mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
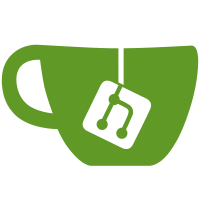
* first version * cli context * fix service * starting change to ccache * ristretto cache * added test * test on evict * remove evict test * test onevict * comment for exported flag * update all span maps on load * fix setup db * span cache added to help flags * start save cache on exit * save cache to db before close * comment fix * fix flags * setup db new * data update from archive node * gaz * slashing detection on old attestations * un-export * rename * nishant feedback * workspace cr * lint fix * fix calls * start db * fix test * Update slasher/db/db.go Co-Authored-By: Nishant Das <nishdas93@gmail.com> * add flag * fix fail to start beacon client * mock beacon service * fix imports * gaz * goimports * add clear db flag * print finalized epoch * better msg * Update slasher/db/attester_slashings.go Co-Authored-By: Raul Jordan <raul@prysmaticlabs.com> * raul feedback * raul feedback * raul feedback * raul feedback * raul feedback * add detection in runtime * fix tests * raul feedbacks * raul feedback * raul feedback * goimports * Update beacon-chain/blockchain/process_attestation_helpers.go * Update beacon-chain/blockchain/receive_block.go * Update beacon-chain/core/blocks/block_operations_test.go * Update beacon-chain/core/blocks/block_operations.go * Update beacon-chain/core/epoch/epoch_processing.go * Update beacon-chain/sync/validate_aggregate_proof_test.go * Update shared/testutil/block.go * Update slasher/service/data_update.go * Update tools/blocktree/main.go * Update slasher/service/service.go * Update beacon-chain/core/epoch/precompute/attestation_test.go * Update beacon-chain/core/helpers/committee_test.go * Update beacon-chain/core/state/transition_test.go * Update beacon-chain/rpc/aggregator/server_test.go * Update beacon-chain/sync/validate_aggregate_proof.go * Update beacon-chain/rpc/validator/proposer_test.go * Update beacon-chain/blockchain/forkchoice/process_attestation.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * Update slasher/db/indexed_attestations.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * Update slasher/service/data_update.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * terence feedback * terence feedback * goimports Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> Co-authored-by: Nishant Das <nish1993@hotmail.com> Co-authored-by: terence tsao <terence@prysmaticlabs.com>
462 lines
13 KiB
Go
462 lines
13 KiB
Go
package testutil
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"log"
|
|
"math"
|
|
"math/rand"
|
|
|
|
"github.com/golang/protobuf/proto"
|
|
"github.com/pkg/errors"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/go-bitfield"
|
|
"github.com/prysmaticlabs/go-ssz"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/helpers"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/core/state"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/prysmaticlabs/prysm/shared/attestationutil"
|
|
"github.com/prysmaticlabs/prysm/shared/bls"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/stateutil"
|
|
)
|
|
|
|
// BlockGenConfig is used to define the requested conditions
|
|
// for block generation.
|
|
type BlockGenConfig struct {
|
|
NumProposerSlashings uint64
|
|
NumAttesterSlashings uint64
|
|
NumAttestations uint64
|
|
NumDeposits uint64
|
|
NumVoluntaryExits uint64
|
|
}
|
|
|
|
// DefaultBlockGenConfig returns the block config that utilizes the
|
|
// current params in the beacon config.
|
|
func DefaultBlockGenConfig() *BlockGenConfig {
|
|
return &BlockGenConfig{
|
|
NumProposerSlashings: 0,
|
|
NumAttesterSlashings: 0,
|
|
NumAttestations: 1,
|
|
NumDeposits: 0,
|
|
NumVoluntaryExits: 0,
|
|
}
|
|
}
|
|
|
|
// GenerateFullBlock generates a fully valid block with the requested parameters.
|
|
// Use BlockGenConfig to declare the conditions you would like the block generated under.
|
|
func GenerateFullBlock(
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
conf *BlockGenConfig,
|
|
slot uint64,
|
|
) (*ethpb.SignedBeaconBlock, error) {
|
|
currentSlot := bState.Slot
|
|
if currentSlot > slot {
|
|
return nil, fmt.Errorf("current slot in state is larger than given slot. %d > %d", currentSlot, slot)
|
|
}
|
|
|
|
if conf == nil {
|
|
conf = &BlockGenConfig{}
|
|
}
|
|
|
|
var err error
|
|
pSlashings := []*ethpb.ProposerSlashing{}
|
|
numToGen := conf.NumProposerSlashings
|
|
if numToGen > 0 {
|
|
pSlashings, err = generateProposerSlashings(bState, privs, numToGen)
|
|
if err != nil {
|
|
return nil, errors.Wrapf(err, "failed generating %d proposer slashings:", numToGen)
|
|
}
|
|
}
|
|
|
|
numToGen = conf.NumAttesterSlashings
|
|
aSlashings := []*ethpb.AttesterSlashing{}
|
|
if numToGen > 0 {
|
|
aSlashings, err = generateAttesterSlashings(bState, privs, numToGen)
|
|
if err != nil {
|
|
return nil, errors.Wrapf(err, "failed generating %d attester slashings:", numToGen)
|
|
}
|
|
}
|
|
|
|
numToGen = conf.NumAttestations
|
|
atts := []*ethpb.Attestation{}
|
|
if numToGen > 0 {
|
|
atts, err = GenerateAttestations(bState, privs, numToGen, slot)
|
|
if err != nil {
|
|
return nil, errors.Wrapf(err, "failed generating %d attestations:", numToGen)
|
|
}
|
|
}
|
|
|
|
numToGen = conf.NumDeposits
|
|
newDeposits, eth1Data := []*ethpb.Deposit{}, bState.Eth1Data
|
|
if numToGen > 0 {
|
|
newDeposits, eth1Data, err = generateDepositsAndEth1Data(bState, numToGen)
|
|
if err != nil {
|
|
return nil, errors.Wrapf(err, "failed generating %d deposits:", numToGen)
|
|
}
|
|
}
|
|
|
|
numToGen = conf.NumVoluntaryExits
|
|
exits := []*ethpb.SignedVoluntaryExit{}
|
|
if numToGen > 0 {
|
|
exits, err = generateVoluntaryExits(bState, privs, numToGen)
|
|
if err != nil {
|
|
return nil, errors.Wrapf(err, "failed generating %d attester slashings:", numToGen)
|
|
}
|
|
}
|
|
|
|
newHeader := proto.Clone(bState.LatestBlockHeader).(*ethpb.BeaconBlockHeader)
|
|
prevStateRoot, err := stateutil.HashTreeRootState(bState)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
newHeader.StateRoot = prevStateRoot[:]
|
|
parentRoot, err := ssz.HashTreeRoot(newHeader)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if slot == currentSlot {
|
|
slot = currentSlot + 1
|
|
}
|
|
|
|
// Temporarily incrementing the beacon state slot here since BeaconProposerIndex is a
|
|
// function deterministic on beacon state slot.
|
|
bState.Slot = slot
|
|
reveal, err := RandaoReveal(bState, helpers.CurrentEpoch(bState), privs)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
block := ðpb.BeaconBlock{
|
|
Slot: slot,
|
|
ParentRoot: parentRoot[:],
|
|
Body: ðpb.BeaconBlockBody{
|
|
Eth1Data: eth1Data,
|
|
RandaoReveal: reveal,
|
|
ProposerSlashings: pSlashings,
|
|
AttesterSlashings: aSlashings,
|
|
Attestations: atts,
|
|
VoluntaryExits: exits,
|
|
Deposits: newDeposits,
|
|
},
|
|
}
|
|
bState.Slot = currentSlot
|
|
|
|
signature, err := BlockSignature(bState, block, privs)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return ðpb.SignedBeaconBlock{Block: block, Signature: signature.Marshal()}, nil
|
|
}
|
|
|
|
func generateProposerSlashings(
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
numSlashings uint64,
|
|
) ([]*ethpb.ProposerSlashing, error) {
|
|
currentEpoch := helpers.CurrentEpoch(bState)
|
|
|
|
proposerSlashings := make([]*ethpb.ProposerSlashing, numSlashings)
|
|
for i := uint64(0); i < numSlashings; i++ {
|
|
proposerIndex, err := randValIndex(bState)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
header1 := ðpb.SignedBeaconBlockHeader{
|
|
Header: ðpb.BeaconBlockHeader{
|
|
Slot: bState.Slot,
|
|
BodyRoot: []byte{0, 1, 0},
|
|
},
|
|
}
|
|
root, err := ssz.HashTreeRoot(header1.Header)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
domain := helpers.Domain(bState.Fork, currentEpoch, params.BeaconConfig().DomainBeaconProposer)
|
|
header1.Signature = privs[proposerIndex].Sign(root[:], domain).Marshal()
|
|
|
|
header2 := ðpb.SignedBeaconBlockHeader{
|
|
Header: ðpb.BeaconBlockHeader{
|
|
Slot: bState.Slot,
|
|
BodyRoot: []byte{0, 2, 0},
|
|
},
|
|
}
|
|
root, err = ssz.HashTreeRoot(header2.Header)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
header2.Signature = privs[proposerIndex].Sign(root[:], domain).Marshal()
|
|
|
|
slashing := ðpb.ProposerSlashing{
|
|
ProposerIndex: proposerIndex,
|
|
Header_1: header1,
|
|
Header_2: header2,
|
|
}
|
|
proposerSlashings[i] = slashing
|
|
}
|
|
return proposerSlashings, nil
|
|
}
|
|
|
|
func generateAttesterSlashings(
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
numSlashings uint64,
|
|
) ([]*ethpb.AttesterSlashing, error) {
|
|
currentEpoch := helpers.CurrentEpoch(bState)
|
|
attesterSlashings := make([]*ethpb.AttesterSlashing, numSlashings)
|
|
for i := uint64(0); i < numSlashings; i++ {
|
|
committeeIndex := rand.Uint64() % params.BeaconConfig().MaxCommitteesPerSlot
|
|
committee, err := helpers.BeaconCommitteeFromState(bState, bState.Slot, committeeIndex)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
committeeSize := uint64(len(committee))
|
|
randIndex := rand.Uint64() % uint64(len(committee))
|
|
valIndex := committee[randIndex]
|
|
|
|
aggregationBits := bitfield.NewBitlist(committeeSize)
|
|
aggregationBits.SetBitAt(randIndex, true)
|
|
att1 := ðpb.Attestation{
|
|
Data: ðpb.AttestationData{
|
|
Slot: bState.Slot,
|
|
CommitteeIndex: committeeIndex,
|
|
Target: ðpb.Checkpoint{
|
|
Epoch: currentEpoch,
|
|
Root: params.BeaconConfig().ZeroHash[:],
|
|
},
|
|
Source: ðpb.Checkpoint{
|
|
Epoch: currentEpoch + 1,
|
|
Root: params.BeaconConfig().ZeroHash[:],
|
|
},
|
|
},
|
|
AggregationBits: aggregationBits,
|
|
}
|
|
dataRoot, err := ssz.HashTreeRoot(att1.Data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
domain := helpers.Domain(bState.Fork, i, params.BeaconConfig().DomainBeaconAttester)
|
|
sig := privs[valIndex].Sign(dataRoot[:], domain)
|
|
att1.Signature = bls.AggregateSignatures([]*bls.Signature{sig}).Marshal()
|
|
|
|
att2 := ðpb.Attestation{
|
|
Data: ðpb.AttestationData{
|
|
Slot: bState.Slot,
|
|
CommitteeIndex: committeeIndex,
|
|
Target: ðpb.Checkpoint{
|
|
Epoch: currentEpoch,
|
|
Root: params.BeaconConfig().ZeroHash[:],
|
|
},
|
|
Source: ðpb.Checkpoint{
|
|
Epoch: currentEpoch,
|
|
Root: params.BeaconConfig().ZeroHash[:],
|
|
},
|
|
},
|
|
AggregationBits: aggregationBits,
|
|
}
|
|
dataRoot, err = ssz.HashTreeRoot(att2.Data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
sig = privs[valIndex].Sign(dataRoot[:], domain)
|
|
att2.Signature = bls.AggregateSignatures([]*bls.Signature{sig}).Marshal()
|
|
|
|
indexedAtt1, err := attestationutil.ConvertToIndexed(context.Background(), att1, committee)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
indexedAtt2, err := attestationutil.ConvertToIndexed(context.Background(), att2, committee)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
slashing := ðpb.AttesterSlashing{
|
|
Attestation_1: indexedAtt1,
|
|
Attestation_2: indexedAtt2,
|
|
}
|
|
attesterSlashings[i] = slashing
|
|
}
|
|
return attesterSlashings, nil
|
|
}
|
|
|
|
// GenerateAttestations creates attestations that are entirely valid, for all
|
|
// the committees of the current state slot. This function expects attestations
|
|
// requested to be cleanly divisible by committees per slot. If there is 1 committee
|
|
// in the slot, and numToGen is set to 4, then it will return 4 attestations
|
|
// for the same data with their aggregation bits split uniformly.
|
|
//
|
|
// If you request 4 attestations, but there are 8 committees, you will get 4 fully aggregated attestations.
|
|
func GenerateAttestations(
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
numToGen uint64,
|
|
slot uint64,
|
|
) ([]*ethpb.Attestation, error) {
|
|
currentEpoch := helpers.SlotToEpoch(slot)
|
|
attestations := []*ethpb.Attestation{}
|
|
generateHeadState := false
|
|
if slot > bState.Slot {
|
|
// Going back a slot here so there's no inclusion delay issues.
|
|
slot--
|
|
generateHeadState = true
|
|
}
|
|
|
|
var err error
|
|
targetRoot := make([]byte, 32)
|
|
headRoot := make([]byte, 32)
|
|
// Only calculate head state if its an attestation for the current slot or future slot.
|
|
if generateHeadState || slot == bState.Slot {
|
|
headState := proto.Clone(bState).(*pb.BeaconState)
|
|
headState, err := state.ProcessSlots(context.Background(), headState, slot+1)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
headRoot, err = helpers.BlockRootAtSlot(headState, slot)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
targetRoot, err = helpers.BlockRoot(headState, currentEpoch)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
} else {
|
|
headRoot, err = helpers.BlockRootAtSlot(bState, slot)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
|
|
activeValidatorCount, err := helpers.ActiveValidatorCount(bState, currentEpoch)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
committeesPerSlot := helpers.SlotCommitteeCount(activeValidatorCount)
|
|
|
|
if numToGen < committeesPerSlot {
|
|
log.Printf(
|
|
"Warning: %d attestations requested is less than %d committees in current slot, not all validators will be attesting.",
|
|
numToGen,
|
|
committeesPerSlot,
|
|
)
|
|
} else if numToGen > committeesPerSlot {
|
|
log.Printf(
|
|
"Warning: %d attestations requested are more than %d committees in current slot, attestations will not be perfectly efficient.",
|
|
numToGen,
|
|
committeesPerSlot,
|
|
)
|
|
}
|
|
|
|
attsPerCommittee := math.Max(float64(numToGen/committeesPerSlot), 1)
|
|
if math.Trunc(attsPerCommittee) != attsPerCommittee {
|
|
return nil, fmt.Errorf(
|
|
"requested attestations %d must be easily divisible by committees in slot %d, calculated %f",
|
|
numToGen,
|
|
committeesPerSlot,
|
|
attsPerCommittee,
|
|
)
|
|
}
|
|
|
|
domain := helpers.Domain(bState.Fork, currentEpoch, params.BeaconConfig().DomainBeaconAttester)
|
|
for c := uint64(0); c < committeesPerSlot && c < numToGen; c++ {
|
|
committee, err := helpers.BeaconCommitteeFromState(bState, slot, c)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
attData := ðpb.AttestationData{
|
|
Slot: slot,
|
|
CommitteeIndex: c,
|
|
BeaconBlockRoot: headRoot,
|
|
Source: bState.CurrentJustifiedCheckpoint,
|
|
Target: ðpb.Checkpoint{
|
|
Epoch: currentEpoch,
|
|
Root: targetRoot,
|
|
},
|
|
}
|
|
|
|
dataRoot, err := ssz.HashTreeRoot(attData)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
committeeSize := uint64(len(committee))
|
|
bitsPerAtt := committeeSize / uint64(attsPerCommittee)
|
|
for i := uint64(0); i < committeeSize; i += bitsPerAtt {
|
|
aggregationBits := bitfield.NewBitlist(committeeSize)
|
|
sigs := []*bls.Signature{}
|
|
for b := i; b < i+bitsPerAtt; b++ {
|
|
aggregationBits.SetBitAt(b, true)
|
|
sigs = append(sigs, privs[committee[b]].Sign(dataRoot[:], domain))
|
|
}
|
|
|
|
att := ðpb.Attestation{
|
|
Data: attData,
|
|
AggregationBits: aggregationBits,
|
|
Signature: bls.AggregateSignatures(sigs).Marshal(),
|
|
}
|
|
attestations = append(attestations, att)
|
|
}
|
|
}
|
|
return attestations, nil
|
|
}
|
|
|
|
func generateDepositsAndEth1Data(
|
|
bState *pb.BeaconState,
|
|
numDeposits uint64,
|
|
) (
|
|
[]*ethpb.Deposit,
|
|
*ethpb.Eth1Data,
|
|
error,
|
|
) {
|
|
previousDepsLen := bState.Eth1DepositIndex
|
|
currentDeposits, _, err := DeterministicDepositsAndKeys(previousDepsLen + numDeposits)
|
|
if err != nil {
|
|
return nil, nil, errors.Wrap(err, "could not get deposits")
|
|
}
|
|
eth1Data, err := DeterministicEth1Data(len(currentDeposits))
|
|
if err != nil {
|
|
return nil, nil, errors.Wrap(err, "could not get eth1data")
|
|
}
|
|
return currentDeposits[previousDepsLen:], eth1Data, nil
|
|
}
|
|
|
|
func generateVoluntaryExits(
|
|
bState *pb.BeaconState,
|
|
privs []*bls.SecretKey,
|
|
numExits uint64,
|
|
) ([]*ethpb.SignedVoluntaryExit, error) {
|
|
currentEpoch := helpers.CurrentEpoch(bState)
|
|
|
|
voluntaryExits := make([]*ethpb.SignedVoluntaryExit, numExits)
|
|
for i := 0; i < len(voluntaryExits); i++ {
|
|
valIndex, err := randValIndex(bState)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
exit := ðpb.SignedVoluntaryExit{
|
|
Exit: ðpb.VoluntaryExit{
|
|
Epoch: helpers.PrevEpoch(bState),
|
|
ValidatorIndex: valIndex,
|
|
},
|
|
}
|
|
root, err := ssz.HashTreeRoot(exit.Exit)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
domain := helpers.Domain(bState.Fork, currentEpoch, params.BeaconConfig().DomainVoluntaryExit)
|
|
exit.Signature = privs[valIndex].Sign(root[:], domain).Marshal()
|
|
voluntaryExits[i] = exit
|
|
}
|
|
return voluntaryExits, nil
|
|
}
|
|
|
|
func randValIndex(bState *pb.BeaconState) (uint64, error) {
|
|
activeCount, err := helpers.ActiveValidatorCount(bState, helpers.CurrentEpoch(bState))
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
return rand.Uint64() % activeCount, nil
|
|
}
|