mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
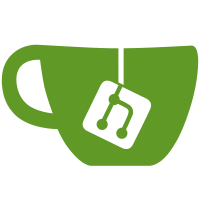
* change default wallet dir path to not be hidden * gaz + pass wallet dir * gaz + move const to flags * move to flags * move to flags * use filepath join in order to create a valid dir name * add wallet dir * return err no wallet found issues * fix up edit remote * all tests passing * fix test * create or open wallet * ivan feedback * enter password for account with pubkey * Update validator/accounts/v2/accounts_create.go Co-authored-by: Ivan Martinez <ivanthegreatdev@gmail.com> * works * preston feedback * nothing to export * fmt * test for create or open * gaz Co-authored-by: shayzluf <thezluf@gmail.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> Co-authored-by: Ivan Martinez <ivanthegreatdev@gmail.com>
80 lines
2.7 KiB
Go
80 lines
2.7 KiB
Go
package v2
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/validator/flags"
|
|
v2keymanager "github.com/prysmaticlabs/prysm/validator/keymanager/v2"
|
|
"github.com/prysmaticlabs/prysm/validator/keymanager/v2/direct"
|
|
"github.com/prysmaticlabs/prysm/validator/keymanager/v2/remote"
|
|
"github.com/urfave/cli/v2"
|
|
)
|
|
|
|
// EditWalletConfiguration for a user's on-disk wallet, being able to change
|
|
// things such as remote gRPC credentials for remote signing, derivation paths
|
|
// for HD wallets, and more.
|
|
func EditWalletConfiguration(cliCtx *cli.Context) error {
|
|
ctx := context.Background()
|
|
wallet, err := OpenWallet(cliCtx)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not open wallet")
|
|
}
|
|
switch wallet.KeymanagerKind() {
|
|
case v2keymanager.Direct:
|
|
enc, err := wallet.ReadKeymanagerConfigFromDisk(ctx)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not read config")
|
|
}
|
|
cfg, err := direct.UnmarshalConfigFile(enc)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not unmarshal config")
|
|
}
|
|
log.Info("Current configuration")
|
|
// Prints the current configuration to stdout.
|
|
fmt.Println(cfg)
|
|
passwordsDir, err := inputDirectory(cliCtx, passwordsDirPromptText, flags.WalletPasswordsDirFlag)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not get password directory")
|
|
}
|
|
defaultCfg := direct.DefaultConfig()
|
|
defaultCfg.AccountPasswordsDirectory = passwordsDir
|
|
encodedCfg, err := direct.MarshalConfigFile(ctx, defaultCfg)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not marshal config file")
|
|
}
|
|
if err := wallet.WriteKeymanagerConfigToDisk(ctx, encodedCfg); err != nil {
|
|
return errors.Wrap(err, "could not write config to disk")
|
|
}
|
|
case v2keymanager.Derived:
|
|
return errors.New("derived keymanager is not yet supported")
|
|
case v2keymanager.Remote:
|
|
enc, err := wallet.ReadKeymanagerConfigFromDisk(ctx)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not read config")
|
|
}
|
|
cfg, err := remote.UnmarshalConfigFile(enc)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not unmarshal config")
|
|
}
|
|
log.Info("Current configuration")
|
|
// Prints the current configuration to stdout.
|
|
fmt.Println(cfg)
|
|
newCfg, err := inputRemoteKeymanagerConfig(cliCtx)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not get keymanager config")
|
|
}
|
|
encodedCfg, err := remote.MarshalConfigFile(ctx, newCfg)
|
|
if err != nil {
|
|
return errors.Wrap(err, "could not marshal config file")
|
|
}
|
|
if err := wallet.WriteKeymanagerConfigToDisk(ctx, encodedCfg); err != nil {
|
|
return errors.Wrap(err, "could not write config to disk")
|
|
}
|
|
default:
|
|
return fmt.Errorf("keymanager type %s is not supported", wallet.KeymanagerKind())
|
|
}
|
|
return nil
|
|
}
|