mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-23 11:57:18 +00:00
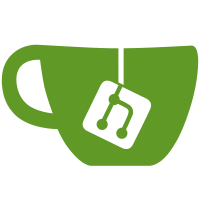
* begin state service * begin on the state trie idea * created beacon state structure * add in the full clone getter * return by value instead * add all setters * new state setters are being completed * arrays roots exposed * close to finishing all these headerssss * functionality complete * added in proto benchmark test * test for compatibility * add test for compat * comments fixed * add clone * add clone * remove underlying copies * make it immutable * integrate it into chainservice * revert * wrap up comments for package * address all comments and godocs * address all comments * clone the pending attestation properly * properly clone remaining items * tests pass fixed bug * prevent nil pointer exceptions * fixed up some bugs in the clone comparisons Co-authored-by: Nishant Das <nish1993@hotmail.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
82 lines
2.9 KiB
Go
82 lines
2.9 KiB
Go
package stateutil
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/binary"
|
|
|
|
"github.com/pkg/errors"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
)
|
|
|
|
// BlockHeaderRoot computes the HashTreeRoot Merkleization of
|
|
// a BeaconBlockHeader struct according to the eth2
|
|
// Simple Serialize specification.
|
|
func BlockHeaderRoot(header *ethpb.BeaconBlockHeader) ([32]byte, error) {
|
|
fieldRoots := make([][]byte, 4)
|
|
if header != nil {
|
|
headerSlotBuf := make([]byte, 8)
|
|
binary.LittleEndian.PutUint64(headerSlotBuf, header.Slot)
|
|
headerSlotRoot := bytesutil.ToBytes32(headerSlotBuf)
|
|
fieldRoots[0] = headerSlotRoot[:]
|
|
fieldRoots[1] = header.ParentRoot
|
|
fieldRoots[2] = header.StateRoot
|
|
fieldRoots[3] = header.BodyRoot
|
|
}
|
|
return bitwiseMerkleize(fieldRoots, uint64(len(fieldRoots)), uint64(len(fieldRoots)))
|
|
}
|
|
|
|
// Eth1Root computes the HashTreeRoot Merkleization of
|
|
// a BeaconBlockHeader struct according to the eth2
|
|
// Simple Serialize specification.
|
|
func Eth1Root(eth1Data *ethpb.Eth1Data) ([32]byte, error) {
|
|
fieldRoots := make([][]byte, 3)
|
|
for i := 0; i < len(fieldRoots); i++ {
|
|
fieldRoots[i] = make([]byte, 32)
|
|
}
|
|
if eth1Data != nil {
|
|
if len(eth1Data.DepositRoot) > 0 {
|
|
fieldRoots[0] = eth1Data.DepositRoot
|
|
}
|
|
eth1DataCountBuf := make([]byte, 8)
|
|
binary.LittleEndian.PutUint64(eth1DataCountBuf, eth1Data.DepositCount)
|
|
eth1CountRoot := bytesutil.ToBytes32(eth1DataCountBuf)
|
|
fieldRoots[1] = eth1CountRoot[:]
|
|
if len(eth1Data.BlockHash) > 0 {
|
|
fieldRoots[2] = eth1Data.BlockHash
|
|
}
|
|
}
|
|
return bitwiseMerkleize(fieldRoots, uint64(len(fieldRoots)), uint64(len(fieldRoots)))
|
|
}
|
|
|
|
// Eth1DataVotesRoot computes the HashTreeRoot Merkleization of
|
|
// a list of Eth1Data structs according to the eth2
|
|
// Simple Serialize specification.
|
|
func Eth1DataVotesRoot(eth1DataVotes []*ethpb.Eth1Data) ([32]byte, error) {
|
|
eth1VotesRoots := make([][]byte, 0)
|
|
for i := 0; i < len(eth1DataVotes); i++ {
|
|
eth1, err := Eth1Root(eth1DataVotes[i])
|
|
if err != nil {
|
|
return [32]byte{}, errors.Wrap(err, "could not compute eth1data merkleization")
|
|
}
|
|
eth1VotesRoots = append(eth1VotesRoots, eth1[:])
|
|
}
|
|
eth1Chunks, err := pack(eth1VotesRoots)
|
|
if err != nil {
|
|
return [32]byte{}, errors.Wrap(err, "could not chunk eth1 votes roots")
|
|
}
|
|
eth1VotesRootsRoot, err := bitwiseMerkleize(eth1Chunks, uint64(len(eth1Chunks)), params.BeaconConfig().SlotsPerEth1VotingPeriod)
|
|
if err != nil {
|
|
return [32]byte{}, errors.Wrap(err, "could not compute eth1data votes merkleization")
|
|
}
|
|
eth1VotesRootBuf := new(bytes.Buffer)
|
|
if err := binary.Write(eth1VotesRootBuf, binary.LittleEndian, uint64(len(eth1DataVotes))); err != nil {
|
|
return [32]byte{}, errors.Wrap(err, "could not marshal eth1data votes length")
|
|
}
|
|
// We need to mix in the length of the slice.
|
|
eth1VotesRootBufRoot := make([]byte, 32)
|
|
copy(eth1VotesRootBufRoot, eth1VotesRootBuf.Bytes())
|
|
return mixInLength(eth1VotesRootsRoot, eth1VotesRootBufRoot), nil
|
|
}
|