mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
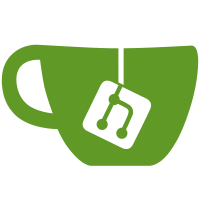
* add main.go * interop readme * proper visibility * standardize and abstract into simpler funcs * formatting * no os pkg * add test * no panics anywhere, properly and nicely handle errors * proper comments * fix broken test * readme * comment * recommend ssz * install * tool now works * README * build * readme * 64 validators * rem print * register the no powchain flag * work on mock eth1 start * common interface * getting closer with the interface defs * only two uses of powchain * remove powchain dependency * remove powchain dependency * common powchain interface * proper comment in case of flag * proper args into rpc services * rename fields * pass in mock flag into RPC * conforms to iface * use client instead of block fetcher iface * broken tests * block fetcher * finalized * resolved broken build * fix build * comment * fix tests * tests pass * resolved confs * took them out * rename into smaller interfaces * resolve some confs * ensure tests pass * properly utilize mock instead of localized mock * res lint * lint * finish test for mock eth1data * run gazelle * include flag again * fix broken build * disable powchain * dont dial eth1 nodes * reenable pow * use smaller interfaces, standardize naming * abstract mock into its own package * faulty mock lint * fix stutter in lint * rpc tests all passing * use mocks for operations * no more mocks in the entire rpc package * no mock * viz * testonly
90 lines
2.3 KiB
Go
90 lines
2.3 KiB
Go
package blockchain
|
|
|
|
import (
|
|
"bytes"
|
|
"context"
|
|
"reflect"
|
|
"testing"
|
|
|
|
testDB "github.com/prysmaticlabs/prysm/beacon-chain/db/testing"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
)
|
|
|
|
// Ensure Service implements chain info interface.
|
|
var _ = ChainInfoFetcher(&Service{})
|
|
|
|
func TestFinalizedCheckpt_Nil(t *testing.T) {
|
|
c := setupBeaconChain(t, nil)
|
|
if !bytes.Equal(c.FinalizedCheckpt().Root, params.BeaconConfig().ZeroHash[:]) {
|
|
t.Error("Incorrect pre chain start value")
|
|
}
|
|
}
|
|
|
|
func TestHeadRoot_Nil(t *testing.T) {
|
|
c := setupBeaconChain(t, nil)
|
|
if !bytes.Equal(c.HeadRoot(), params.BeaconConfig().ZeroHash[:]) {
|
|
t.Error("Incorrect pre chain start value")
|
|
}
|
|
}
|
|
|
|
func TestFinalizedCheckpt_CanRetrieve(t *testing.T) {
|
|
db := testDB.SetupDB(t)
|
|
defer testDB.TeardownDB(t, db)
|
|
ctx := context.Background()
|
|
|
|
c := setupBeaconChain(t, db)
|
|
|
|
if err := c.forkChoiceStore.GenesisStore(ctx, ðpb.Checkpoint{}, ðpb.Checkpoint{}); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
if c.FinalizedCheckpt().Epoch != 0 {
|
|
t.Errorf("Finalized epoch at genesis should be 0, got: %d", c.FinalizedCheckpt().Epoch)
|
|
}
|
|
}
|
|
|
|
func TestHeadSlot_CanRetrieve(t *testing.T) {
|
|
c := &Service{}
|
|
c.headSlot = 100
|
|
if c.HeadSlot() != 100 {
|
|
t.Errorf("Wanted head slot: %d, got: %d", 100, c.HeadSlot())
|
|
}
|
|
}
|
|
|
|
func TestHeadRoot_CanRetrieve(t *testing.T) {
|
|
c := &Service{canonicalRoots: make(map[uint64][]byte)}
|
|
c.headSlot = 100
|
|
c.canonicalRoots[c.headSlot] = []byte{'A'}
|
|
if !bytes.Equal([]byte{'A'}, c.HeadRoot()) {
|
|
t.Errorf("Wanted head root: %v, got: %d", []byte{'A'}, c.HeadRoot())
|
|
}
|
|
}
|
|
|
|
func TestHeadBlock_CanRetrieve(t *testing.T) {
|
|
b := ðpb.BeaconBlock{Slot: 1}
|
|
c := &Service{headBlock: b}
|
|
if !reflect.DeepEqual(b, c.HeadBlock()) {
|
|
t.Error("incorrect head block received")
|
|
}
|
|
}
|
|
|
|
func TestHeadState_CanRetrieve(t *testing.T) {
|
|
s := &pb.BeaconState{Slot: 2}
|
|
c := &Service{headState: s}
|
|
if !reflect.DeepEqual(s, c.HeadState()) {
|
|
t.Error("incorrect head state received")
|
|
}
|
|
}
|
|
|
|
func TestCanonicalRoot_CanRetrieve(t *testing.T) {
|
|
c := &Service{canonicalRoots: make(map[uint64][]byte)}
|
|
slot := uint64(123)
|
|
r := []byte{'B'}
|
|
c.canonicalRoots[slot] = r
|
|
if !bytes.Equal(r, c.CanonicalRoot(slot)) {
|
|
t.Errorf("Wanted head root: %v, got: %d", []byte{'A'}, c.CanonicalRoot(slot))
|
|
}
|
|
}
|