mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 13:18:57 +00:00
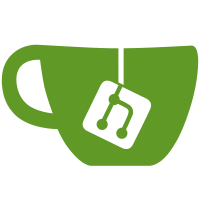
* Reverse broadcast-slashings to disable-broadcast-slashings * Fix tests * Merge branch 'master' of github.com:prysmaticlabs/prysm into toggle-broadcast-flag * Merge refs/heads/master into toggle-broadcast-flag * Fix for comments * Merge branch 'toggle-broadcast-flag' of github.com:prysmaticlabs/prysm into toggle-broadcast-flag * Merge refs/heads/master into toggle-broadcast-flag
61 lines
2.2 KiB
Go
61 lines
2.2 KiB
Go
package beacon
|
|
|
|
import (
|
|
"context"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/featureconfig"
|
|
"github.com/prysmaticlabs/prysm/shared/sliceutil"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
)
|
|
|
|
// SubmitProposerSlashing receives a proposer slashing object via
|
|
// RPC and injects it into the beacon node's operations pool.
|
|
// Submission into this pool does not guarantee inclusion into a beacon block.
|
|
func (bs *Server) SubmitProposerSlashing(
|
|
ctx context.Context,
|
|
req *ethpb.ProposerSlashing,
|
|
) (*ethpb.SubmitSlashingResponse, error) {
|
|
beaconState, err := bs.HeadFetcher.HeadState(ctx)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not retrieve head state: %v", err)
|
|
}
|
|
if err := bs.SlashingsPool.InsertProposerSlashing(ctx, beaconState, req); err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not insert proposer slashing into pool: %v", err)
|
|
}
|
|
if !featureconfig.Get().DisableBroadcastSlashings {
|
|
if err := bs.Broadcaster.Broadcast(ctx, req); err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
return ðpb.SubmitSlashingResponse{
|
|
SlashedIndices: []uint64{req.Header_1.Header.ProposerIndex},
|
|
}, nil
|
|
}
|
|
|
|
// SubmitAttesterSlashing receives an attester slashing object via
|
|
// RPC and injects it into the beacon node's operations pool.
|
|
// Submission into this pool does not guarantee inclusion into a beacon block.
|
|
func (bs *Server) SubmitAttesterSlashing(
|
|
ctx context.Context,
|
|
req *ethpb.AttesterSlashing,
|
|
) (*ethpb.SubmitSlashingResponse, error) {
|
|
beaconState, err := bs.HeadFetcher.HeadState(ctx)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not retrieve head state: %v", err)
|
|
}
|
|
if err := bs.SlashingsPool.InsertAttesterSlashing(ctx, beaconState, req); err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not insert attester slashing into pool: %v", err)
|
|
}
|
|
if !featureconfig.Get().DisableBroadcastSlashings {
|
|
if err := bs.Broadcaster.Broadcast(ctx, req); err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
slashedIndices := sliceutil.IntersectionUint64(req.Attestation_1.AttestingIndices, req.Attestation_2.AttestingIndices)
|
|
return ðpb.SubmitSlashingResponse{
|
|
SlashedIndices: slashedIndices,
|
|
}, nil
|
|
}
|