mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-27 21:57:16 +00:00
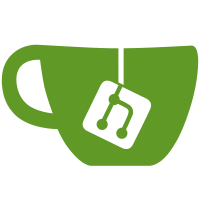
* first version * cli context * fix service * starting change to ccache * ristretto cache * added test * test on evict * remove evict test * test onevict * comment for exported flag * update all span maps on load * fix setup db * span cache added to help flags * start save cache on exit * save cache to db before close * comment fix * fix flags * setup db new * data update from archive node * gaz * slashing detection on old attestations * un-export * rename * nishant feedback * workspace cr * lint fix * fix calls * start db * fix test * Update slasher/db/db.go Co-Authored-By: Nishant Das <nishdas93@gmail.com> * add flag * fix fail to start beacon client * mock beacon service * fix imports * gaz * goimports * add clear db flag * print finalized epoch * better msg * Update slasher/db/attester_slashings.go Co-Authored-By: Raul Jordan <raul@prysmaticlabs.com> * raul feedback * raul feedback * raul feedback * raul feedback * raul feedback * add detection in runtime * fix tests * raul feedbacks * raul feedback * raul feedback * goimports * Update beacon-chain/blockchain/process_attestation_helpers.go * Update beacon-chain/blockchain/receive_block.go * Update beacon-chain/core/blocks/block_operations_test.go * Update beacon-chain/core/blocks/block_operations.go * Update beacon-chain/core/epoch/epoch_processing.go * Update beacon-chain/sync/validate_aggregate_proof_test.go * Update shared/testutil/block.go * Update slasher/service/data_update.go * Update tools/blocktree/main.go * Update slasher/service/service.go * Update beacon-chain/core/epoch/precompute/attestation_test.go * Update beacon-chain/core/helpers/committee_test.go * Update beacon-chain/core/state/transition_test.go * Update beacon-chain/rpc/aggregator/server_test.go * Update beacon-chain/sync/validate_aggregate_proof.go * Update beacon-chain/rpc/validator/proposer_test.go * Update beacon-chain/blockchain/forkchoice/process_attestation.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * Update slasher/db/indexed_attestations.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * Update slasher/service/data_update.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * terence feedback * terence feedback * goimports Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> Co-authored-by: Nishant Das <nish1993@hotmail.com> Co-authored-by: terence tsao <terence@prysmaticlabs.com>
150 lines
3.8 KiB
Go
150 lines
3.8 KiB
Go
package service
|
|
|
|
import (
|
|
"errors"
|
|
"flag"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/golang/mock/gomock"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/mock"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
"github.com/sirupsen/logrus"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
"github.com/urfave/cli"
|
|
)
|
|
|
|
func init() {
|
|
logrus.SetLevel(logrus.DebugLevel)
|
|
logrus.SetOutput(ioutil.Discard)
|
|
}
|
|
|
|
func TestLifecycle_OK(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
app := cli.NewApp()
|
|
set := flag.NewFlagSet("test", 0)
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
|
|
context := cli.NewContext(app, set, nil)
|
|
rpcService, err := NewRPCService(&Config{
|
|
Port: 7348,
|
|
}, context)
|
|
if err != nil {
|
|
t.Error("gRPC Service fail to initialize:", err)
|
|
}
|
|
client := mock.NewMockBeaconChainClient(ctrl)
|
|
rpcService.beaconClient = client
|
|
client.EXPECT().GetChainHead(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(ðpb.ChainHead{HeadSlot: 1}, nil)
|
|
client.EXPECT().StreamAttestations(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(nil, nil)
|
|
waitForStarted(rpcService, t)
|
|
rpcService.Close()
|
|
testutil.AssertLogsContain(t, hook, "Starting service")
|
|
testutil.AssertLogsContain(t, hook, "Listening on port")
|
|
testutil.AssertLogsContain(t, hook, "Stopping service")
|
|
|
|
}
|
|
|
|
func TestRPC_BadEndpoint(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
app := cli.NewApp()
|
|
set := flag.NewFlagSet("test", 0)
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
|
|
context := cli.NewContext(app, set, nil)
|
|
rpcService, err := NewRPCService(&Config{
|
|
Port: 99999999,
|
|
}, context)
|
|
if err != nil {
|
|
t.Error("gRPC Service fail to initialize:", err)
|
|
}
|
|
client := mock.NewMockBeaconChainClient(ctrl)
|
|
rpcService.beaconClient = client
|
|
client.EXPECT().GetChainHead(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(ðpb.ChainHead{HeadSlot: 1}, nil)
|
|
client.EXPECT().StreamAttestations(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(nil, nil)
|
|
testutil.AssertLogsDoNotContain(t, hook, "Could not listen to port in Start()")
|
|
testutil.AssertLogsDoNotContain(t, hook, "Could not load TLS keys")
|
|
testutil.AssertLogsDoNotContain(t, hook, "Could not serve gRPC")
|
|
|
|
waitForStarted(rpcService, t)
|
|
|
|
testutil.AssertLogsContain(t, hook, "Starting service")
|
|
testutil.AssertLogsContain(t, hook, "Could not listen to port in Start()")
|
|
|
|
rpcService.Close()
|
|
}
|
|
|
|
func TestStatus_CredentialError(t *testing.T) {
|
|
credentialErr := errors.New("credentialError")
|
|
s := &Service{credentialError: credentialErr}
|
|
|
|
if _, err := s.Status(); err != s.credentialError {
|
|
t.Errorf("Wanted: %v, got: %v", s.credentialError, err)
|
|
}
|
|
}
|
|
|
|
func TestRPC_InsecureEndpoint(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
app := cli.NewApp()
|
|
set := flag.NewFlagSet("test", 0)
|
|
context := cli.NewContext(app, set, nil)
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
|
|
rpcService, err := NewRPCService(&Config{
|
|
Port: 5555,
|
|
}, context)
|
|
if err != nil {
|
|
t.Error("gRPC Service fail to initialize:", err)
|
|
}
|
|
client := mock.NewMockBeaconChainClient(ctrl)
|
|
rpcService.beaconClient = client
|
|
client.EXPECT().GetChainHead(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(ðpb.ChainHead{HeadSlot: 1}, nil)
|
|
client.EXPECT().StreamAttestations(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(nil, nil)
|
|
waitForStarted(rpcService, t)
|
|
|
|
testutil.AssertLogsContain(t, hook, "Starting service")
|
|
testutil.AssertLogsContain(t, hook, fmt.Sprint("Listening on port"))
|
|
testutil.AssertLogsContain(t, hook, "You are using an insecure gRPC connection")
|
|
|
|
rpcService.Close()
|
|
testutil.AssertLogsContain(t, hook, "Stopping service")
|
|
}
|
|
|
|
func waitForStarted(rpcService *Service, t *testing.T) {
|
|
go rpcService.Start()
|
|
tick := time.Tick(100 * time.Millisecond)
|
|
for {
|
|
<-tick
|
|
s, err := rpcService.Status()
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if s {
|
|
break
|
|
}
|
|
}
|
|
}
|