mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
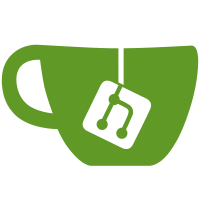
* slasher/beaconclient tests * slasher/db/kv tests * Merge branch 'master' into apply-testutils-assertions-to-slasher * fix build * slasher/detection tests * rest of the tests * misc tests * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Update slasher/db/kv/spanner_test.go Co-authored-by: Shay Zluf <thezluf@gmail.com> * Update slasher/node/node_test.go Co-authored-by: Shay Zluf <thezluf@gmail.com> * Merge refs/heads/master into apply-testutils-assertions-to-slasher * Update slasher/db/kv/spanner_test.go * Merge refs/heads/master into apply-testutils-assertions-to-slasher
86 lines
2.5 KiB
Go
86 lines
2.5 KiB
Go
package beaconclient
|
|
|
|
import (
|
|
"context"
|
|
"strconv"
|
|
"testing"
|
|
|
|
"github.com/golang/mock/gomock"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/mock"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
testDB "github.com/prysmaticlabs/prysm/slasher/db/testing"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
func TestService_RequestHistoricalAttestations(t *testing.T) {
|
|
params.SetupTestConfigCleanup(t)
|
|
hook := logTest.NewGlobal()
|
|
ctrl := gomock.NewController(t)
|
|
defer ctrl.Finish()
|
|
db := testDB.SetupSlasherDB(t, false)
|
|
client := mock.NewMockBeaconChainClient(ctrl)
|
|
|
|
bs := Service{
|
|
beaconClient: client,
|
|
slasherDB: db,
|
|
}
|
|
|
|
numAtts := 1000
|
|
wanted := make([]*ethpb.IndexedAttestation, numAtts)
|
|
for i := 0; i < numAtts; i++ {
|
|
wanted[i] = ðpb.IndexedAttestation{
|
|
AttestingIndices: []uint64{1, 2, 3},
|
|
Data: ðpb.AttestationData{
|
|
Slot: uint64(i),
|
|
Target: ðpb.Checkpoint{
|
|
Epoch: 1,
|
|
Root: make([]byte, 32),
|
|
},
|
|
},
|
|
}
|
|
}
|
|
|
|
// We override the page size in the requests to 100 so we will
|
|
// obtain 10 pages of indexed attestations from the server.
|
|
numPages := 100
|
|
perPage := numAtts / numPages
|
|
cfg := params.BeaconConfig()
|
|
cfg.DefaultPageSize = perPage
|
|
params.OverrideBeaconConfig(cfg)
|
|
|
|
// We expect there to be numPages calls to ListIndexedAttestations
|
|
// to retrieve all attestations for epoch 0.
|
|
for i := 0; i < numAtts; i += perPage {
|
|
if i+perPage >= numAtts {
|
|
client.EXPECT().ListIndexedAttestations(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(ðpb.ListIndexedAttestationsResponse{
|
|
IndexedAttestations: wanted[i:],
|
|
NextPageToken: "",
|
|
TotalSize: int32(numAtts),
|
|
}, nil)
|
|
} else {
|
|
client.EXPECT().ListIndexedAttestations(
|
|
gomock.Any(),
|
|
gomock.Any(),
|
|
).Return(ðpb.ListIndexedAttestationsResponse{
|
|
IndexedAttestations: wanted[i : i+perPage],
|
|
NextPageToken: strconv.Itoa(i + 1),
|
|
TotalSize: int32(numAtts),
|
|
}, nil)
|
|
}
|
|
}
|
|
|
|
// We request attestations for epoch 0.
|
|
res, err := bs.RequestHistoricalAttestations(context.Background(), 0)
|
|
require.NoError(t, err)
|
|
assert.DeepEqual(t, wanted, res)
|
|
require.LogsContain(t, hook, "Retrieved 100/1000 indexed attestations for epoch 0")
|
|
require.LogsContain(t, hook, "Retrieved 500/1000 indexed attestations for epoch 0")
|
|
require.LogsContain(t, hook, "Retrieved 1000/1000 indexed attestations for epoch 0")
|
|
}
|