mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
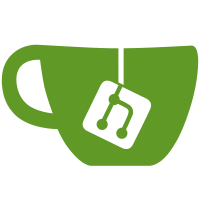
* double_tree_changes * protoarray changes * beacon-chain changes * spec tests and debug rpc fixes * more conflicts * more conflicts * Terence's review Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
51 lines
1.5 KiB
Go
51 lines
1.5 KiB
Go
package blockchain
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"testing"
|
|
|
|
testDB "github.com/prysmaticlabs/prysm/beacon-chain/db/testing"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/forkchoice/protoarray"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/state"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/state/stategen"
|
|
)
|
|
|
|
func testServiceOptsWithDB(t *testing.T) []Option {
|
|
beaconDB := testDB.SetupDB(t)
|
|
fcs := protoarray.New()
|
|
return []Option{
|
|
WithDatabase(beaconDB),
|
|
WithStateGen(stategen.New(beaconDB)),
|
|
WithForkChoiceStore(fcs),
|
|
}
|
|
}
|
|
|
|
// WARNING: only use these opts when you are certain there are no db calls
|
|
// in your code path. this is a lightweight way to satisfy the stategen/beacondb
|
|
// initialization requirements w/o the overhead of db init.
|
|
func testServiceOptsNoDB() []Option {
|
|
return []Option{
|
|
withStateBalanceCache(satisfactoryStateBalanceCache()),
|
|
}
|
|
}
|
|
|
|
type mockStateByRooter struct {
|
|
state state.BeaconState
|
|
err error
|
|
}
|
|
|
|
var _ stateByRooter = &mockStateByRooter{}
|
|
|
|
func (m mockStateByRooter) StateByRoot(_ context.Context, _ [32]byte) (state.BeaconState, error) {
|
|
return m.state, m.err
|
|
}
|
|
|
|
// returns an instance of the state balance cache that can be used
|
|
// to satisfy the requirement for one in NewService, but which will
|
|
// always return an error if used.
|
|
func satisfactoryStateBalanceCache() *stateBalanceCache {
|
|
err := errors.New("satisfactoryStateBalanceCache doesn't perform real caching")
|
|
return &stateBalanceCache{stateGen: mockStateByRooter{err: err}}
|
|
}
|