mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
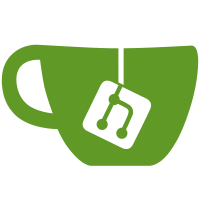
* clean up test * adding test setup * add chainstart check to sync querier * goimports * goimports * backend cleanup * adding getters * lint * moving to db * gazelle * adding more services setup * adding more changes * adding different test setups * other merge issues * imports * fixing keys * get intial sync able to be set up * new changes and gazelle * use simulated p2p * everything finally works * unexport fields * revert changes from merge * remove mock server * add documentation * gazelle * add another node * review comments * fix tests
33 lines
831 B
Go
33 lines
831 B
Go
package db
|
|
|
|
import (
|
|
"crypto/rand"
|
|
"fmt"
|
|
"math/big"
|
|
"os"
|
|
"path"
|
|
)
|
|
|
|
// SetupDB instantiates and returns a simulated backend BeaconDB instance.
|
|
func SetupDB() (*BeaconDB, error) {
|
|
randPath, err := rand.Int(rand.Reader, big.NewInt(1000000))
|
|
if err != nil {
|
|
return nil, fmt.Errorf("could not generate random file path: %v", err)
|
|
}
|
|
path := path.Join(os.TempDir(), fmt.Sprintf("/%d", randPath))
|
|
if err := os.RemoveAll(path); err != nil {
|
|
return nil, fmt.Errorf("failed to remove directory: %v", err)
|
|
}
|
|
return NewDB(path)
|
|
}
|
|
|
|
// TeardownDB cleans up a simulated backend BeaconDB instance.
|
|
func TeardownDB(db *BeaconDB) {
|
|
if err := db.Close(); err != nil {
|
|
log.Fatalf("failed to close database: %v", err)
|
|
}
|
|
if err := os.RemoveAll(db.DatabasePath); err != nil {
|
|
log.Fatalf("could not remove tmp db dir: %v", err)
|
|
}
|
|
}
|