mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 21:27:19 +00:00
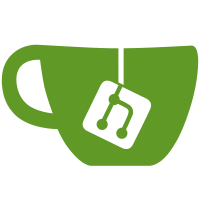
* parent c837dfb2f2
author dv8silencer <dv8silencer+github@gmail.com> 1592805180 -0500
committer dv8silencer <dv8silencer+github@gmail.com> 1592855989 -0500
Create a bootstrap-node-file flag which reads nodes from a YAML file and make the flag mutually exclusive to the bootstrap-node flag
* Merge branch 'master' into dv8s-iss6316
* Refactor so that boot node reading is in its own function. Added test
* Added period
Co-authored-by: terence tsao <terence@prysmaticlabs.com>
* Merge branch 'master' into dv8s-iss6316
* Edit Bazel to include go-yaml dep
* Help Bazel build successfully
* Merge branch 'master' into dv8s-iss6316
* bazel run //:gazella -- fix
* Handle error from YAML parser to address build checks
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Updated flags.go to refine the Usage for the BootStrapNodeFile flag
Co-authored-by: Nishant Das <nish1993@hotmail.com>
* Merge branch 'master' into dv8s-iss6316
* Utilize stdlib TempFile to avoid polluting
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* merge master into this branch
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Merge branch 'master' into dv8s-iss6316
* Changed bootstrap-node to StringSlice flag to allow multiple nodes to be passed. Each value can be .enr file which will be YAML parsed to extract nodes
* Refactored to create separate readbootNodes function and added a test for it.
* More cleaning up
* Changed wording in the cli help for --bootstrap-node
* Merge branch 'master' into dv8s-iss6316
* Since we are taking YAML files, got rid of .enr check and instead we check for the string prefix of a enr record or not to determine if file.
* Merge with dv8silencer/tempissue6316
* Correct spacing
Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com>
* Changed how we check for YAML file vs CLI-direct ENR
* Minor: Changed to 1 string from concatenating 2
* Merge branch 'master' into dv8s-iss6316
82 lines
2.3 KiB
Go
82 lines
2.3 KiB
Go
package node
|
|
|
|
import (
|
|
"flag"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
"testing"
|
|
|
|
statefeed "github.com/prysmaticlabs/prysm/beacon-chain/core/feed/state"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
"github.com/urfave/cli/v2"
|
|
)
|
|
|
|
// Ensure BeaconNode implements interfaces.
|
|
var _ = statefeed.Notifier(&BeaconNode{})
|
|
|
|
// Test that beacon chain node can close.
|
|
func TestNodeClose_OK(t *testing.T) {
|
|
hook := logTest.NewGlobal()
|
|
|
|
tmp := fmt.Sprintf("%s/datadirtest2", testutil.TempDir())
|
|
if err := os.RemoveAll(tmp); err != nil {
|
|
t.Log(err)
|
|
}
|
|
|
|
app := cli.App{}
|
|
set := flag.NewFlagSet("test", 0)
|
|
set.Bool("test-skip-pow", true, "skip pow dial")
|
|
set.String("datadir", tmp, "node data directory")
|
|
set.String("p2p-encoding", "ssz", "p2p encoding scheme")
|
|
set.Bool("demo-config", true, "demo configuration")
|
|
set.String("deposit-contract", "0x0000000000000000000000000000000000000000", "deposit contract address")
|
|
|
|
context := cli.NewContext(&app, set, nil)
|
|
|
|
node, err := NewBeaconNode(context)
|
|
if err != nil {
|
|
t.Fatalf("Failed to create BeaconNode: %v", err)
|
|
}
|
|
|
|
node.Close()
|
|
|
|
testutil.AssertLogsContain(t, hook, "Stopping beacon node")
|
|
|
|
if err := os.RemoveAll(tmp); err != nil {
|
|
t.Log(err)
|
|
}
|
|
}
|
|
|
|
func TestBootStrapNodeFile(t *testing.T) {
|
|
file, err := ioutil.TempFile(testutil.TempDir(), "bootstrapFile")
|
|
if err != nil {
|
|
t.Fatalf("Error in TempFile call: %v", err)
|
|
}
|
|
defer func() {
|
|
if err := os.Remove(file.Name()); err != nil {
|
|
t.Log(err)
|
|
}
|
|
}()
|
|
|
|
sampleNode0 := "- enr:-Ku4QMKVC_MowDsmEa20d5uGjrChI0h8_KsKXDmgVQbIbngZV0i" +
|
|
"dV6_RL7fEtZGo-kTNZ5o7_EJI_vCPJ6scrhwX0Z4Bh2F0dG5ldHOIAAAAAAAAAACEZXRoMpD" +
|
|
"1pf1CAAAAAP__________gmlkgnY0gmlwhBLf22SJc2VjcDI1NmsxoQJxCnE6v_x2ekgY_uo" +
|
|
"E1rtwzvGy40mq9eD66XfHPBWgIIN1ZHCCD6A"
|
|
sampleNode1 := "- enr:-TESTNODE2"
|
|
sampleNode2 := "- enr:-TESTNODE3"
|
|
err = ioutil.WriteFile(file.Name(), []byte(sampleNode0+"\n"+sampleNode1+"\n"+sampleNode2), 0644)
|
|
if err != nil {
|
|
t.Fatalf("Error in WriteFile call: %v", err)
|
|
}
|
|
nodeList, err := readbootNodes(file.Name())
|
|
if err != nil {
|
|
t.Fatalf("Error in readbootNodes call: %v", err)
|
|
}
|
|
if nodeList[0] != sampleNode0[2:] || nodeList[1] != sampleNode1[2:] || nodeList[2] != sampleNode2[2:] {
|
|
// nodeList's YAML parsing will have removed the leading "- "
|
|
t.Fatalf("TestBootStrapNodeFile failed. Nodes do not match")
|
|
}
|
|
}
|