mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-08 10:41:19 +00:00
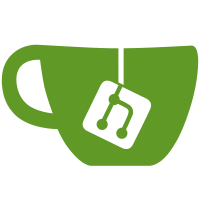
Former-commit-id: 8b8b695ddc0a39bfe6a536fd7b7f40d74c4105da [formerly 829d481af0eebd1647a34006018761e3e64c5e3e] Former-commit-id: 1d607fcb8fae1327c346bdf3557ec40a8816a09b
50 lines
1.4 KiB
Go
50 lines
1.4 KiB
Go
package notary
|
|
|
|
import (
|
|
"github.com/ethereum/go-ethereum/log"
|
|
"github.com/ethereum/go-ethereum/sharding/database"
|
|
"github.com/ethereum/go-ethereum/sharding/node"
|
|
)
|
|
|
|
// Notary holds functionality required to run a collation notary
|
|
// in a sharded system. Must satisfy the Service interface defined in
|
|
// sharding/service.go.
|
|
type Notary struct {
|
|
node node.Node
|
|
shardDB database.ShardBackend
|
|
}
|
|
|
|
// NewNotary creates a new notary instance.
|
|
func NewNotary(node node.Node) (*Notary, error) {
|
|
// Initializes a shardDB that writes to disk at /path/to/datadir/shardchaindata.
|
|
// This DB can be used by the Notary service to create Shard struct
|
|
// instances.
|
|
shardDB, err := database.NewShardDB(node.DataDirFlag(), "shardchaindata")
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &Notary{node, shardDB}, nil
|
|
}
|
|
|
|
// Start the main routine for a notary.
|
|
func (n *Notary) Start() error {
|
|
log.Info("Starting notary service")
|
|
|
|
// TODO: handle this better through goroutines. Right now, these methods
|
|
// have their own nested channels and goroutines within them. We need
|
|
// to make this as flat as possible at the Notary layer.
|
|
if n.node.DepositFlagSet() {
|
|
if err := joinNotaryPool(n.node); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
return subscribeBlockHeaders(n.node)
|
|
}
|
|
|
|
// Stop the main loop for notarizing collations.
|
|
func (n *Notary) Stop() error {
|
|
log.Info("Stopping notary service")
|
|
return nil
|
|
}
|