mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
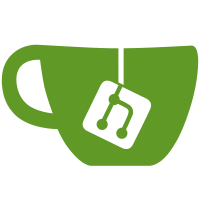
* add forkchoice to stategen.New, update everywhere * conflict_1 * Fix proposer_bellatrix test Co-authored-by: Potuz <potuz@prysmaticlabs.com>
51 lines
1.5 KiB
Go
51 lines
1.5 KiB
Go
package blockchain
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"testing"
|
|
|
|
testDB "github.com/prysmaticlabs/prysm/v3/beacon-chain/db/testing"
|
|
doublylinkedtree "github.com/prysmaticlabs/prysm/v3/beacon-chain/forkchoice/doubly-linked-tree"
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/state"
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/state/stategen"
|
|
)
|
|
|
|
func testServiceOptsWithDB(t *testing.T) []Option {
|
|
beaconDB := testDB.SetupDB(t)
|
|
fcs := doublylinkedtree.New()
|
|
return []Option{
|
|
WithDatabase(beaconDB),
|
|
WithStateGen(stategen.New(beaconDB, fcs)),
|
|
WithForkChoiceStore(fcs),
|
|
}
|
|
}
|
|
|
|
// WARNING: only use these opts when you are certain there are no db calls
|
|
// in your code path. this is a lightweight way to satisfy the stategen/beacondb
|
|
// initialization requirements w/o the overhead of db init.
|
|
func testServiceOptsNoDB() []Option {
|
|
return []Option{
|
|
withStateBalanceCache(satisfactoryStateBalanceCache()),
|
|
}
|
|
}
|
|
|
|
type mockStateByRooter struct {
|
|
state state.BeaconState
|
|
err error
|
|
}
|
|
|
|
var _ stateByRooter = &mockStateByRooter{}
|
|
|
|
func (m mockStateByRooter) StateByRoot(_ context.Context, _ [32]byte) (state.BeaconState, error) {
|
|
return m.state, m.err
|
|
}
|
|
|
|
// returns an instance of the state balance cache that can be used
|
|
// to satisfy the requirement for one in NewService, but which will
|
|
// always return an error if used.
|
|
func satisfactoryStateBalanceCache() *stateBalanceCache {
|
|
err := errors.New("satisfactoryStateBalanceCache doesn't perform real caching")
|
|
return &stateBalanceCache{stateGen: mockStateByRooter{err: err}}
|
|
}
|