mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 04:47:18 +00:00
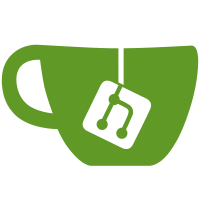
* polling interval * adding proto message * changing proto messages * changing naming * adding slot functionality * initial sync working * new changes * more sync fixes * its working now * finally working * add tests * fix tests * tests * adding tests * lint * log checks * making changes to simulator * update logs * fix tests * get sync to work with crystallized state * fixing race * making requested changes * unexport * documentation * gazelle and fix merge conflicts * adding repeated requests * fix lint * adding new clock , db methods, and util func * revert change to test * gazelle * add in test * gazelle * finally working * save slot * fix lint and constant
125 lines
2.4 KiB
Go
125 lines
2.4 KiB
Go
package bytes
|
|
|
|
import (
|
|
"bytes"
|
|
"testing"
|
|
)
|
|
|
|
func TestBytes1(t *testing.T) {
|
|
tests := []struct {
|
|
a uint64
|
|
b []byte
|
|
}{
|
|
{0, []byte{0}},
|
|
{1, []byte{1}},
|
|
{2, []byte{2}},
|
|
{253, []byte{253}},
|
|
{254, []byte{254}},
|
|
{255, []byte{255}},
|
|
}
|
|
for _, tt := range tests {
|
|
b := Bytes1(tt.a)
|
|
if !bytes.Equal(b, tt.b) {
|
|
t.Errorf("Bytes1(%d) = %v, want = %d", tt.a, b, tt.b)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestBytes2(t *testing.T) {
|
|
tests := []struct {
|
|
a uint64
|
|
b []byte
|
|
}{
|
|
{0, []byte{0, 0}},
|
|
{1, []byte{0, 1}},
|
|
{255, []byte{0, 255}},
|
|
{256, []byte{1, 0}},
|
|
{65534, []byte{255, 254}},
|
|
{65535, []byte{255, 255}},
|
|
}
|
|
for _, tt := range tests {
|
|
b := Bytes2(tt.a)
|
|
if !bytes.Equal(b, tt.b) {
|
|
t.Errorf("Bytes2(%d) = %v, want = %d", tt.a, b, tt.b)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestBytes3(t *testing.T) {
|
|
tests := []struct {
|
|
a uint64
|
|
b []byte
|
|
}{
|
|
{0, []byte{0, 0, 0}},
|
|
{255, []byte{0, 0, 255}},
|
|
{256, []byte{0, 1, 0}},
|
|
{65535, []byte{0, 255, 255}},
|
|
{65536, []byte{1, 0, 0}},
|
|
{16777215, []byte{255, 255, 255}},
|
|
}
|
|
for _, tt := range tests {
|
|
b := Bytes3(tt.a)
|
|
if !bytes.Equal(b, tt.b) {
|
|
t.Errorf("Bytes3(%d) = %v, want = %d", tt.a, b, tt.b)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestBytes4(t *testing.T) {
|
|
tests := []struct {
|
|
a uint64
|
|
b []byte
|
|
}{
|
|
{0, []byte{0, 0, 0, 0}},
|
|
{256, []byte{0, 0, 1, 0}},
|
|
{65536, []byte{0, 1, 0, 0}},
|
|
{16777216, []byte{1, 0, 0, 0}},
|
|
{16777217, []byte{1, 0, 0, 1}},
|
|
{4294967295, []byte{255, 255, 255, 255}},
|
|
}
|
|
for _, tt := range tests {
|
|
b := Bytes4(tt.a)
|
|
if !bytes.Equal(b, tt.b) {
|
|
t.Errorf("Bytes4(%d) = %v, want = %d", tt.a, b, tt.b)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestBytes8(t *testing.T) {
|
|
tests := []struct {
|
|
a uint64
|
|
b []byte
|
|
}{
|
|
{0, []byte{0, 0, 0, 0, 0, 0, 0, 0}},
|
|
{16777216, []byte{0, 0, 0, 0, 1, 0, 0, 0}},
|
|
{4294967296, []byte{0, 0, 0, 1, 0, 0, 0, 0}},
|
|
{4294967297, []byte{0, 0, 0, 1, 0, 0, 0, 1}},
|
|
{9223372036854775806, []byte{127, 255, 255, 255, 255, 255, 255, 254}},
|
|
{9223372036854775807, []byte{127, 255, 255, 255, 255, 255, 255, 255}},
|
|
}
|
|
for _, tt := range tests {
|
|
b := Bytes8(tt.a)
|
|
if !bytes.Equal(b, tt.b) {
|
|
t.Errorf("Bytes8(%d) = %v, want = %d", tt.a, b, tt.b)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestFromBytes8(t *testing.T) {
|
|
tests := []uint64{
|
|
0,
|
|
1776,
|
|
96726,
|
|
4290997,
|
|
922376854775806,
|
|
42893720984775807,
|
|
}
|
|
for _, tt := range tests {
|
|
b := Bytes8(tt)
|
|
c := FromBytes8(b)
|
|
if c != tt {
|
|
t.Errorf("Wanted %d but got %d", tt, c)
|
|
}
|
|
}
|
|
}
|