mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 19:40:37 +00:00
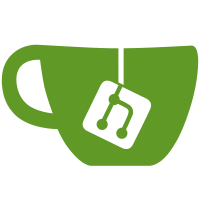
* beacon-chain/state/attenstation: alloc +1 items for append Signed-off-by: jsvisa <delweng@gmail.com> * beacon-chain/state/eth1: alloc +1 items for append Signed-off-by: jsvisa <delweng@gmail.com> * beacon-chain/state/misc: alloc +1 items for append Signed-off-by: jsvisa <delweng@gmail.com> * beacon-chain/state/participation: alloc +1 items for append Signed-off-by: jsvisa <delweng@gmail.com> * beacon-chain/state/validator: alloc +1 items for append Signed-off-by: jsvisa <delweng@gmail.com> * Add some benchmarks * Evaluate append vs copy. Apply results * fix copy issue * revert copy changes from a5ba8d4352f647ad384981264cb6e0553481f23b --------- Signed-off-by: jsvisa <delweng@gmail.com> Co-authored-by: Nishant Das <nishdas93@gmail.com> Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
64 lines
1.9 KiB
Go
64 lines
1.9 KiB
Go
package state_native
|
|
|
|
import (
|
|
"github.com/prysmaticlabs/prysm/v4/beacon-chain/state/state-native/types"
|
|
"github.com/prysmaticlabs/prysm/v4/beacon-chain/state/stateutil"
|
|
ethpb "github.com/prysmaticlabs/prysm/v4/proto/prysm/v1alpha1"
|
|
)
|
|
|
|
// SetEth1Data for the beacon state.
|
|
func (b *BeaconState) SetEth1Data(val *ethpb.Eth1Data) error {
|
|
b.lock.Lock()
|
|
defer b.lock.Unlock()
|
|
|
|
b.eth1Data = val
|
|
b.markFieldAsDirty(types.Eth1Data)
|
|
return nil
|
|
}
|
|
|
|
// SetEth1DataVotes for the beacon state. Updates the entire
|
|
// list to a new value by overwriting the previous one.
|
|
func (b *BeaconState) SetEth1DataVotes(val []*ethpb.Eth1Data) error {
|
|
b.lock.Lock()
|
|
defer b.lock.Unlock()
|
|
|
|
b.sharedFieldReferences[types.Eth1DataVotes].MinusRef()
|
|
b.sharedFieldReferences[types.Eth1DataVotes] = stateutil.NewRef(1)
|
|
|
|
b.eth1DataVotes = val
|
|
b.markFieldAsDirty(types.Eth1DataVotes)
|
|
b.rebuildTrie[types.Eth1DataVotes] = true
|
|
return nil
|
|
}
|
|
|
|
// SetEth1DepositIndex for the beacon state.
|
|
func (b *BeaconState) SetEth1DepositIndex(val uint64) error {
|
|
b.lock.Lock()
|
|
defer b.lock.Unlock()
|
|
|
|
b.eth1DepositIndex = val
|
|
b.markFieldAsDirty(types.Eth1DepositIndex)
|
|
return nil
|
|
}
|
|
|
|
// AppendEth1DataVotes for the beacon state. Appends the new value
|
|
// to the end of list.
|
|
func (b *BeaconState) AppendEth1DataVotes(val *ethpb.Eth1Data) error {
|
|
b.lock.Lock()
|
|
defer b.lock.Unlock()
|
|
|
|
votes := b.eth1DataVotes
|
|
if b.sharedFieldReferences[types.Eth1DataVotes].Refs() > 1 {
|
|
// Copy elements in underlying array by reference.
|
|
votes = make([]*ethpb.Eth1Data, 0, len(b.eth1DataVotes)+1)
|
|
votes = append(votes, b.eth1DataVotes...)
|
|
b.sharedFieldReferences[types.Eth1DataVotes].MinusRef()
|
|
b.sharedFieldReferences[types.Eth1DataVotes] = stateutil.NewRef(1)
|
|
}
|
|
|
|
b.eth1DataVotes = append(votes, val)
|
|
b.markFieldAsDirty(types.Eth1DataVotes)
|
|
b.addDirtyIndices(types.Eth1DataVotes, []uint64{uint64(len(b.eth1DataVotes) - 1)})
|
|
return nil
|
|
}
|