mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
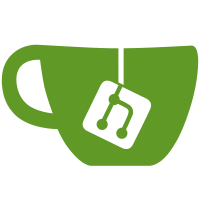
* add main.go * interop readme * proper visibility * standardize and abstract into simpler funcs * formatting * no os pkg * add test * no panics anywhere, properly and nicely handle errors * proper comments * fix broken test * readme * comment * recommend ssz * install * tool now works * README * build * readme * 64 validators * rem print * register the no powchain flag * work on mock eth1 start * common interface * getting closer with the interface defs * only two uses of powchain * remove powchain dependency * remove powchain dependency * common powchain interface * proper comment in case of flag * proper args into rpc services * rename fields * pass in mock flag into RPC * conforms to iface * use client instead of block fetcher iface * broken tests * block fetcher * finalized * resolved broken build * fix build * comment * fix tests * tests pass * resolved confs * took them out * rename into smaller interfaces * resolve some confs * ensure tests pass * properly utilize mock instead of localized mock * res lint * lint * finish test for mock eth1data * run gazelle * include flag again * fix broken build * disable powchain * dont dial eth1 nodes * reenable pow * use smaller interfaces, standardize naming * abstract mock into its own package * faulty mock lint * fix stutter in lint * rpc tests all passing * use mocks for operations * no more mocks in the entire rpc package * no mock * viz * testonly
91 lines
2.4 KiB
Go
91 lines
2.4 KiB
Go
package blockchain
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/gogo/protobuf/proto"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
)
|
|
|
|
// ChainInfoFetcher defines a common interface for methods in blockchain service which
|
|
// directly retrieves chain info related data.
|
|
type ChainInfoFetcher interface {
|
|
HeadFetcher
|
|
CanonicalRootFetcher
|
|
FinalizationFetcher
|
|
}
|
|
|
|
// HeadFetcher defines a common interface for methods in blockchain service which
|
|
// directly retrieves head related data.
|
|
type HeadFetcher interface {
|
|
HeadSlot() uint64
|
|
HeadRoot() []byte
|
|
HeadBlock() *ethpb.BeaconBlock
|
|
HeadState() *pb.BeaconState
|
|
}
|
|
|
|
// CanonicalRootFetcher defines a common interface for methods in blockchain service which
|
|
// directly retrieves canonical roots related data.
|
|
type CanonicalRootFetcher interface {
|
|
CanonicalRoot(slot uint64) []byte
|
|
}
|
|
|
|
// FinalizationFetcher defines a common interface for methods in blockchain service which
|
|
// directly retrieves finalization related data.
|
|
type FinalizationFetcher interface {
|
|
FinalizedCheckpt() *ethpb.Checkpoint
|
|
}
|
|
|
|
// FinalizedCheckpt returns the latest finalized checkpoint tracked in fork choice service.
|
|
func (s *Service) FinalizedCheckpt() *ethpb.Checkpoint {
|
|
cp := s.forkChoiceStore.FinalizedCheckpt()
|
|
if cp != nil {
|
|
return cp
|
|
}
|
|
|
|
return ðpb.Checkpoint{Root: params.BeaconConfig().ZeroHash[:]}
|
|
}
|
|
|
|
// HeadSlot returns the slot of the head of the chain.
|
|
func (s *Service) HeadSlot() uint64 {
|
|
return s.headSlot
|
|
}
|
|
|
|
// HeadRoot returns the root of the head of the chain.
|
|
func (s *Service) HeadRoot() []byte {
|
|
s.canonicalRootsLock.RLock()
|
|
defer s.canonicalRootsLock.RUnlock()
|
|
|
|
root := s.canonicalRoots[s.headSlot]
|
|
if len(root) != 0 {
|
|
return root
|
|
}
|
|
|
|
return params.BeaconConfig().ZeroHash[:]
|
|
}
|
|
|
|
// HeadBlock returns the head block of the chain.
|
|
func (s *Service) HeadBlock() *ethpb.BeaconBlock {
|
|
return proto.Clone(s.headBlock).(*ethpb.BeaconBlock)
|
|
}
|
|
|
|
// HeadState returns the head state of the chain.
|
|
func (s *Service) HeadState() *pb.BeaconState {
|
|
return proto.Clone(s.headState).(*pb.BeaconState)
|
|
}
|
|
|
|
// CanonicalRoot returns the canonical root of a given slot.
|
|
func (s *Service) CanonicalRoot(slot uint64) []byte {
|
|
s.canonicalRootsLock.RLock()
|
|
defer s.canonicalRootsLock.RUnlock()
|
|
|
|
return s.canonicalRoots[slot]
|
|
}
|
|
|
|
// GenesisTime returns the genesis time of beacon chain.
|
|
func (s *Service) GenesisTime() time.Time {
|
|
return s.genesisTime
|
|
}
|