mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
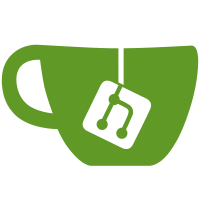
* Remove Feature Flag From Prater (#12082) * Use Epoch boundary cache to retrieve balances (#12083) * Use Epoch boundary cache to retrieve balances * save boundary states before inserting to forkchoice * move up last block save * remove boundary checks on balances * fix ordering --------- Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> * create the log file along with its parent directory if not present * only give ReadWritePermissions to the log file * use io/file package to create the parent directories * fix ci related issues * add regression tests * run gazelle * fix tests * remove print statements * gazelle * Remove failing test for MkdirAll, this failure is not expected --------- Co-authored-by: Nishant Das <nishdas93@gmail.com> Co-authored-by: Potuz <potuz@prysmaticlabs.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
64 lines
2.0 KiB
Go
64 lines
2.0 KiB
Go
package logs
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"testing"
|
|
|
|
"github.com/prysmaticlabs/prysm/v5/testing/require"
|
|
)
|
|
|
|
var urltests = []struct {
|
|
url string
|
|
maskedUrl string
|
|
}{
|
|
{"https://a:b@xyz.net", "https://***@xyz.net"},
|
|
{"https://eth-goerli.alchemyapi.io/v2/tOZG5mjl3.zl_nZdZTNIBUzsDq62R_dkOtY",
|
|
"https://eth-goerli.alchemyapi.io/***"},
|
|
{"https://google.com/search?q=golang", "https://google.com/***"},
|
|
{"https://user@example.com/foo%2fbar", "https://***@example.com/***"},
|
|
{"http://john@example.com/#x/y%2Fz", "http://***@example.com/#***"},
|
|
{"https://me:pass@example.com/foo/bar?x=1&y=2", "https://***@example.com/***"},
|
|
}
|
|
|
|
func TestMaskCredentialsLogging(t *testing.T) {
|
|
for _, test := range urltests {
|
|
require.Equal(t, MaskCredentialsLogging(test.url), test.maskedUrl)
|
|
}
|
|
}
|
|
|
|
func TestConfigurePersistantLogging(t *testing.T) {
|
|
testParentDir := t.TempDir()
|
|
|
|
// 1. Test creation of file in an existing parent directory
|
|
logFileName := "test.log"
|
|
existingDirectory := "test-1-existing-testing-dir"
|
|
|
|
err := ConfigurePersistentLogging(fmt.Sprintf("%s/%s/%s", testParentDir, existingDirectory, logFileName))
|
|
require.NoError(t, err)
|
|
|
|
// 2. Test creation of file along with parent directory
|
|
nonExistingDirectory := "test-2-non-existing-testing-dir"
|
|
|
|
err = ConfigurePersistentLogging(fmt.Sprintf("%s/%s/%s", testParentDir, nonExistingDirectory, logFileName))
|
|
require.NoError(t, err)
|
|
|
|
// 3. Test creation of file in an existing parent directory with a non-existing sub-directory
|
|
existingDirectory = "test-3-existing-testing-dir"
|
|
nonExistingSubDirectory := "test-3-non-existing-sub-dir"
|
|
err = os.Mkdir(fmt.Sprintf("%s/%s", testParentDir, existingDirectory), 0700)
|
|
if err != nil {
|
|
return
|
|
}
|
|
|
|
err = ConfigurePersistentLogging(fmt.Sprintf("%s/%s/%s/%s", testParentDir, existingDirectory, nonExistingSubDirectory, logFileName))
|
|
require.NoError(t, err)
|
|
|
|
//4. Create log file in a directory without 700 permissions
|
|
existingDirectory = "test-4-existing-testing-dir"
|
|
err = os.Mkdir(fmt.Sprintf("%s/%s", testParentDir, existingDirectory), 0750)
|
|
if err != nil {
|
|
return
|
|
}
|
|
}
|