mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
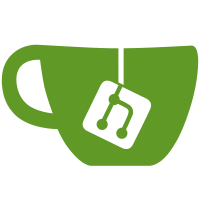
* combines func params * update leftovers Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
31 lines
859 B
Go
31 lines
859 B
Go
package depositcontract
|
|
|
|
import (
|
|
"bytes"
|
|
|
|
"github.com/ethereum/go-ethereum/accounts/abi"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// UnpackDepositLogData unpacks the data from a deposit log using the ABI decoder.
|
|
func UnpackDepositLogData(data []byte) (pubkey, withdrawalCredentials, amount, signature, index []byte, err error) {
|
|
reader := bytes.NewReader([]byte(DepositContractABI))
|
|
contractAbi, err := abi.JSON(reader)
|
|
if err != nil {
|
|
return nil, nil, nil, nil, nil, errors.Wrap(err, "unable to generate contract abi")
|
|
}
|
|
|
|
unpackedLogs := []interface{}{
|
|
&pubkey,
|
|
&withdrawalCredentials,
|
|
&amount,
|
|
&signature,
|
|
&index,
|
|
}
|
|
if err := contractAbi.Unpack(&unpackedLogs, "DepositEvent", data); err != nil {
|
|
return nil, nil, nil, nil, nil, errors.Wrap(err, "unable to unpack logs")
|
|
}
|
|
|
|
return pubkey, withdrawalCredentials, amount, signature, index, nil
|
|
}
|