mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
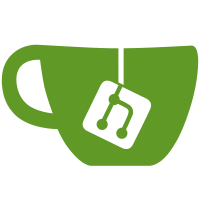
* begin accounts-v2 new * password validation * validator accounts new with eip-2335 keystore * select different wallet type based on enum * clean up code significantly * more robust code structure * check if wallet exists * define read and create wallet methods * fmt * go mod and comment * comment * redundant name * satify gofmt * add instructions with keymanager opts * wrap up create and read wallet functionality * prep for readiness * doc improvements * tests for create and read wallet * update deps * tidy * visibility * gaz * fix up * refactor for proper usage, with wallet and keymanager ifaces * Update validator/flags/flags.go Co-authored-by: Ivan Martinez <ivanthegreatdev@gmail.com> * import * improve structure * wrap up all comments * simplify * lint * Update validator/accounts/v2/cmd.go * viz check * add interface methods as needed * fix build * lint * nishant feedback * simplify structure * add tests for strong password check * all feedback done * ivan feedback * ivan feedback Co-authored-by: Ivan Martinez <ivanthegreatdev@gmail.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
107 lines
2.8 KiB
Go
107 lines
2.8 KiB
Go
package v2
|
|
|
|
import (
|
|
"context"
|
|
"crypto/rand"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"math/big"
|
|
"os"
|
|
"path"
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
v2keymanager "github.com/prysmaticlabs/prysm/validator/keymanager/v2"
|
|
"github.com/prysmaticlabs/prysm/validator/keymanager/v2/direct"
|
|
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
func init() {
|
|
logrus.SetLevel(logrus.DebugLevel)
|
|
logrus.SetOutput(ioutil.Discard)
|
|
}
|
|
|
|
var _ = direct.Wallet(&Wallet{})
|
|
|
|
type mockKeymanager struct {
|
|
configFileContents []byte
|
|
}
|
|
|
|
func (m *mockKeymanager) CreateAccount(ctx context.Context, password string) error {
|
|
return nil
|
|
}
|
|
|
|
func (m *mockKeymanager) MarshalConfigFile(ctx context.Context) ([]byte, error) {
|
|
return m.configFileContents, nil
|
|
}
|
|
|
|
func setupWalletDir(t testing.TB) (string, string) {
|
|
randPath, err := rand.Int(rand.Reader, big.NewInt(1000000))
|
|
if err != nil {
|
|
t.Fatalf("Could not generate random file path: %v", err)
|
|
}
|
|
walletDir := path.Join(testutil.TempDir(), fmt.Sprintf("/%d", randPath))
|
|
if err := os.RemoveAll(walletDir); err != nil {
|
|
t.Fatalf("Failed to remove directory: %v", err)
|
|
}
|
|
passwordsDir := path.Join(testutil.TempDir(), fmt.Sprintf("/%d", randPath))
|
|
if err := os.RemoveAll(passwordsDir); err != nil {
|
|
t.Fatalf("Failed to remove directory: %v", err)
|
|
}
|
|
t.Cleanup(func() {
|
|
if err := os.RemoveAll(walletDir); err != nil {
|
|
t.Fatalf("Failed to remove directory: %v", err)
|
|
}
|
|
if err := os.RemoveAll(passwordsDir); err != nil {
|
|
t.Fatalf("Failed to remove directory: %v", err)
|
|
}
|
|
})
|
|
return walletDir, passwordsDir
|
|
}
|
|
|
|
func TestCreateAndReadWallet(t *testing.T) {
|
|
ctx := context.Background()
|
|
if _, err := CreateWallet(ctx, &WalletConfig{
|
|
PasswordsDir: "",
|
|
WalletDir: "",
|
|
}); err == nil {
|
|
t.Error("Expected error when passing in empty directories, received nil")
|
|
}
|
|
walletDir, passwordsDir := setupWalletDir(t)
|
|
keymanagerKind := v2keymanager.Direct
|
|
wallet, err := CreateWallet(ctx, &WalletConfig{
|
|
PasswordsDir: passwordsDir,
|
|
WalletDir: walletDir,
|
|
KeymanagerKind: keymanagerKind,
|
|
})
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
keymanager := &mockKeymanager{
|
|
configFileContents: []byte("hello-world"),
|
|
}
|
|
keymanagerConfig, err := keymanager.MarshalConfigFile(ctx)
|
|
if err != nil {
|
|
t.Fatalf("Could not marshal keymanager config file: %v", err)
|
|
}
|
|
if err := wallet.WriteKeymanagerConfigToDisk(ctx, keymanagerConfig); err != nil {
|
|
t.Fatalf("Could not write keymanager config file to disk: %v", err)
|
|
}
|
|
|
|
walletPath := path.Join(walletDir, keymanagerKind.String())
|
|
configFilePath := path.Join(walletPath, keymanagerConfigFileName)
|
|
if !fileExists(configFilePath) {
|
|
t.Fatalf("Expected config file to have been created at path: %s", configFilePath)
|
|
}
|
|
|
|
// We should be able to now read the wallet as well.
|
|
if _, err := CreateWallet(ctx, &WalletConfig{
|
|
PasswordsDir: passwordsDir,
|
|
WalletDir: walletDir,
|
|
}); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
}
|