
|
t.Fatalf("unable to setup web3 ETH1.0 chain service: %v", err)
|
|
}
|
|
web3Service = setDefaultMocks(web3Service)
|
|
web3Service.depositContractCaller, err = contracts.NewDepositContractCaller(testAcc.ContractAddr, testAcc.Backend)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
bConfig := params.MinimalSpecConfig()
|
|
bConfig.MinGenesisTime = 0
|
|
params.OverrideBeaconConfig(bConfig)
|
|
|
|
testAcc.Backend.Commit()
|
|
testAcc.Backend.AdjustTime(time.Duration(int64(time.Now().Nanosecond())))
|
|
|
|
deposits, depositRoots, _ := testutil.SetupInitialDeposits(t, uint64(depositsReqForChainStart))
|
|
|
|
// 64 Validators are used as size required for beacon-chain to start. This number
|
|
// is defined in the deposit contract as the number required for the testnet. The actual number
|
|
// is 2**14
|
|
for i := 0; i < depositsReqForChainStart; i++ {
|
|
data := deposits[i].Data
|
|
testAcc.TxOpts.Value = contracts.Amount32Eth()
|
|
testAcc.TxOpts.GasLimit = 1000000
|
|
if _, err := testAcc.Contract.Deposit(testAcc.TxOpts, data.PublicKey, data.WithdrawalCredentials, data.Signature, depositRoots[i]); err != nil {
|
|
t.Fatalf("Could not deposit to deposit contract %v", err)
|
|
}
|
|
|
|
testAcc.Backend.Commit()
|
|
}
|
|
|
|
query := ethereum.FilterQuery{
|
|
Addresses: []common.Address{
|
|
web3Service.depositContractAddress,
|
|
},
|
|
}
|
|
|
|
logs, err := testAcc.Backend.FilterLogs(web3Service.ctx, query)
|
|
if err != nil {
|
|
t.Fatalf("Unable to retrieve logs %v", err)
|
|
}
|
|
|
|
if len(logs) != depositsReqForChainStart {
|
|
t.Fatalf(
|
|
"Did not receive enough logs, received %d, wanted %d",
|
|
len(logs),
|
|
depositsReqForChainStart,
|
|
)
|
|
}
|
|
genesisTimeChan := make(chan time.Time, 1)
|
|
sub := web3Service.chainStartFeed.Subscribe(genesisTimeChan)
|
|
defer sub.Unsubscribe()
|
|
|
|
for _, log := range logs {
|
|
web3Service.ProcessLog(context.Background(), log)
|
|
}
|
|
|
|
err = web3Service.ProcessETH1Block(context.Background(), big.NewInt(int64(logs[len(logs)-1].BlockNumber)))
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
cachedDeposits := web3Service.ChainStartDeposits()
|
|
if len(cachedDeposits) != depositsReqForChainStart {
|
|
t.Fatalf(
|
|
"Did not cache the chain start deposits correctly, received %d, wanted %d",
|
|
len(cachedDeposits),
|
|
depositsReqForChainStart,
|
|
)
|
|
}
|
|
|
|
<-genesisTimeChan
|
|
testutil.AssertLogsDoNotContain(t, hook, "Unable to unpack ChainStart log data")
|
|
testutil.AssertLogsDoNotContain(t, hook, "Receipt root from log doesn't match the root saved in memory")
|
|
testutil.AssertLogsDoNotContain(t, hook, "Invalid timestamp from log")
|
|
testutil.AssertLogsContain(t, hook, "Minimum number of validators reached for beacon-chain to start")
|
|
|
|
hook.Reset()
|
|
}
|
|
|
|
func TestWeb3ServiceProcessDepositLog_RequestMissedDeposits(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
testAcc, err := contracts.Setup()
|
|
if err != nil {
|
|
t.Fatalf("Unable to set up simulated backend %v", err)
|
|
}
|
|
web3Service, err := NewService(context.Background(), &Web3ServiceConfig{
|
|
ETH1Endpoint: endpoint,
|
|
DepositContract: testAcc.ContractAddr,
|
|
BeaconDB: &kv.Store{},
|
|
DepositCache: depositcache.NewDepositCache(),
|
|
})
|
|
if err != nil {
|
|
t.Fatalf("unable to setup web3 ETH1.0 chain service: %v", err)
|
|
}
|
|
web3Service = setDefaultMocks(web3Service)
|
|
web3Service.depositContractCaller, err = contracts.NewDepositContractCaller(testAcc.ContractAddr, testAcc.Backend)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
web3Service.httpLogger = testAcc.Backend
|
|
bConfig := params.MinimalSpecConfig()
|
|
bConfig.MinGenesisTime = 0
|
|
params.OverrideBeaconConfig(bConfig)
|
|
|
|
testAcc.Backend.Commit()
|
|
testAcc.Backend.AdjustTime(time.Duration(int64(time.Now().Nanosecond())))
|
|
depositsWanted := 10
|
|
deposits, depositRoots, _ := testutil.SetupInitialDeposits(t, uint64(depositsWanted))
|
|
|
|
for i := 0; i < depositsWanted; i++ {
|
|
data := deposits[i].Data
|
|
testAcc.TxOpts.Value = contracts.Amount32Eth()
|
|
testAcc.TxOpts.GasLimit = 1000000
|
|
if _, err := testAcc.Contract.Deposit(testAcc.TxOpts, data.PublicKey, data.WithdrawalCredentials, data.Signature, depositRoots[i]); err != nil {
|
|
t.Fatalf("Could not deposit to deposit contract %v", err)
|
|
}
|
|
|
|
testAcc.Backend.Commit()
|
|
}
|
|
|
|
query := ethereum.FilterQuery{
|
|
Addresses: []common.Address{
|
|
web3Service.depositContractAddress,
|
|
},
|
|
}
|
|
|
|
logs, err := testAcc.Backend.FilterLogs(web3Service.ctx, query)
|
|
if err != nil {
|
|
t.Fatalf("Unable to retrieve logs %v", err)
|
|
}
|
|
|
|
if len(logs) != depositsWanted {
|
|
t.Fatalf(
|
|
"Did not receive enough logs, received %d, wanted %d",
|
|
len(logs),
|
|
depositsReqForChainStart,
|
|
)
|
|
}
|
|
|
|
logsToBeProcessed := append(logs[:depositsWanted-3], logs[depositsWanted-2:]...)
|
|
// we purposely miss processing the middle two logs so that the service, re-requests them
|
|
for _, log := range logsToBeProcessed {
|
|
if err := web3Service.ProcessLog(context.Background(), log); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
web3Service.lastRequestedBlock.Set(big.NewInt(int64(log.BlockNumber)))
|
|
}
|
|
|
|
if web3Service.lastReceivedMerkleIndex != int64(depositsWanted-1) {
|
|
t.Errorf("missing logs were not re-requested. Wanted Index %d but got %d", depositsWanted-1, web3Service.lastReceivedMerkleIndex)
|
|
}
|
|
|
|
hook.Reset()
|
|
}
|