mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 04:47:18 +00:00
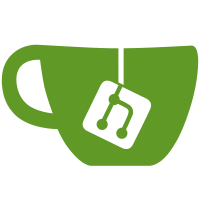
* adding flags * adding modified key utils * adding more funcs * more changes * more changes * documentation * changes to node * gazelle * fixing bazel build * gazelle * adding tests * more tests * addressing terence's feedback * adding geth header * test * changes * fixedd it * fixed marshalling * adding more to tests * gazelle * adding more tests * lint * add cov * cov * fix imports
89 lines
2.5 KiB
Go
89 lines
2.5 KiB
Go
// Package bls implements a go-wrapper around a C BLS library leveraging
|
|
// the BLS12-381 curve. This package exposes a public API for verifying and
|
|
// aggregating BLS signatures used by Ethereum Serenity.
|
|
package bls
|
|
|
|
import (
|
|
"fmt"
|
|
"math/big"
|
|
)
|
|
|
|
// Signature used in the BLS signature scheme.
|
|
type Signature struct{}
|
|
|
|
// SecretKey used in the BLS scheme.
|
|
type SecretKey struct {
|
|
K *big.Int
|
|
}
|
|
|
|
// PublicKey corresponding to secret key used in the BLS scheme.
|
|
type PublicKey struct{}
|
|
|
|
// PublicKey returns the corresponding public key for the
|
|
// Secret Key
|
|
func (s *SecretKey) PublicKey() (*PublicKey, error) {
|
|
return &PublicKey{}, nil
|
|
}
|
|
|
|
// BufferedSecretKey returns the secret key in a byte format.
|
|
func (s *SecretKey) BufferedSecretKey() []byte {
|
|
return s.K.Bytes()
|
|
}
|
|
|
|
// BufferedPublicKey returns the public key in a byte format.
|
|
func (p *PublicKey) BufferedPublicKey() []byte {
|
|
return []byte{}
|
|
}
|
|
|
|
// UnBufferSecretKey takes the byte representation of a secret key
|
|
// and sets it to a big int of the underlying secret key object.
|
|
func (s *SecretKey) UnBufferSecretKey(bufferedKey []byte) {
|
|
s.K = big.NewInt(0).SetBytes(bufferedKey)
|
|
|
|
}
|
|
|
|
// UnBufferPublicKey takes the byte representation of a public key
|
|
// and sets it to a big int of the underlying public key object.
|
|
func (p *PublicKey) UnBufferPublicKey(bufferedKey []byte) {
|
|
|
|
}
|
|
|
|
// GenerateKey generates a new secret key using a seed.
|
|
func GenerateKey(seed []byte) *SecretKey {
|
|
return &SecretKey{
|
|
K: big.NewInt(0).SetBytes(seed),
|
|
}
|
|
}
|
|
|
|
// Sign a message using a secret key - in a beacon/validator client,
|
|
// this key will come from and be unlocked from the account keystore.
|
|
func Sign(sec *SecretKey, msg []byte) (*Signature, error) {
|
|
return &Signature{}, nil
|
|
}
|
|
|
|
// VerifySig against a public key.
|
|
func VerifySig(pub *PublicKey, msg []byte, sig *Signature) (bool, error) {
|
|
return true, nil
|
|
}
|
|
|
|
// VerifyAggregateSig created using the underlying BLS signature
|
|
// aggregation scheme.
|
|
func VerifyAggregateSig(pubs []*PublicKey, msg []byte, asig *Signature) (bool, error) {
|
|
return true, nil
|
|
}
|
|
|
|
// BatchVerify a list of individual signatures by aggregating them.
|
|
func BatchVerify(pubs []*PublicKey, msg []byte, sigs []*Signature) (bool, error) {
|
|
asigs, err := AggregateSigs(sigs)
|
|
if err != nil {
|
|
return false, fmt.Errorf("could not aggregate signatures: %v", err)
|
|
}
|
|
return VerifyAggregateSig(pubs, msg, asigs)
|
|
}
|
|
|
|
// AggregateSigs puts multiple signatures into one using the underlying
|
|
// BLS sum functions.
|
|
func AggregateSigs(sigs []*Signature) (*Signature, error) {
|
|
return &Signature{}, nil
|
|
}
|