mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
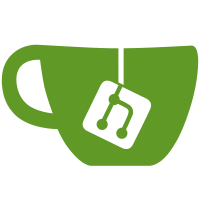
* add main.go * interop readme * proper visibility * standardize and abstract into simpler funcs * formatting * no os pkg * add test * no panics anywhere, properly and nicely handle errors * proper comments * fix broken test * readme * comment * recommend ssz * install * tool now works * README * build * readme * 64 validators * rem print * register the no powchain flag * work on mock eth1 start * common interface * getting closer with the interface defs * only two uses of powchain * remove powchain dependency * remove powchain dependency * common powchain interface * proper comment in case of flag * proper args into rpc services * rename fields * pass in mock flag into RPC * conforms to iface * use client instead of block fetcher iface * broken tests * block fetcher * finalized * resolved broken build * fix build * comment * fix tests * tests pass * resolved confs * took them out * rename into smaller interfaces * resolve some confs * ensure tests pass * properly utilize mock instead of localized mock * res lint * lint * finish test for mock eth1data * run gazelle * include flag again * fix broken build * disable powchain * dont dial eth1 nodes * reenable pow * use smaller interfaces, standardize naming * abstract mock into its own package * faulty mock lint * fix stutter in lint * rpc tests all passing * use mocks for operations * no more mocks in the entire rpc package * no mock * viz * testonly
98 lines
2.8 KiB
Go
98 lines
2.8 KiB
Go
package rpc
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"testing"
|
|
|
|
mock "github.com/prysmaticlabs/prysm/beacon-chain/blockchain/testing"
|
|
mockSync "github.com/prysmaticlabs/prysm/beacon-chain/sync/testing"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
"github.com/sirupsen/logrus"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
func init() {
|
|
logrus.SetLevel(logrus.DebugLevel)
|
|
logrus.SetOutput(ioutil.Discard)
|
|
}
|
|
|
|
func TestLifecycle_OK(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
rpcService := NewService(context.Background(), &Config{
|
|
Port: "7348",
|
|
CertFlag: "alice.crt",
|
|
KeyFlag: "alice.key",
|
|
SyncService: &mockSync.Sync{IsSyncing: false},
|
|
BlockReceiver: &mock.ChainService{},
|
|
AttestationReceiver: &mock.ChainService{},
|
|
HeadFetcher: &mock.ChainService{},
|
|
StateFeedListener: &mock.ChainService{},
|
|
})
|
|
|
|
rpcService.Start()
|
|
|
|
testutil.AssertLogsContain(t, hook, "Starting service")
|
|
testutil.AssertLogsContain(t, hook, "Listening on port")
|
|
|
|
rpcService.Stop()
|
|
testutil.AssertLogsContain(t, hook, "Stopping service")
|
|
|
|
}
|
|
|
|
func TestRPC_BadEndpoint(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
|
|
rpcService := NewService(context.Background(), &Config{
|
|
Port: "ralph merkle!!!",
|
|
SyncService: &mockSync.Sync{IsSyncing: false},
|
|
BlockReceiver: &mock.ChainService{},
|
|
AttestationReceiver: &mock.ChainService{},
|
|
HeadFetcher: &mock.ChainService{},
|
|
StateFeedListener: &mock.ChainService{},
|
|
})
|
|
|
|
testutil.AssertLogsDoNotContain(t, hook, "Could not listen to port in Start()")
|
|
testutil.AssertLogsDoNotContain(t, hook, "Could not load TLS keys")
|
|
testutil.AssertLogsDoNotContain(t, hook, "Could not serve gRPC")
|
|
|
|
rpcService.Start()
|
|
|
|
testutil.AssertLogsContain(t, hook, "Starting service")
|
|
testutil.AssertLogsContain(t, hook, "Could not listen to port in Start()")
|
|
|
|
rpcService.Stop()
|
|
}
|
|
|
|
func TestStatus_CredentialError(t *testing.T) {
|
|
credentialErr := errors.New("credentialError")
|
|
s := &Service{credentialError: credentialErr}
|
|
|
|
if err := s.Status(); err != s.credentialError {
|
|
t.Errorf("Wanted: %v, got: %v", s.credentialError, s.Status())
|
|
}
|
|
}
|
|
|
|
func TestRPC_InsecureEndpoint(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
rpcService := NewService(context.Background(), &Config{
|
|
Port: "7777",
|
|
SyncService: &mockSync.Sync{IsSyncing: false},
|
|
BlockReceiver: &mock.ChainService{},
|
|
AttestationReceiver: &mock.ChainService{},
|
|
HeadFetcher: &mock.ChainService{},
|
|
StateFeedListener: &mock.ChainService{},
|
|
})
|
|
|
|
rpcService.Start()
|
|
|
|
testutil.AssertLogsContain(t, hook, "Starting service")
|
|
testutil.AssertLogsContain(t, hook, fmt.Sprint("Listening on port"))
|
|
testutil.AssertLogsContain(t, hook, "You are using an insecure gRPC connection")
|
|
|
|
rpcService.Stop()
|
|
testutil.AssertLogsContain(t, hook, "Stopping service")
|
|
}
|