mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-24 12:27:18 +00:00
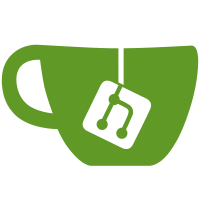
* add context to beacon APIs * add TODO to merge GET and POST methods * fix linter action Co-authored-by: kasey <489222+kasey@users.noreply.github.com> Co-authored-by: james-prysm <90280386+james-prysm@users.noreply.github.com>
34 lines
1.0 KiB
Go
34 lines
1.0 KiB
Go
package beacon_api
|
|
|
|
import (
|
|
"context"
|
|
"strconv"
|
|
|
|
"github.com/ethereum/go-ethereum/common/hexutil"
|
|
"github.com/pkg/errors"
|
|
types "github.com/prysmaticlabs/prysm/v3/consensus-types/primitives"
|
|
ethpb "github.com/prysmaticlabs/prysm/v3/proto/prysm/v1alpha1"
|
|
)
|
|
|
|
func (c beaconApiValidatorClient) validatorIndex(ctx context.Context, in *ethpb.ValidatorIndexRequest) (*ethpb.ValidatorIndexResponse, error) {
|
|
stringPubKey := hexutil.Encode(in.PublicKey)
|
|
|
|
stateValidator, err := c.stateValidatorsProvider.GetStateValidators(ctx, []string{stringPubKey}, nil, nil)
|
|
if err != nil {
|
|
return nil, errors.Wrap(err, "failed to get state validator")
|
|
}
|
|
|
|
if len(stateValidator.Data) == 0 {
|
|
return nil, errors.Errorf("could not find validator index for public key `%s`", stringPubKey)
|
|
}
|
|
|
|
stringValidatorIndex := stateValidator.Data[0].Index
|
|
|
|
index, err := strconv.ParseUint(stringValidatorIndex, 10, 64)
|
|
if err != nil {
|
|
return nil, errors.Wrap(err, "failed to parse validator index")
|
|
}
|
|
|
|
return ðpb.ValidatorIndexResponse{Index: types.ValidatorIndex(index)}, nil
|
|
}
|