mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
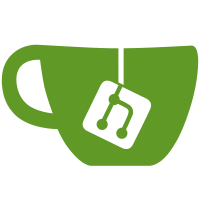
* do not use batch for SaveAttestations * use snappy compression * Encode / decode everything with snappy * Add snappy migration path * batch is probably fine... * fix test * gofmt * Merge branch 'master' of github.com:prysmaticlabs/prysm into remove-batch-attestations * add sanity check * remove that thing * gaz * Merge branch 'master' of github.com:prysmaticlabs/prysm into remove-batch-attestations
35 lines
656 B
Go
35 lines
656 B
Go
package kv
|
|
|
|
import (
|
|
"github.com/gogo/protobuf/proto"
|
|
"github.com/golang/snappy"
|
|
"github.com/prysmaticlabs/prysm/shared/featureconfig"
|
|
)
|
|
|
|
func decode(data []byte, dst proto.Message) error {
|
|
if featureconfig.Get().EnableSnappyDBCompression {
|
|
var err error
|
|
data, err = snappy.Decode(nil, data)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
}
|
|
if err := proto.Unmarshal(data, dst); err != nil {
|
|
return err
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func encode(msg proto.Message) ([]byte, error) {
|
|
enc, err := proto.Marshal(msg)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if !featureconfig.Get().EnableSnappyDBCompression {
|
|
return enc, nil
|
|
}
|
|
|
|
return snappy.Encode(nil, enc), nil
|
|
}
|