mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
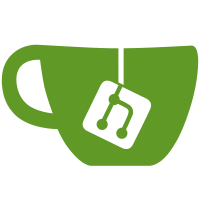
* Applies assertion funcs to validator/client tests * gazelle * Merge branch 'master' into validator-client-apply-testutils-assertions * Merge refs/heads/master into validator-client-apply-testutils-assertions * Merge refs/heads/master into validator-client-apply-testutils-assertions * Merge refs/heads/master into validator-client-apply-testutils-assertions * Merge refs/heads/master into validator-client-apply-testutils-assertions * Merge refs/heads/master into validator-client-apply-testutils-assertions * Merge refs/heads/master into validator-client-apply-testutils-assertions
60 lines
1.9 KiB
Go
60 lines
1.9 KiB
Go
package client
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/featureconfig"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
mockSlasher "github.com/prysmaticlabs/prysm/validator/testing"
|
|
)
|
|
|
|
func TestPreBlockSignValidation(t *testing.T) {
|
|
config := &featureconfig.Flags{
|
|
LocalProtection: false,
|
|
SlasherProtection: true,
|
|
}
|
|
reset := featureconfig.InitWithReset(config)
|
|
defer reset()
|
|
validator, _, finish := setup(t)
|
|
defer finish()
|
|
|
|
block := ðpb.BeaconBlock{
|
|
Slot: 10,
|
|
ProposerIndex: 0,
|
|
}
|
|
mockProtector := &mockSlasher.MockProtector{AllowBlock: false}
|
|
validator.protector = mockProtector
|
|
err := validator.preBlockSignValidations(context.Background(), validatorPubKey, block)
|
|
require.ErrorContains(t, failedPreBlockSignExternalErr, err)
|
|
mockProtector.AllowBlock = true
|
|
err = validator.preBlockSignValidations(context.Background(), validatorPubKey, block)
|
|
require.NoError(t, err, "Expected allowed attestation not to throw error")
|
|
}
|
|
|
|
func TestPostBlockSignUpdate(t *testing.T) {
|
|
config := &featureconfig.Flags{
|
|
LocalProtection: false,
|
|
SlasherProtection: true,
|
|
}
|
|
reset := featureconfig.InitWithReset(config)
|
|
defer reset()
|
|
validator, _, finish := setup(t)
|
|
defer finish()
|
|
|
|
block := ðpb.SignedBeaconBlock{
|
|
Block: ðpb.BeaconBlock{
|
|
Slot: 10,
|
|
ProposerIndex: 0,
|
|
},
|
|
}
|
|
mockProtector := &mockSlasher.MockProtector{AllowBlock: false}
|
|
validator.protector = mockProtector
|
|
err := validator.postBlockSignUpdate(context.Background(), validatorPubKey, block)
|
|
require.ErrorContains(t, failedPostBlockSignErr, err, "Expected error when post signature update is detected as slashable")
|
|
mockProtector.AllowBlock = true
|
|
err = validator.postBlockSignUpdate(context.Background(), validatorPubKey, block)
|
|
require.NoError(t, err, "Expected allowed attestation not to throw error")
|
|
}
|