mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-04 00:44:27 +00:00
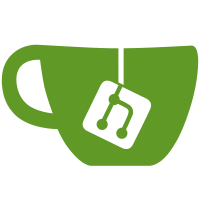
* rem slasher proto * Merge branch 'master' of github.com:prysmaticlabs/prysm * Merge branch 'master' of github.com:prysmaticlabs/prysm * Merge branch 'master' of github.com:prysmaticlabs/prysm * Merge branch 'master' of github.com:prysmaticlabs/prysm * Merge branch 'master' of github.com:prysmaticlabs/prysm * add a bit more better logging * Empty db fix * Improve logs * Fix small issues in spanner, improvements * Change costs back to 1 for now * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into cleanup-slasher * Change the cache back to 0 * Cleanup * Merge branch 'master' into cleanup-slasher * lint * added in better spans * log * rem spanner in super intensive operation * Merge branch 'master' into cleanup-slasher * add todo * Merge branch 'cleanup-slasher' of github.com:prysmaticlabs/prysm into cleanup-slasher * Merge branch 'master' into cleanup-slasher * Apply suggestions from code review * no logrus * Merge branch 'master' into cleanup-slasher * Merge branch 'cleanup-slasher' of https://github.com/prysmaticlabs/Prysm into cleanup-slasher * Remove spammy logs * Merge branch 'master' of https://github.com/prysmaticlabs/Prysm into cleanup-slasher * gaz * Rename func * Add back needed code * Add todo * Add span to cache func
48 lines
1.3 KiB
Go
48 lines
1.3 KiB
Go
package kv
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/boltdb/bolt"
|
|
"github.com/gogo/protobuf/proto"
|
|
"github.com/pkg/errors"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"go.opencensus.io/trace"
|
|
)
|
|
|
|
// ChainHead retrieves the persisted chain head from the database accordingly.
|
|
func (db *Store) ChainHead(ctx context.Context) (*ethpb.ChainHead, error) {
|
|
ctx, span := trace.StartSpan(ctx, "slasherDB.ChainHead")
|
|
defer span.End()
|
|
var res *ethpb.ChainHead
|
|
if err := db.update(func(tx *bolt.Tx) error {
|
|
bucket := tx.Bucket(chainDataBucket)
|
|
enc := bucket.Get([]byte(chainHeadKey))
|
|
if enc == nil {
|
|
return nil
|
|
}
|
|
res = ðpb.ChainHead{}
|
|
return proto.Unmarshal(enc, res)
|
|
}); err != nil {
|
|
return nil, err
|
|
}
|
|
return res, nil
|
|
}
|
|
|
|
// SaveChainHead accepts a beacon chain head object and persists it to the DB.
|
|
func (db *Store) SaveChainHead(ctx context.Context, head *ethpb.ChainHead) error {
|
|
ctx, span := trace.StartSpan(ctx, "slasherDB.SaveChainHead")
|
|
defer span.End()
|
|
enc, err := proto.Marshal(head)
|
|
if err != nil {
|
|
return errors.Wrap(err, "failed to encode chain head")
|
|
}
|
|
return db.update(func(tx *bolt.Tx) error {
|
|
bucket := tx.Bucket(chainDataBucket)
|
|
if err := bucket.Put([]byte(chainHeadKey), enc); err != nil {
|
|
return errors.Wrap(err, "failed to save chain head to db")
|
|
}
|
|
return err
|
|
})
|
|
}
|