mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
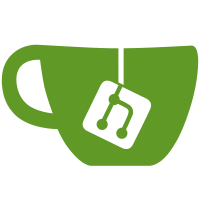
* Add a few test cases to ReceiveBlockNoPubsub with race detection, fix data race in state * Expose voluntary exits included API. More race condition fixes in state. More tests * add tests to norace as well for coverage * Merge branch 'master' of github.com:prysmaticlabs/prysm into add-test-coverage-on-receive-block * PR feedback * Fixed can't save head in DB by: 1.) Remove new state mgmt condition in SaveHeadBlockRoot 2.) Reuse state summary cache between db and service * Merge branch 'master' of github.com:prysmaticlabs/prysm into add-test-coverage-on-receive-block * passing tests * gofmt and goimports * Merge branch 'master' of github.com:prysmaticlabs/prysm into add-test-coverage-on-receive-block * lint * move import and goimport again * move import and goimport again * Merge refs/heads/master into add-test-coverage-on-receive-block * Merge refs/heads/master into add-test-coverage-on-receive-block * Add bool to selectively record events * Merge refs/heads/master into add-test-coverage-on-receive-block * Merge refs/heads/master into add-test-coverage-on-receive-block * Merge refs/heads/master into add-test-coverage-on-receive-block * Merge refs/heads/master into add-test-coverage-on-receive-block
139 lines
4.7 KiB
Go
139 lines
4.7 KiB
Go
package params
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
)
|
|
|
|
// MainnetConfig returns the configuration to be used in the main network.
|
|
func MainnetConfig() *BeaconChainConfig {
|
|
return mainnetBeaconConfig
|
|
}
|
|
|
|
// UseMainnetConfig for beacon chain services.
|
|
func UseMainnetConfig() {
|
|
beaconConfig = MainnetConfig()
|
|
}
|
|
|
|
var mainnetNetworkConfig = &NetworkConfig{
|
|
GossipMaxSize: 1 << 20, // 1 MiB
|
|
MaxChunkSize: 1 << 20, // 1 MiB
|
|
AttestationSubnetCount: 64,
|
|
AttestationPropagationSlotRange: 32,
|
|
RandomSubnetsPerValidator: 1 << 0,
|
|
EpochsPerRandomSubnetSubscription: 1 << 8,
|
|
MaxRequestBlocks: 1 << 10, // 1024
|
|
TtfbTimeout: 5 * time.Second,
|
|
RespTimeout: 10 * time.Second,
|
|
MaximumGossipClockDisparity: 500 * time.Millisecond,
|
|
ETH2Key: "eth2",
|
|
AttSubnetKey: "attnets",
|
|
ContractDeploymentBlock: 0,
|
|
DepositContractAddress: "0x", // To be updated once the mainnet contract is deployed.
|
|
BootstrapNodes: []string{},
|
|
}
|
|
|
|
var mainnetBeaconConfig = &BeaconChainConfig{
|
|
// Constants (Non-configurable)
|
|
FarFutureEpoch: 1<<64 - 1,
|
|
BaseRewardsPerEpoch: 4,
|
|
DepositContractTreeDepth: 32,
|
|
GenesisDelay: 172800, // 2 days
|
|
|
|
// Misc constant.
|
|
TargetCommitteeSize: 128,
|
|
MaxValidatorsPerCommittee: 2048,
|
|
MaxCommitteesPerSlot: 64,
|
|
MinPerEpochChurnLimit: 4,
|
|
ChurnLimitQuotient: 1 << 16,
|
|
ShuffleRoundCount: 90,
|
|
MinGenesisActiveValidatorCount: 16384,
|
|
MinGenesisTime: 0, // Zero until a proper time is decided.
|
|
TargetAggregatorsPerCommittee: 16,
|
|
HysteresisQuotient: 4,
|
|
HysteresisDownwardMultiplier: 1,
|
|
HysteresisUpwardMultiplier: 5,
|
|
|
|
// Gwei value constants.
|
|
MinDepositAmount: 1 * 1e9,
|
|
MaxEffectiveBalance: 32 * 1e9,
|
|
EjectionBalance: 16 * 1e9,
|
|
EffectiveBalanceIncrement: 1 * 1e9,
|
|
|
|
// Initial value constants.
|
|
BLSWithdrawalPrefixByte: byte(0),
|
|
ZeroHash: [32]byte{},
|
|
|
|
// Time parameter constants.
|
|
MinAttestationInclusionDelay: 1,
|
|
SecondsPerSlot: 12,
|
|
SlotsPerEpoch: 32,
|
|
MinSeedLookahead: 1,
|
|
MaxSeedLookahead: 4,
|
|
EpochsPerEth1VotingPeriod: 32,
|
|
SlotsPerHistoricalRoot: 8192,
|
|
MinValidatorWithdrawabilityDelay: 256,
|
|
ShardCommitteePeriod: 256,
|
|
MinEpochsToInactivityPenalty: 4,
|
|
Eth1FollowDistance: 1024,
|
|
SafeSlotsToUpdateJustified: 8,
|
|
SecondsPerETH1Block: 14,
|
|
|
|
// State list length constants.
|
|
EpochsPerHistoricalVector: 65536,
|
|
EpochsPerSlashingsVector: 8192,
|
|
HistoricalRootsLimit: 16777216,
|
|
ValidatorRegistryLimit: 1099511627776,
|
|
|
|
// Reward and penalty quotients constants.
|
|
BaseRewardFactor: 64,
|
|
WhistleBlowerRewardQuotient: 512,
|
|
ProposerRewardQuotient: 8,
|
|
InactivityPenaltyQuotient: 1 << 24,
|
|
MinSlashingPenaltyQuotient: 32,
|
|
|
|
// Max operations per block constants.
|
|
MaxProposerSlashings: 16,
|
|
MaxAttesterSlashings: 2,
|
|
MaxAttestations: 128,
|
|
MaxDeposits: 16,
|
|
MaxVoluntaryExits: 16,
|
|
|
|
// BLS domain values.
|
|
DomainBeaconProposer: bytesutil.ToBytes4(bytesutil.Bytes4(0)),
|
|
DomainBeaconAttester: bytesutil.ToBytes4(bytesutil.Bytes4(1)),
|
|
DomainRandao: bytesutil.ToBytes4(bytesutil.Bytes4(2)),
|
|
DomainDeposit: bytesutil.ToBytes4(bytesutil.Bytes4(3)),
|
|
DomainVoluntaryExit: bytesutil.ToBytes4(bytesutil.Bytes4(4)),
|
|
DomainSelectionProof: bytesutil.ToBytes4(bytesutil.Bytes4(5)),
|
|
DomainAggregateAndProof: bytesutil.ToBytes4(bytesutil.Bytes4(6)),
|
|
|
|
// Prysm constants.
|
|
GweiPerEth: 1000000000,
|
|
BLSSecretKeyLength: 32,
|
|
BLSPubkeyLength: 48,
|
|
BLSSignatureLength: 96,
|
|
DefaultBufferSize: 10000,
|
|
WithdrawalPrivkeyFileName: "/shardwithdrawalkey",
|
|
ValidatorPrivkeyFileName: "/validatorprivatekey",
|
|
RPCSyncCheck: 1,
|
|
EmptySignature: [96]byte{},
|
|
DefaultPageSize: 250,
|
|
MaxPeersToSync: 15,
|
|
SlotsPerArchivedPoint: 2048,
|
|
GenesisCountdownInterval: time.Minute,
|
|
|
|
// Slasher related values.
|
|
WeakSubjectivityPeriod: 54000,
|
|
PruneSlasherStoragePeriod: 10,
|
|
|
|
// Fork related values.
|
|
GenesisForkVersion: []byte{0, 0, 0, 0},
|
|
NextForkVersion: []byte{0, 0, 0, 0}, // Set to GenesisForkVersion unless there is a scheduled fork
|
|
NextForkEpoch: 1<<64 - 1, // Set to FarFutureEpoch unless there is a scheduled fork.
|
|
ForkVersionSchedule: map[uint64][]byte{
|
|
// Any further forks must be specified here by their epoch number.
|
|
},
|
|
}
|