mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-07 10:12:19 +00:00
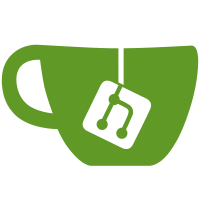
* move state protos * regen ssz * edit v1 code * fix imports * building * beacon chain builds * validator and shared builds * fuzz builds * changes * spectest builds * tools build * remove import cycle * generate ssz * pcli * gaz * kafka * gaz
81 lines
2.8 KiB
Go
81 lines
2.8 KiB
Go
package debug
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/rpc/statefetcher"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/eth/v1"
|
|
statepb "github.com/prysmaticlabs/prysm/proto/eth/v1"
|
|
"go.opencensus.io/trace"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
"google.golang.org/protobuf/types/known/emptypb"
|
|
)
|
|
|
|
// GetBeaconState returns the full beacon state for a given state id.
|
|
func (ds *Server) GetBeaconState(ctx context.Context, req *ethpb.StateRequest) (*statepb.BeaconStateResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "beaconv1.GetBeaconState")
|
|
defer span.End()
|
|
|
|
state, err := ds.StateFetcher.State(ctx, req.StateId)
|
|
if err != nil {
|
|
if stateNotFoundErr, ok := err.(*statefetcher.StateNotFoundError); ok {
|
|
return nil, status.Errorf(codes.NotFound, "State not found: %v", stateNotFoundErr)
|
|
} else if parseErr, ok := err.(*statefetcher.StateIdParseError); ok {
|
|
return nil, status.Errorf(codes.InvalidArgument, "Invalid state ID: %v", parseErr)
|
|
}
|
|
return nil, status.Errorf(codes.Internal, "Invalid state ID: %v", err)
|
|
}
|
|
|
|
protoState, err := state.ToProto()
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not convert state to proto: %v", err)
|
|
}
|
|
|
|
return &statepb.BeaconStateResponse{
|
|
Data: protoState,
|
|
}, nil
|
|
}
|
|
|
|
// GetBeaconStateSSZ returns the SSZ-serialized version of the full beacon state object for given stateId.
|
|
func (ds *Server) GetBeaconStateSSZ(ctx context.Context, req *ethpb.StateRequest) (*statepb.BeaconStateSSZResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "beaconv1.GetBeaconStateSSZ")
|
|
defer span.End()
|
|
|
|
state, err := ds.StateFetcher.State(ctx, req.StateId)
|
|
if err != nil {
|
|
if stateNotFoundErr, ok := err.(*statefetcher.StateNotFoundError); ok {
|
|
return nil, status.Errorf(codes.NotFound, "State not found: %v", stateNotFoundErr)
|
|
} else if parseErr, ok := err.(*statefetcher.StateIdParseError); ok {
|
|
return nil, status.Errorf(codes.InvalidArgument, "Invalid state ID: %v", parseErr)
|
|
}
|
|
return nil, status.Errorf(codes.Internal, "Invalid state ID: %v", err)
|
|
}
|
|
|
|
sszState, err := state.MarshalSSZ()
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not marshal state into SSZ: %v", err)
|
|
}
|
|
|
|
return &statepb.BeaconStateSSZResponse{Data: sszState}, nil
|
|
}
|
|
|
|
// ListForkChoiceHeads retrieves the fork choice leaves for the current head.
|
|
func (ds *Server) ListForkChoiceHeads(ctx context.Context, _ *emptypb.Empty) (*ethpb.ForkChoiceHeadsResponse, error) {
|
|
ctx, span := trace.StartSpan(ctx, "debugv1.ListForkChoiceHeads")
|
|
defer span.End()
|
|
|
|
headRoots, headSlots := ds.HeadFetcher.ChainHeads()
|
|
resp := ðpb.ForkChoiceHeadsResponse{
|
|
Data: make([]*ethpb.ForkChoiceHead, len(headRoots)),
|
|
}
|
|
for i := range headRoots {
|
|
resp.Data[i] = ðpb.ForkChoiceHead{
|
|
Root: headRoots[i][:],
|
|
Slot: headSlots[i],
|
|
}
|
|
}
|
|
|
|
return resp, nil
|
|
}
|