mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-24 20:37:17 +00:00
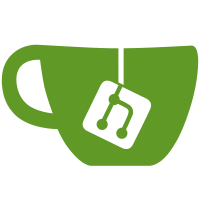
* Use new attestation protection * tests fixes * fix tests * fix comment * fix TestSetTargetData * fix tests * empty history handling * fix another test * mock domain request * fix empty handling * use far future epoch * use far future epoch * migrate data * copy byte array to resolve sigbus error * init validator protection on pre validation * Import interchange json * Import interchange json * reduce visibility * use return value * raul feedback * rename fixes * import test * checkout att v2 changes * define import method for interchange format in its own package * rename and made operations atomic * eip comment * begin amending test file * finish happy path for import tests * attempt the interchange import tests * fixed tests * happy and sad paths tested * good error messages * fix up comment with proper eip link * tests for helpers * helpers * all tests pass * proper test comment * terence feedback * validate metadata func * versioning check * begin handling duplicatesz * handle duplicate public keys with potentially different data, first pass * better handling of duplicate data * ensure duplicates are taken care of * comprehensive tests for deduplication of signed blocks * tests for deduplication * Update validator/slashing-protection/local/standard-protection-format/helpers_test.go Co-authored-by: Shay Zluf <thezluf@gmail.com> * Update validator/slashing-protection/local/standard-protection-format/helpers_test.go Co-authored-by: Shay Zluf <thezluf@gmail.com> * tests for maxuint64 and package level comment * tests passing * edge cases pass Co-authored-by: Raul Jordan <raul@prysmaticlabs.com>
166 lines
4.3 KiB
Go
166 lines
4.3 KiB
Go
package interchangeformat
|
|
|
|
import (
|
|
"math"
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func Test_uint64FromString(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
str string
|
|
want uint64
|
|
wantErr bool
|
|
}{
|
|
{
|
|
name: "Overflow uint64 gets MaxUint64",
|
|
str: "29348902839048290384902839048290384902938748278934789273984728934789273894798273498",
|
|
want: math.MaxUint64,
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "Max Uint64 works",
|
|
str: "18446744073709551615",
|
|
want: math.MaxUint64,
|
|
wantErr: false,
|
|
},
|
|
{
|
|
name: "Negative number fails",
|
|
str: "-3",
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "Junk fails",
|
|
str: "alksjdkjasd",
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "0 works",
|
|
str: "0",
|
|
want: 0,
|
|
},
|
|
{
|
|
name: "Normal uint64 works",
|
|
str: "23980",
|
|
want: 23980,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
got, err := uint64FromString(tt.str)
|
|
if (err != nil) != tt.wantErr {
|
|
t.Errorf("uint64FromString() error = %v, wantErr %v", err, tt.wantErr)
|
|
return
|
|
}
|
|
if got != tt.want {
|
|
t.Errorf("uint64FromString() got = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func Test_pubKeyFromHex(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
str string
|
|
want [48]byte
|
|
wantErr bool
|
|
}{
|
|
{
|
|
name: "Empty value fails due to wrong length",
|
|
str: "",
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "Junk fails",
|
|
str: "alksjdkjasd",
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "Empty value with 0x prefix fails due to wrong length",
|
|
str: "0x",
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "Works with 0x prefix and good public key",
|
|
str: "0xb845089a1457f811bfc000588fbb4e713669be8ce060ea6be3c6ece09afc3794106c91ca73acda5e5457122d58723bed",
|
|
want: [48]byte{184, 69, 8, 154, 20, 87, 248, 17, 191, 192, 0, 88, 143, 187, 78, 113, 54, 105, 190, 140, 224, 96, 234, 107, 227, 198, 236, 224, 154, 252, 55, 148, 16, 108, 145, 202, 115, 172, 218, 94, 84, 87, 18, 45, 88, 114, 59, 237},
|
|
},
|
|
{
|
|
name: "Works without 0x prefix and good public key",
|
|
str: "b845089a1457f811bfc000588fbb4e713669be8ce060ea6be3c6ece09afc3794106c91ca73acda5e5457122d58723bed",
|
|
want: [48]byte{184, 69, 8, 154, 20, 87, 248, 17, 191, 192, 0, 88, 143, 187, 78, 113, 54, 105, 190, 140, 224, 96, 234, 107, 227, 198, 236, 224, 154, 252, 55, 148, 16, 108, 145, 202, 115, 172, 218, 94, 84, 87, 18, 45, 88, 114, 59, 237},
|
|
},
|
|
{
|
|
name: "0x prefix and wrong length public key fails",
|
|
str: "0xb845089a1457f811bfc000588fbb4e713669be8",
|
|
wantErr: true,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
got, err := pubKeyFromHex(tt.str)
|
|
if (err != nil) != tt.wantErr {
|
|
t.Errorf("pubKeyFromHex() error = %v, wantErr %v", err, tt.wantErr)
|
|
return
|
|
}
|
|
if !reflect.DeepEqual(got, tt.want) {
|
|
t.Errorf("pubKeyFromHex() got = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func Test_rootFromHex(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
str string
|
|
want [32]byte
|
|
wantErr bool
|
|
}{
|
|
{
|
|
name: "Empty value fails due to wrong length",
|
|
str: "",
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "Junk fails",
|
|
str: "alksjdkjasd",
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "Empty value with 0x prefix fails due to wrong length",
|
|
str: "0x",
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "Works with 0x prefix and good root",
|
|
str: "0x4ff6f743a43f3b4f95350831aeaf0a122a1a392922c45d804280284a69eb850b",
|
|
want: [32]byte{79, 246, 247, 67, 164, 63, 59, 79, 149, 53, 8, 49, 174, 175, 10, 18, 42, 26, 57, 41, 34, 196, 93, 128, 66, 128, 40, 74, 105, 235, 133, 11},
|
|
},
|
|
{
|
|
name: "Works without 0x prefix and good root",
|
|
str: "4ff6f743a43f3b4f95350831aeaf0a122a1a392922c45d804280284a69eb850b",
|
|
want: [32]byte{79, 246, 247, 67, 164, 63, 59, 79, 149, 53, 8, 49, 174, 175, 10, 18, 42, 26, 57, 41, 34, 196, 93, 128, 66, 128, 40, 74, 105, 235, 133, 11},
|
|
},
|
|
{
|
|
name: "0x prefix and wrong length root fails",
|
|
str: "0xb845089a14",
|
|
wantErr: true,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
got, err := rootFromHex(tt.str)
|
|
if (err != nil) != tt.wantErr {
|
|
t.Errorf("rootFromHex() error = %v, wantErr %v", err, tt.wantErr)
|
|
return
|
|
}
|
|
if !reflect.DeepEqual(got, tt.want) {
|
|
t.Errorf("rootFromHex() got = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|