mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
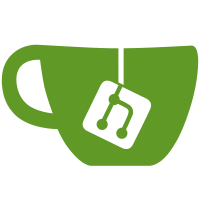
* Removed * All tests pass * Gaz * Removed new lines * A few more lines... * I think i got them all * and I didnt : ) * Could this be last...
67 lines
1.9 KiB
Go
67 lines
1.9 KiB
Go
package testutil
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/binary"
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/go-ssz"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
)
|
|
|
|
func TestBlockSignature(t *testing.T) {
|
|
beaconState, privKeys := DeterministicGenesisState(t, 100)
|
|
block, err := GenerateFullBlock(beaconState, privKeys, nil, 0)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
beaconState.Slot++
|
|
proposerIdx, err := helpers.BeaconProposerIndex(beaconState)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
beaconState.Slot--
|
|
signingRoot, err := ssz.SigningRoot(block)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
epoch := helpers.SlotToEpoch(block.Slot)
|
|
domain := helpers.Domain(beaconState.Fork, epoch, params.BeaconConfig().DomainBeaconProposer)
|
|
blockSig := privKeys[proposerIdx].Sign(signingRoot[:], domain).Marshal()
|
|
|
|
signature, err := BlockSignature(beaconState, block, privKeys)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
if !bytes.Equal(blockSig[:], signature.Marshal()) {
|
|
t.Errorf("Expected block signatures to be equal, received %#x != %#x", blockSig[:], signature.Marshal())
|
|
}
|
|
}
|
|
|
|
func TestRandaoReveal(t *testing.T) {
|
|
beaconState, privKeys := DeterministicGenesisState(t, 100)
|
|
|
|
epoch := helpers.CurrentEpoch(beaconState)
|
|
randaoReveal, err := RandaoReveal(beaconState, epoch, privKeys)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
proposerIdx, err := helpers.BeaconProposerIndex(beaconState)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
buf := make([]byte, 32)
|
|
binary.LittleEndian.PutUint64(buf, epoch)
|
|
domain := helpers.Domain(beaconState.Fork, epoch, params.BeaconConfig().DomainRandao)
|
|
// We make the previous validator's index sign the message instead of the proposer.
|
|
epochSignature := privKeys[proposerIdx].Sign(buf, domain).Marshal()
|
|
|
|
if !bytes.Equal(randaoReveal[:], epochSignature[:]) {
|
|
t.Errorf("Expected randao reveals to be equal, received %#x != %#x", randaoReveal[:], epochSignature[:])
|
|
}
|
|
}
|