mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
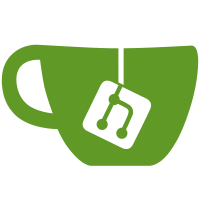
* Implemented ProcessSlashingsPrecompute * Tests for ProcessSlashingsPrecompute * Gaz * Lint * Removed comment
35 lines
1.3 KiB
Go
35 lines
1.3 KiB
Go
package precompute
|
|
|
|
import (
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/prysmaticlabs/prysm/shared/mathutil"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
)
|
|
|
|
// ProcessSlashingsPrecompute processes the slashed validators during epoch processing.
|
|
// This is an optimized version by passing in precomputed total epoch balances.
|
|
func ProcessSlashingsPrecompute(state *pb.BeaconState, p *Balance) *pb.BeaconState {
|
|
currentEpoch := helpers.CurrentEpoch(state)
|
|
exitLength := params.BeaconConfig().EpochsPerSlashingsVector
|
|
|
|
// Compute the sum of state slashings
|
|
totalSlashing := uint64(0)
|
|
for _, slashing := range state.Slashings {
|
|
totalSlashing += slashing
|
|
}
|
|
|
|
// Compute slashing for each validator.
|
|
for index, validator := range state.Validators {
|
|
correctEpoch := (currentEpoch + exitLength/2) == validator.WithdrawableEpoch
|
|
if validator.Slashed && correctEpoch {
|
|
minSlashing := mathutil.Min(totalSlashing*3, p.CurrentEpoch)
|
|
increment := params.BeaconConfig().EffectiveBalanceIncrement
|
|
penaltyNumerator := validator.EffectiveBalance / increment * minSlashing
|
|
penalty := penaltyNumerator / p.CurrentEpoch * increment
|
|
state = helpers.DecreaseBalance(state, uint64(index), penalty)
|
|
}
|
|
}
|
|
return state
|
|
}
|