mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
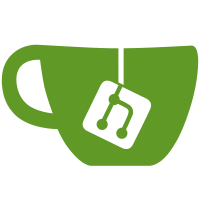
* include migration and logic for stopping early in slashing protection checks * remove commented code * extract methods * migration logic tested up * migration up and down tests * Update validator/db/kv/attester_protection.go Co-authored-by: terence tsao <terence@prysmaticlabs.com> * added in pruning and batched migrations Co-authored-by: Victor Farazdagi <simple.square@gmail.com> Co-authored-by: terence tsao <terence@prysmaticlabs.com>
60 lines
1.2 KiB
Go
60 lines
1.2 KiB
Go
package kv
|
|
|
|
import (
|
|
"context"
|
|
|
|
bolt "go.etcd.io/bbolt"
|
|
)
|
|
|
|
type migration func(*bolt.Tx) error
|
|
|
|
var (
|
|
migrationCompleted = []byte("done")
|
|
upMigrations []migration
|
|
downMigrations []migration
|
|
)
|
|
|
|
// RunUpMigrations defined in the upMigrations list.
|
|
func (s *Store) RunUpMigrations(ctx context.Context) error {
|
|
// Run any special migrations that require special conditions.
|
|
if err := s.migrateOptimalAttesterProtectionUp(ctx); err != nil {
|
|
return err
|
|
}
|
|
if err := s.migrateSourceTargetEpochsBucketUp(ctx); err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, m := range upMigrations {
|
|
if ctx.Err() != nil {
|
|
return ctx.Err()
|
|
}
|
|
|
|
if err := s.db.Update(m); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// RunDownMigrations defined in the downMigrations list.
|
|
func (s *Store) RunDownMigrations(ctx context.Context) error {
|
|
// Run any special migrations that require special conditions.
|
|
if err := s.migrateOptimalAttesterProtectionDown(ctx); err != nil {
|
|
return err
|
|
}
|
|
if err := s.migrateSourceTargetEpochsBucketDown(ctx); err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, m := range downMigrations {
|
|
if ctx.Err() != nil {
|
|
return ctx.Err()
|
|
}
|
|
|
|
if err := s.db.Update(m); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|