mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-09 19:21:19 +00:00
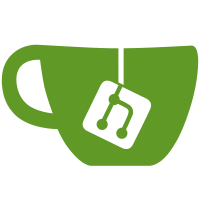
Former-commit-id: f7344b7d3fb2fa07f0fd421c5ed3721f6c7a9258 [formerly e678ab2f1681d9492c0e1b142cd06ee08a462fdb] Former-commit-id: d942da3d5cfcde921bebb149c26edd5c4ed178dd
77 lines
1.8 KiB
Go
77 lines
1.8 KiB
Go
// Package database provides several constructs including a simple in-memory database.
|
|
// This should not be used for production, but would be a helpful interim
|
|
// solution for development.
|
|
package database
|
|
|
|
import (
|
|
"fmt"
|
|
"path/filepath"
|
|
|
|
"github.com/ethereum/go-ethereum/ethdb"
|
|
sharedDB "github.com/prysmaticlabs/prysm/shared/database"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
var log = logrus.WithField("prefix", "db")
|
|
|
|
// ShardDB defines a service for the sharding system's persistent storage.
|
|
type ShardDB struct {
|
|
inmemory bool
|
|
dataDir string
|
|
name string
|
|
cache int
|
|
handles int
|
|
db ethdb.Database
|
|
}
|
|
|
|
// ShardDBConfig specifies configuration options for the db service.
|
|
type ShardDBConfig struct {
|
|
DataDir string
|
|
Name string
|
|
InMemory bool
|
|
}
|
|
|
|
// NewShardDB initializes a shardDB.
|
|
func NewShardDB(config *ShardDBConfig) (*ShardDB, error) {
|
|
// Uses default cache and handles values.
|
|
// TODO: allow these arguments to be set based on cli context.
|
|
shardDB := &ShardDB{
|
|
name: config.Name,
|
|
dataDir: config.DataDir,
|
|
}
|
|
if config.InMemory {
|
|
shardDB.inmemory = true
|
|
shardDB.db = sharedDB.NewKVStore()
|
|
} else {
|
|
shardDB.inmemory = false
|
|
shardDB.cache = 16
|
|
shardDB.handles = 16
|
|
}
|
|
return shardDB, nil
|
|
}
|
|
|
|
// Start the shard DB service.
|
|
func (s *ShardDB) Start() {
|
|
log.Info("Starting shardDB service")
|
|
if !s.inmemory {
|
|
db, err := ethdb.NewLDBDatabase(filepath.Join(s.dataDir, s.name), s.cache, s.handles)
|
|
if err != nil {
|
|
log.Error(fmt.Sprintf("Could not start shard DB: %v", err))
|
|
return
|
|
}
|
|
s.db = db
|
|
}
|
|
}
|
|
|
|
// Stop the shard DB service gracefully.
|
|
func (s *ShardDB) Stop() error {
|
|
log.Info("Stopping shardDB service")
|
|
s.db.Close()
|
|
return nil
|
|
}
|
|
|
|
// DB returns the attached ethdb instance.
|
|
func (s *ShardDB) DB() ethdb.Database {
|
|
return s.db
|
|
}
|