mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
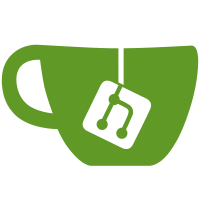
Former-commit-id: f7344b7d3fb2fa07f0fd421c5ed3721f6c7a9258 [formerly e678ab2f1681d9492c0e1b142cd06ee08a462fdb] Former-commit-id: d942da3d5cfcde921bebb149c26edd5c4ed178dd
42 lines
1.1 KiB
Go
42 lines
1.1 KiB
Go
package blockchain
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/database"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
var log = logrus.WithField("prefix", "blockchain")
|
|
|
|
// ChainService represents a service that handles the internal
|
|
// logic of managing the full PoS beacon chain.
|
|
type ChainService struct {
|
|
ctx context.Context
|
|
cancel context.CancelFunc
|
|
beaconDB *database.BeaconDB
|
|
chain *BeaconChain
|
|
}
|
|
|
|
// NewChainService instantiates a new service instance that will
|
|
// be registered into a running beacon node.
|
|
func NewChainService(ctx context.Context, beaconDB *database.BeaconDB) (*ChainService, error) {
|
|
ctx, cancel := context.WithCancel(ctx)
|
|
return &ChainService{ctx, cancel, beaconDB, nil}, nil
|
|
}
|
|
|
|
// Start a blockchain service's main event loop.
|
|
func (c *ChainService) Start() {
|
|
log.Infof("Starting blockchain service")
|
|
if _, err := NewBeaconChain(c.beaconDB.DB()); err != nil {
|
|
log.Errorf("Unable to setup blockchain: %v", err)
|
|
}
|
|
}
|
|
|
|
// Stop the blockchain service's main event loop and associated goroutines.
|
|
func (c *ChainService) Stop() error {
|
|
defer c.cancel()
|
|
log.Info("Stopping blockchain service")
|
|
return nil
|
|
}
|