mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
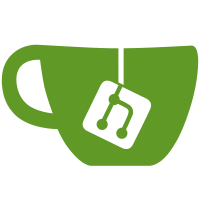
* initial changes * gaz * unexport and add in godoc * nocache * fix edge case * fix bad implementation * fix build file * add it in * terence's review * gaz * fix build * Apply suggestions from code review remove assigned ctx Co-authored-by: terence tsao <terence@prysmaticlabs.com>
56 lines
1.4 KiB
Go
56 lines
1.4 KiB
Go
package v1
|
|
|
|
import (
|
|
ethpb "github.com/prysmaticlabs/prysm/proto/prysm/v1alpha1"
|
|
)
|
|
|
|
// PreviousEpochAttestations corresponding to blocks on the beacon chain.
|
|
func (b *BeaconState) PreviousEpochAttestations() ([]*ethpb.PendingAttestation, error) {
|
|
if !b.hasInnerState() {
|
|
return nil, nil
|
|
}
|
|
if b.state.PreviousEpochAttestations == nil {
|
|
return nil, nil
|
|
}
|
|
|
|
b.lock.RLock()
|
|
defer b.lock.RUnlock()
|
|
|
|
return b.previousEpochAttestations(), nil
|
|
}
|
|
|
|
// previousEpochAttestations corresponding to blocks on the beacon chain.
|
|
// This assumes that a lock is already held on BeaconState.
|
|
func (b *BeaconState) previousEpochAttestations() []*ethpb.PendingAttestation {
|
|
if !b.hasInnerState() {
|
|
return nil
|
|
}
|
|
|
|
return ethpb.CopyPendingAttestationSlice(b.state.PreviousEpochAttestations)
|
|
}
|
|
|
|
// CurrentEpochAttestations corresponding to blocks on the beacon chain.
|
|
func (b *BeaconState) CurrentEpochAttestations() ([]*ethpb.PendingAttestation, error) {
|
|
if !b.hasInnerState() {
|
|
return nil, nil
|
|
}
|
|
if b.state.CurrentEpochAttestations == nil {
|
|
return nil, nil
|
|
}
|
|
|
|
b.lock.RLock()
|
|
defer b.lock.RUnlock()
|
|
|
|
return b.currentEpochAttestations(), nil
|
|
}
|
|
|
|
// currentEpochAttestations corresponding to blocks on the beacon chain.
|
|
// This assumes that a lock is already held on BeaconState.
|
|
func (b *BeaconState) currentEpochAttestations() []*ethpb.PendingAttestation {
|
|
if !b.hasInnerState() {
|
|
return nil
|
|
}
|
|
|
|
return ethpb.CopyPendingAttestationSlice(b.state.CurrentEpochAttestations)
|
|
}
|