mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
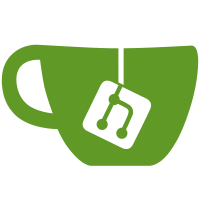
* initial implementation of command * Add second confirmation step * Merge branch 'origin-master' into voluntary-exit-command * fix variable name * added unit tests * Merge branch 'origin-master' into voluntary-exit-command * add comment about stdin * remove backup-related code from accounts_delete_test * fix comments * move filterPublicKeysFromUserInput to new account helper * remove SkipMnemonicConfirmFlag * Merge refs/heads/master into voluntary-exit-command * Merge refs/heads/master into voluntary-exit-command * Merge refs/heads/master into voluntary-exit-command * Merge refs/heads/master into voluntary-exit-command * Merge refs/heads/master into voluntary-exit-command * Merge refs/heads/master into voluntary-exit-command * Merge refs/heads/master into voluntary-exit-command * code review fixes * removed commented-out code * Merge branch 'voluntary-exit-command' into account-commands-cleanup # Conflicts: # shared/promptutil/prompt.go * Merge branch 'origin-master' into account-commands-cleanup # Conflicts: # shared/promptutil/prompt.go # validator/accounts/v2/BUILD.bazel # validator/accounts/v2/accounts_exit.go # validator/accounts/v2/accounts_exit_test.go * Merge refs/heads/master into account-commands-cleanup
47 lines
1.2 KiB
Go
47 lines
1.2 KiB
Go
package v2
|
|
|
|
import (
|
|
"encoding/hex"
|
|
"fmt"
|
|
"strings"
|
|
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/shared/bls"
|
|
"github.com/urfave/cli/v2"
|
|
)
|
|
|
|
func filterPublicKeysFromUserInput(
|
|
cliCtx *cli.Context,
|
|
publicKeysFlag *cli.StringFlag,
|
|
validatingPublicKeys [][48]byte,
|
|
selectionPrompt string,
|
|
) ([]bls.PublicKey, error) {
|
|
var filteredPubKeys []bls.PublicKey
|
|
if cliCtx.IsSet(publicKeysFlag.Name) {
|
|
pubKeyStrings := strings.Split(cliCtx.String(publicKeysFlag.Name), ",")
|
|
if len(pubKeyStrings) == 0 {
|
|
return nil, fmt.Errorf(
|
|
"could not parse %s. It must be a string of comma-separated hex strings",
|
|
publicKeysFlag.Name,
|
|
)
|
|
}
|
|
for _, str := range pubKeyStrings {
|
|
pkString := str
|
|
if strings.Contains(pkString, "0x") {
|
|
pkString = pkString[2:]
|
|
}
|
|
pubKeyBytes, err := hex.DecodeString(pkString)
|
|
if err != nil {
|
|
return nil, errors.Wrapf(err, "could not decode string %s as hex", pkString)
|
|
}
|
|
blsPublicKey, err := bls.PublicKeyFromBytes(pubKeyBytes)
|
|
if err != nil {
|
|
return nil, errors.Wrapf(err, "%#x is not a valid BLS public key", pubKeyBytes)
|
|
}
|
|
filteredPubKeys = append(filteredPubKeys, blsPublicKey)
|
|
}
|
|
return filteredPubKeys, nil
|
|
}
|
|
return selectAccounts(selectionPrompt, validatingPublicKeys)
|
|
}
|