mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 13:18:57 +00:00
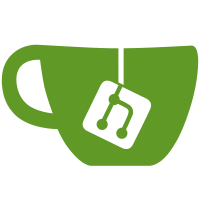
* begin state service * begin on the state trie idea * created beacon state structure * add in the full clone getter * return by value instead * add all setters * new state setters are being completed * arrays roots exposed * close to finishing all these headerssss * functionality complete * added in proto benchmark test * test for compatibility * add test for compat * comments fixed * add clone * add clone * remove underlying copies * make it immutable * integrate it into chainservice * revert * wrap up comments for package * address all comments and godocs * address all comments * clone the pending attestation properly * properly clone remaining items * tests pass fixed bug * begin using it instead of head state * prevent nil pointer exceptions * begin using new struct in db * integrated new type into db package * add proper nil checks * using new state in archiver * refactored much of core * editing all the precompute functions * done with most core refactor * fixed up some bugs in the clone comparisons * append current epoch atts * add missing setters * add new setters * fix other core methods * fix up transition * main service and forkchoice * fix rpc * integrated to powchain * some more changes * fix build * improve processing of deposits * fix error * prevent panic * comment * fix process att * gaz * fix up att process * resolve existing review comments * resolve another batch of gh comments * resolve broken cpt state * revise testutil to use the new state * begin updating the state transition func to pass in more compartmentalized args * finish editing transition function to return errors * block operations pretty much done with refactor * state transition fully refactored * got epoch processing completed * fix build in fork choice * fixing more of the build * fix up broken sync package * it builds nowww it buildssss * revert registry changes * Recompute on Read (#4627) * compute on read * fix up eth1 data votes * looking into slashings bug introduced in core/ * able to advance more slots * add logging * can now sync with testnet yay * remove the leaves algorithm and other merkle imports * expose initialize unsafe funcs * Update beacon-chain/db/kv/state.go * lint Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * More Optimizations for New State (#4641) * map optimization * more optimizations * use a custom hasher * comment * block operations optimizations * Update beacon-chain/state/types.go Co-Authored-By: Raul Jordan <raul@prysmaticlabs.com> * fixed up various operations to use the validator index map access Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * archiver tests pass * fixing cache tests * cache tests passing * edited validator tests * powchain tests passing * halfway thru sync tests * more sync test fixes * add in tests for state/ * working through rpc tests * assignments tests passed * almost done with rpc/beacon tests * resolved painful validator test * fixed up even more tests * resolve tests * fix build * reduce a randao mixes copy * fixes under //beacon-chain/blockchain/... * build //beacon-chain/core/... * fixes * Runtime Optimizations (#4648) * parallelize shuffling * clean up * lint * fix build * use callback to read from registry * fix array roots and size map * new improvements * reduce hash allocs * improved shuffling * terence's review * use different method * raul's comment * new array roots * remove clone in pre-compute * Update beacon-chain/state/types.go Co-Authored-By: Raul Jordan <raul@prysmaticlabs.com> * raul's review * lint * fix build issues * fix visibility Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * fix visibility * build works for all * fix blockchain test * fix a few tests * fix more tests * update validator in slashing * archiver passing * fixed rpc/validator * progress on core tests * resolve broken rpc tests * blockchain tests passed * fix up some tests in core * fix message diff * remove unnecessary save * Save validator after slashing * Update validators one by one * another update * fix everything * fix more precompute tests * fix blocks tests * more elegant fix * more helper fixes * change back ? * fix test * fix skip slot * fix test * reset caches * fix testutil * raceoff fixed * passing * Retrieve cached state in the beginning * lint * Fixed tests part 1 * Fixed rest of the tests * Minor changes to avoid copying, small refactor to reduce deplicated code * Handle att req for slot 0 * New beacon state: Only populate merkle layers as needed, copy merkle layers on copy/clone. (#4689) * Only populate merkle layers as needed, copy merkle layers on copy/clone. * use custom copy * Make maps of correct size * slightly fast, doesn't wait for lock Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> * Target root can't be 0x00 * Don't use cache for current slot (may not be the right fix) * fixed up tests * Remove some copy for init sync. Not sure if it is safe enough for runtime though... testing... * Align with prev logic for process slots cachedState.Slot() < slot * Fix Initial Sync Flag (#4692) * fixes * fix up some test failures due to lack of nil checks * fix up some test failures due to lack of nil checks * fix up imports * revert some changes * imports Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> * resolving further conflicts * Better skip slot cache (#4694) * Return copy of skip slot cache state, disable skip slot cache on sync * fix * Fix pruning * fix up issues with broken tests Co-authored-by: Nishant Das <nish1993@hotmail.com> Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com> Co-authored-by: shayzluf <thezluf@gmail.com> Co-authored-by: terence tsao <terence@prysmaticlabs.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
217 lines
7.3 KiB
Go
217 lines
7.3 KiB
Go
package validator
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"math/big"
|
|
"time"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
stateTrie "github.com/prysmaticlabs/prysm/beacon-chain/state"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/traceutil"
|
|
"go.opencensus.io/trace"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
)
|
|
|
|
var errPubkeyDoesNotExist = errors.New("pubkey does not exist")
|
|
|
|
// ValidatorStatus returns the validator status of the current epoch.
|
|
// The status response can be one of the following:
|
|
// DEPOSITED - validator's deposit has been recognized by Ethereum 1, not yet recognized by Ethereum 2.
|
|
// PENDING - validator is in Ethereum 2's activation queue.
|
|
// ACTIVE - validator is active.
|
|
// EXITING - validator has initiated an an exit request, or has dropped below the ejection balance and is being kicked out.
|
|
// EXITED - validator is no longer validating.
|
|
// SLASHING - validator has been kicked out due to meeting a slashing condition.
|
|
// UNKNOWN_STATUS - validator does not have a known status in the network.
|
|
func (vs *Server) ValidatorStatus(
|
|
ctx context.Context,
|
|
req *ethpb.ValidatorStatusRequest) (*ethpb.ValidatorStatusResponse, error) {
|
|
headState, err := vs.HeadFetcher.HeadState(ctx)
|
|
if err != nil {
|
|
return nil, status.Error(codes.Internal, "Could not get head state")
|
|
}
|
|
return vs.validatorStatus(ctx, req.PublicKey, headState), nil
|
|
}
|
|
|
|
// multipleValidatorStatus returns the validator status response for the set of validators
|
|
// requested by their pub keys.
|
|
func (vs *Server) multipleValidatorStatus(
|
|
ctx context.Context,
|
|
pubkeys [][]byte,
|
|
) (bool, []*ethpb.ValidatorActivationResponse_Status, error) {
|
|
headState, err := vs.HeadFetcher.HeadState(ctx)
|
|
if err != nil {
|
|
return false, nil, err
|
|
}
|
|
activeValidatorExists := false
|
|
statusResponses := make([]*ethpb.ValidatorActivationResponse_Status, len(pubkeys))
|
|
for i, key := range pubkeys {
|
|
if ctx.Err() != nil {
|
|
return false, nil, ctx.Err()
|
|
}
|
|
status := vs.validatorStatus(ctx, key, headState)
|
|
if status == nil {
|
|
continue
|
|
}
|
|
resp := ðpb.ValidatorActivationResponse_Status{
|
|
Status: status,
|
|
PublicKey: key,
|
|
}
|
|
statusResponses[i] = resp
|
|
if status.Status == ethpb.ValidatorStatus_ACTIVE {
|
|
activeValidatorExists = true
|
|
}
|
|
}
|
|
|
|
return activeValidatorExists, statusResponses, nil
|
|
}
|
|
|
|
func (vs *Server) validatorStatus(ctx context.Context, pubKey []byte, headState *stateTrie.BeaconState) *ethpb.ValidatorStatusResponse {
|
|
ctx, span := trace.StartSpan(ctx, "validatorServer.validatorStatus")
|
|
defer span.End()
|
|
|
|
resp := ðpb.ValidatorStatusResponse{
|
|
Status: ethpb.ValidatorStatus_UNKNOWN_STATUS,
|
|
ActivationEpoch: int64(params.BeaconConfig().FarFutureEpoch),
|
|
}
|
|
vStatus, idx, err := vs.retrieveStatusFromState(ctx, pubKey, headState)
|
|
if err != nil && err != errPubkeyDoesNotExist {
|
|
traceutil.AnnotateError(span, err)
|
|
return resp
|
|
}
|
|
resp.Status = vStatus
|
|
if err != errPubkeyDoesNotExist {
|
|
val, err := headState.ValidatorAtIndex(idx)
|
|
if err != nil {
|
|
traceutil.AnnotateError(span, err)
|
|
return resp
|
|
}
|
|
resp.ActivationEpoch = int64(val.ActivationEpoch)
|
|
}
|
|
|
|
// If no connection to ETH1, the deposit block number or position in queue cannot be determined.
|
|
if !vs.Eth1InfoFetcher.IsConnectedToETH1() {
|
|
log.Warn("Not connected to ETH1. Cannot determine validator ETH1 deposit block number")
|
|
return resp
|
|
}
|
|
|
|
_, eth1BlockNumBigInt := vs.DepositFetcher.DepositByPubkey(ctx, pubKey)
|
|
if eth1BlockNumBigInt == nil { // No deposit found in ETH1.
|
|
return resp
|
|
}
|
|
|
|
if resp.Status == ethpb.ValidatorStatus_UNKNOWN_STATUS {
|
|
resp.Status = ethpb.ValidatorStatus_DEPOSITED
|
|
}
|
|
|
|
resp.Eth1DepositBlockNumber = eth1BlockNumBigInt.Uint64()
|
|
|
|
depositBlockSlot, err := vs.depositBlockSlot(ctx, eth1BlockNumBigInt, headState)
|
|
if err != nil {
|
|
return resp
|
|
}
|
|
resp.DepositInclusionSlot = int64(depositBlockSlot)
|
|
|
|
// If validator has been activated at any point, they are not in the queue so we can return
|
|
// the request early. Also if the validator has exited,slashed or initiated its exit
|
|
// we return the request early too. We only proceed if its status is pending active
|
|
// Additionally, if idx is zero (default return value) then we know this
|
|
// validator cannot be in the queue either.
|
|
if resp.Status != ethpb.ValidatorStatus_PENDING || idx == 0 {
|
|
return resp
|
|
}
|
|
|
|
var lastActivatedValidatorIdx uint64
|
|
for j := headState.NumValidators() - 1; j >= 0; j-- {
|
|
val, err := headState.ValidatorAtIndex(uint64(j))
|
|
if err != nil {
|
|
return resp
|
|
}
|
|
if helpers.IsActiveValidator(val, helpers.CurrentEpoch(headState)) {
|
|
lastActivatedValidatorIdx = uint64(j)
|
|
break
|
|
}
|
|
}
|
|
// Our position in the activation queue is the above index - our validator index.
|
|
if lastActivatedValidatorIdx < idx {
|
|
resp.PositionInActivationQueue = int64(idx - lastActivatedValidatorIdx)
|
|
}
|
|
|
|
return resp
|
|
}
|
|
|
|
func (vs *Server) retrieveStatusFromState(
|
|
ctx context.Context,
|
|
pubKey []byte,
|
|
headState *stateTrie.BeaconState,
|
|
) (ethpb.ValidatorStatus, uint64, error) {
|
|
if headState == nil {
|
|
return ethpb.ValidatorStatus_UNKNOWN_STATUS, 0, errors.New("head state does not exist")
|
|
}
|
|
idx, ok, err := vs.BeaconDB.ValidatorIndex(ctx, pubKey)
|
|
if err != nil {
|
|
return ethpb.ValidatorStatus_UNKNOWN_STATUS, 0, err
|
|
}
|
|
if !ok || int(idx) >= headState.NumValidators() {
|
|
return ethpb.ValidatorStatus_UNKNOWN_STATUS, 0, errPubkeyDoesNotExist
|
|
}
|
|
return vs.assignmentStatus(idx, headState), idx, nil
|
|
}
|
|
|
|
func (vs *Server) assignmentStatus(validatorIdx uint64, beaconState *stateTrie.BeaconState) ethpb.ValidatorStatus {
|
|
validator, err := beaconState.ValidatorAtIndex(validatorIdx)
|
|
if err != nil {
|
|
return ethpb.ValidatorStatus_UNKNOWN_STATUS
|
|
}
|
|
currentEpoch := helpers.CurrentEpoch(beaconState)
|
|
farFutureEpoch := params.BeaconConfig().FarFutureEpoch
|
|
|
|
if validator == nil {
|
|
return ethpb.ValidatorStatus_UNKNOWN_STATUS
|
|
}
|
|
if currentEpoch < validator.ActivationEligibilityEpoch {
|
|
return ethpb.ValidatorStatus_DEPOSITED
|
|
}
|
|
if currentEpoch < validator.ActivationEpoch {
|
|
return ethpb.ValidatorStatus_PENDING
|
|
}
|
|
if validator.ExitEpoch == farFutureEpoch {
|
|
return ethpb.ValidatorStatus_ACTIVE
|
|
}
|
|
if currentEpoch < validator.ExitEpoch {
|
|
if validator.Slashed {
|
|
return ethpb.ValidatorStatus_SLASHING
|
|
}
|
|
return ethpb.ValidatorStatus_EXITING
|
|
}
|
|
return ethpb.ValidatorStatus_EXITED
|
|
}
|
|
|
|
func (vs *Server) depositBlockSlot(ctx context.Context, eth1BlockNumBigInt *big.Int, beaconState *stateTrie.BeaconState) (uint64, error) {
|
|
var depositBlockSlot uint64
|
|
blockTimeStamp, err := vs.BlockFetcher.BlockTimeByHeight(ctx, eth1BlockNumBigInt)
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
followTime := time.Duration(params.BeaconConfig().Eth1FollowDistance*params.BeaconConfig().GoerliBlockTime) * time.Second
|
|
eth1UnixTime := time.Unix(int64(blockTimeStamp), 0).Add(followTime)
|
|
|
|
votingPeriod := time.Duration(params.BeaconConfig().SlotsPerEth1VotingPeriod*params.BeaconConfig().SecondsPerSlot) * time.Second
|
|
timeToInclusion := eth1UnixTime.Add(votingPeriod)
|
|
|
|
eth2Genesis := time.Unix(int64(beaconState.GenesisTime()), 0)
|
|
|
|
if eth2Genesis.After(timeToInclusion) {
|
|
depositBlockSlot = 0
|
|
} else {
|
|
eth2TimeDifference := timeToInclusion.Sub(eth2Genesis).Seconds()
|
|
depositBlockSlot = uint64(eth2TimeDifference) / params.BeaconConfig().SecondsPerSlot
|
|
}
|
|
|
|
return depositBlockSlot, nil
|
|
}
|