mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
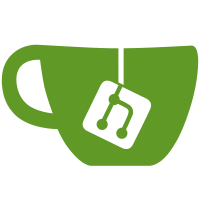
* Verify attestations before putting them into the pool * use existing method * Validate aggregated ones too * Revert "Validate aggregated ones too" This reverts commit a55646d131813c3e65e0539ef5b2e5bda19a5e91. * Merge branch 'master' of github.com:prysmaticlabs/prysm into verify-all-atts * Add feature flag * The remaining shared reference fields with conditional copy on write * Merge branch 'master' into better-copy-2 * Merge branch 'better-copy-2' of github.com:prysmaticlabs/prysm into verify-all-atts * gaz * fix build, put into validate * lint * Merge branch 'master' of github.com:prysmaticlabs/prysm into verify-all-atts * why does goland do this to me * revert unrelated change * fix tests * Update shared/featureconfig/config.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * Merge refs/heads/master into verify-all-atts * Update beacon-chain/blockchain/testing/mock.go Co-Authored-By: terence tsao <terence@prysmaticlabs.com> * gofmt
154 lines
4.3 KiB
Go
154 lines
4.3 KiB
Go
package sync
|
|
|
|
import (
|
|
"bytes"
|
|
"context"
|
|
"testing"
|
|
"time"
|
|
|
|
pubsub "github.com/libp2p/go-libp2p-pubsub"
|
|
pubsubpb "github.com/libp2p/go-libp2p-pubsub/pb"
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/go-bitfield"
|
|
"github.com/prysmaticlabs/go-ssz"
|
|
mockChain "github.com/prysmaticlabs/prysm/beacon-chain/blockchain/testing"
|
|
dbtest "github.com/prysmaticlabs/prysm/beacon-chain/db/testing"
|
|
p2ptest "github.com/prysmaticlabs/prysm/beacon-chain/p2p/testing"
|
|
mockSync "github.com/prysmaticlabs/prysm/beacon-chain/sync/initial-sync/testing"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
)
|
|
|
|
func TestService_validateCommitteeIndexBeaconAttestation(t *testing.T) {
|
|
ctx := context.Background()
|
|
p := p2ptest.NewTestP2P(t)
|
|
db := dbtest.SetupDB(t)
|
|
defer dbtest.TeardownDB(t, db)
|
|
chain := &mockChain.ChainService{
|
|
Genesis: time.Now().Add(time.Duration(-64*int64(params.BeaconConfig().SecondsPerSlot)) * time.Second), // 64 slots ago
|
|
ValidAttestation: true,
|
|
}
|
|
s := &Service{
|
|
initialSync: &mockSync.Sync{IsSyncing: false},
|
|
p2p: p,
|
|
db: db,
|
|
chain: chain,
|
|
blkRootToPendingAtts: make(map[[32]byte][]*ethpb.AggregateAttestationAndProof),
|
|
}
|
|
|
|
blk := ðpb.SignedBeaconBlock{
|
|
Block: ðpb.BeaconBlock{
|
|
Slot: 55,
|
|
},
|
|
}
|
|
if err := db.SaveBlock(ctx, blk); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
validBlockRoot, err := ssz.HashTreeRoot(blk.Block)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
tests := []struct {
|
|
name string
|
|
msg *ethpb.Attestation
|
|
topic string
|
|
validAttestationSignature bool
|
|
want bool
|
|
}{
|
|
{
|
|
name: "validAttestationSignature",
|
|
msg: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0b1010},
|
|
Data: ðpb.AttestationData{
|
|
BeaconBlockRoot: validBlockRoot[:],
|
|
CommitteeIndex: 1,
|
|
Slot: 63,
|
|
},
|
|
},
|
|
topic: "/eth2/committee_index1_beacon_attestation",
|
|
validAttestationSignature: true,
|
|
want: true,
|
|
},
|
|
{
|
|
name: "wrong committee index",
|
|
msg: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0b1010},
|
|
Data: ðpb.AttestationData{
|
|
BeaconBlockRoot: validBlockRoot[:],
|
|
CommitteeIndex: 2,
|
|
Slot: 63,
|
|
},
|
|
},
|
|
topic: "/eth2/committee_index3_beacon_attestation",
|
|
validAttestationSignature: true,
|
|
want: false,
|
|
},
|
|
{
|
|
name: "already aggregated",
|
|
msg: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0b1011},
|
|
Data: ðpb.AttestationData{
|
|
BeaconBlockRoot: validBlockRoot[:],
|
|
CommitteeIndex: 1,
|
|
Slot: 63,
|
|
},
|
|
},
|
|
topic: "/eth2/committee_index1_beacon_attestation",
|
|
validAttestationSignature: true,
|
|
want: false,
|
|
},
|
|
{
|
|
name: "missing block",
|
|
msg: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0b1010},
|
|
Data: ðpb.AttestationData{
|
|
BeaconBlockRoot: []byte("missing"),
|
|
CommitteeIndex: 1,
|
|
Slot: 63,
|
|
},
|
|
},
|
|
topic: "/eth2/committee_index1_beacon_attestation",
|
|
validAttestationSignature: true,
|
|
want: false,
|
|
},
|
|
{
|
|
name: "invalid attestation",
|
|
msg: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0b1010},
|
|
Data: ðpb.AttestationData{
|
|
BeaconBlockRoot: validBlockRoot[:],
|
|
CommitteeIndex: 1,
|
|
Slot: 63,
|
|
},
|
|
},
|
|
topic: "/eth2/committee_index1_beacon_attestation",
|
|
validAttestationSignature: false,
|
|
want: false,
|
|
},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
buf := new(bytes.Buffer)
|
|
_, err := p.Encoding().Encode(buf, tt.msg)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
m := &pubsub.Message{
|
|
Message: &pubsubpb.Message{
|
|
Data: buf.Bytes(),
|
|
TopicIDs: []string{tt.topic},
|
|
},
|
|
}
|
|
chain.ValidAttestation = tt.validAttestationSignature
|
|
if s.validateCommitteeIndexBeaconAttestation(ctx, "" /*peerID*/, m) != tt.want {
|
|
t.Errorf("Did not received wanted validation. Got %v, wanted %v", !tt.want, tt.want)
|
|
}
|
|
if tt.want && m.ValidatorData == nil {
|
|
t.Error("Expected validator data to be set")
|
|
}
|
|
})
|
|
}
|
|
}
|