mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-31 23:41:22 +00:00
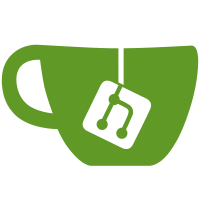
* starting on patch * finish determining all required patches * properly redefine the patch rules * new patch * rem double semicolon * fix patch file * Merge branch 'master' of github.com:prysmaticlabs/prysm into deprecate-eth-protos * building the deps * test target passes using ethereumapis * compile gateway * attempting to build everything * e2e use ethereumapis * more fixes for slasher * other item * getting closer to compiling slasher * build slasher package * Merge branch 'master' into deprecate-eth-protos * Merge branch 'master' into deprecate-eth-protos * fix benches * lint gazelle * Merge branch 'deprecate-eth-protos' of github.com:prysmaticlabs/prysm into deprecate-eth-protos * proper gateway * lint * Merge branch 'master' into deprecate-eth-protos * fix build * Merge branch 'deprecate-eth-protos' of github.com:prysmaticlabs/prysm into deprecate-eth-protos * use swag * resolve * ignore change * include new patch changes * fix test * builds * fix e2e * gaz
196 lines
5.1 KiB
Go
196 lines
5.1 KiB
Go
package db
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
)
|
|
|
|
type testStruct struct {
|
|
iA *ethpb.IndexedAttestation
|
|
}
|
|
|
|
var tests []testStruct
|
|
|
|
func init() {
|
|
tests = []testStruct{
|
|
{
|
|
iA: ðpb.IndexedAttestation{Signature: []byte("let me in"), CustodyBit_0Indices: []uint64{0}, Data: ðpb.AttestationData{
|
|
Source: ðpb.Checkpoint{Epoch: 0},
|
|
Target: ðpb.Checkpoint{Epoch: 1},
|
|
}},
|
|
},
|
|
{
|
|
iA: ðpb.IndexedAttestation{Signature: []byte("let me in 2nd"), CustodyBit_0Indices: []uint64{1, 2}, Data: ðpb.AttestationData{
|
|
Source: ðpb.Checkpoint{Epoch: 0},
|
|
Target: ðpb.Checkpoint{Epoch: 2},
|
|
}},
|
|
},
|
|
{
|
|
iA: ðpb.IndexedAttestation{Signature: []byte("let me in 3rd"), CustodyBit_0Indices: []uint64{0}, Data: ðpb.AttestationData{
|
|
Source: ðpb.Checkpoint{Epoch: 1},
|
|
Target: ðpb.Checkpoint{Epoch: 2},
|
|
}},
|
|
},
|
|
}
|
|
}
|
|
|
|
func TestNilDBHistoryIdxAtt(t *testing.T) {
|
|
db := SetupSlasherDB(t)
|
|
defer TeardownSlasherDB(t, db)
|
|
|
|
epoch := uint64(1)
|
|
validatorID := uint64(1)
|
|
|
|
hasIdxAtt := db.HasIndexedAttestation(epoch, validatorID)
|
|
if hasIdxAtt {
|
|
t.Fatal("HasIndexedAttestation should return false")
|
|
}
|
|
|
|
idxAtt, err := db.IndexedAttestation(epoch, validatorID)
|
|
if err != nil {
|
|
t.Fatalf("failed to get indexed attestation: %v", err)
|
|
}
|
|
if idxAtt != nil {
|
|
t.Fatalf("get should return nil for a non existent key")
|
|
}
|
|
}
|
|
|
|
func TestSaveIdxAtt(t *testing.T) {
|
|
db := SetupSlasherDB(t)
|
|
defer TeardownSlasherDB(t, db)
|
|
|
|
for _, tt := range tests {
|
|
err := db.SaveIndexedAttestation(tt.iA)
|
|
if err != nil {
|
|
t.Fatalf("save indexed attestation failed: %v", err)
|
|
}
|
|
|
|
iAarray, err := db.IndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
if err != nil {
|
|
t.Fatalf("failed to get indexed attestation: %v", err)
|
|
}
|
|
|
|
if iAarray == nil || !reflect.DeepEqual(iAarray[0], tt.iA) {
|
|
t.Fatalf("get should return indexed attestation: %v", iAarray)
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
func TestDeleteHistoryIdxAtt(t *testing.T) {
|
|
db := SetupSlasherDB(t)
|
|
defer TeardownSlasherDB(t, db)
|
|
|
|
for _, tt := range tests {
|
|
|
|
err := db.SaveIndexedAttestation(tt.iA)
|
|
if err != nil {
|
|
t.Fatalf("save indexed attestation failed: %v", err)
|
|
}
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
iAarray, err := db.IndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
if err != nil {
|
|
t.Fatalf("failed to get index attestation: %v", err)
|
|
}
|
|
|
|
if iAarray == nil || !reflect.DeepEqual(iAarray[0], tt.iA) {
|
|
t.Fatalf("get should return indexed attestation: %v", iAarray)
|
|
}
|
|
err = db.DeleteIndexedAttestation(tt.iA)
|
|
if err != nil {
|
|
t.Fatalf("delete index attestation failed: %v", err)
|
|
}
|
|
iAarray, err = db.IndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
hasA := db.HasIndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(iAarray) != 0 {
|
|
t.Errorf("Expected index attestation to have been deleted, received: %v", iAarray)
|
|
}
|
|
if hasA {
|
|
t.Errorf("Expected indexed attestation indexes list to be deleted, received: %v", hasA)
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
func TestHasIdxAtt(t *testing.T) {
|
|
db := SetupSlasherDB(t)
|
|
defer TeardownSlasherDB(t, db)
|
|
|
|
for _, tt := range tests {
|
|
|
|
found := db.HasIndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
if found {
|
|
t.Fatal("has indexed attestation should return false for indexed attestations that are not in db")
|
|
}
|
|
err := db.SaveIndexedAttestation(tt.iA)
|
|
if err != nil {
|
|
t.Fatalf("save indexed attestation failed: %v", err)
|
|
}
|
|
}
|
|
for _, tt := range tests {
|
|
|
|
found := db.HasIndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
|
|
if !found {
|
|
t.Fatal("has indexed attestation should return true")
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestPruneHistoryIdxAtt(t *testing.T) {
|
|
db := SetupSlasherDB(t)
|
|
defer TeardownSlasherDB(t, db)
|
|
|
|
for _, tt := range tests {
|
|
err := db.SaveIndexedAttestation(tt.iA)
|
|
if err != nil {
|
|
t.Fatalf("save indexed attestation failed: %v", err)
|
|
}
|
|
|
|
iAarray, err := db.IndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
if err != nil {
|
|
t.Fatalf("failed to get indexed attestation: %v", err)
|
|
}
|
|
|
|
if iAarray == nil || !reflect.DeepEqual(iAarray[0], tt.iA) {
|
|
t.Fatalf("get should return bh: %v", iAarray)
|
|
}
|
|
}
|
|
currentEpoch := uint64(3)
|
|
historyToKeep := uint64(1)
|
|
err := db.pruneAttHistory(currentEpoch, historyToKeep)
|
|
if err != nil {
|
|
t.Fatalf("failed to prune: %v", err)
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
iAarray, err := db.IndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
if err != nil {
|
|
t.Fatalf("failed to get indexed attestation: %v", err)
|
|
}
|
|
hasIa := db.HasIndexedAttestation(tt.iA.Data.Target.Epoch, tt.iA.CustodyBit_0Indices[0])
|
|
|
|
if tt.iA.Data.Source.Epoch > currentEpoch-historyToKeep {
|
|
if iAarray == nil || !reflect.DeepEqual(iAarray[0], tt.iA) {
|
|
t.Fatalf("get should return indexed attestation: %v", iAarray)
|
|
}
|
|
if !hasIa {
|
|
t.Fatalf("get should have indexed attestation for epoch: %v", iAarray)
|
|
}
|
|
} else {
|
|
if iAarray != nil || hasIa {
|
|
t.Fatalf("indexed attestation should have been pruned: %v has attestation: %v", iAarray, hasIa)
|
|
}
|
|
}
|
|
|
|
}
|
|
}
|