mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-08 02:31:19 +00:00
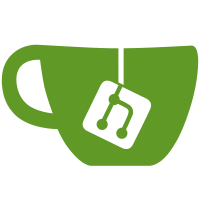
* fork * types * cloners * getters * remove CapellaBlind from fork * hasher * setters * spec params, config tests * generate ssz * executionPayloadHeaderCapella * proto state * BeaconStateCapella SSZ * saving state * configfork * BUILD files * fix RealPosition * fix hasher * SetLatestExecutionPayloadHeaderCapella * fix error message * reduce complexity of saveStatesEfficientInternal * add latestExecutionPayloadHeaderCapella to minimal state * halway done interface * remove withdrawal methods * merge setters * change signatures for v1 and v2 * fixing errors pt. 1 * paylod_test fixes * fix everything * remove unused func * fix tests * state_trie_test improvements * in progress... * hasher test * fix configs * simplify hashing * Revert "fix configs" This reverts commit bcae2825fcc8ba45a2b43d68ad0ab57f8eac8952. * remove capella from config test * unify locking * review * hashing * fixes Co-authored-by: terencechain <terence@prysmaticlabs.com> Co-authored-by: Raul Jordan <raul@prysmaticlabs.com>
95 lines
3.0 KiB
Go
95 lines
3.0 KiB
Go
package state_native
|
|
|
|
import (
|
|
"testing"
|
|
|
|
nativetypes "github.com/prysmaticlabs/prysm/v3/beacon-chain/state/state-native/types"
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/state/stateutil"
|
|
enginev1 "github.com/prysmaticlabs/prysm/v3/proto/engine/v1"
|
|
"github.com/prysmaticlabs/prysm/v3/runtime/version"
|
|
"github.com/prysmaticlabs/prysm/v3/testing/assert"
|
|
"github.com/prysmaticlabs/prysm/v3/testing/require"
|
|
)
|
|
|
|
func TestSetWithdrawalQueue(t *testing.T) {
|
|
t.Run("ok", func(t *testing.T) {
|
|
oldQ := []*enginev1.Withdrawal{
|
|
{
|
|
WithdrawalIndex: 0,
|
|
ExecutionAddress: []byte("address1"),
|
|
Amount: 1,
|
|
},
|
|
{
|
|
WithdrawalIndex: 1,
|
|
ExecutionAddress: []byte("address2"),
|
|
Amount: 2,
|
|
},
|
|
}
|
|
newQ := []*enginev1.Withdrawal{
|
|
{
|
|
WithdrawalIndex: 2,
|
|
ExecutionAddress: []byte("address3"),
|
|
Amount: 3,
|
|
},
|
|
{
|
|
WithdrawalIndex: 3,
|
|
ExecutionAddress: []byte("address4"),
|
|
Amount: 4,
|
|
},
|
|
}
|
|
s := BeaconState{
|
|
version: version.Capella,
|
|
withdrawalQueue: oldQ,
|
|
sharedFieldReferences: map[nativetypes.FieldIndex]*stateutil.Reference{nativetypes.WithdrawalQueue: stateutil.NewRef(1)},
|
|
dirtyFields: map[nativetypes.FieldIndex]bool{},
|
|
rebuildTrie: map[nativetypes.FieldIndex]bool{},
|
|
}
|
|
err := s.SetWithdrawalQueue(newQ)
|
|
require.NoError(t, err)
|
|
assert.DeepEqual(t, newQ, s.withdrawalQueue)
|
|
})
|
|
t.Run("version before Capella not supported", func(t *testing.T) {
|
|
s := BeaconState{version: version.Bellatrix}
|
|
err := s.SetWithdrawalQueue([]*enginev1.Withdrawal{})
|
|
assert.ErrorContains(t, "SetWithdrawalQueue is not supported", err)
|
|
})
|
|
}
|
|
|
|
func TestAppendWithdrawal(t *testing.T) {
|
|
t.Run("ok", func(t *testing.T) {
|
|
oldWithdrawal1 := &enginev1.Withdrawal{
|
|
WithdrawalIndex: 0,
|
|
ExecutionAddress: []byte("address1"),
|
|
Amount: 1,
|
|
}
|
|
oldWithdrawal2 := &enginev1.Withdrawal{
|
|
WithdrawalIndex: 1,
|
|
ExecutionAddress: []byte("address2"),
|
|
Amount: 2,
|
|
}
|
|
q := []*enginev1.Withdrawal{oldWithdrawal1, oldWithdrawal2}
|
|
s := BeaconState{
|
|
version: version.Capella,
|
|
withdrawalQueue: q,
|
|
sharedFieldReferences: map[nativetypes.FieldIndex]*stateutil.Reference{nativetypes.WithdrawalQueue: stateutil.NewRef(1)},
|
|
dirtyFields: map[nativetypes.FieldIndex]bool{},
|
|
dirtyIndices: map[nativetypes.FieldIndex][]uint64{},
|
|
rebuildTrie: map[nativetypes.FieldIndex]bool{},
|
|
}
|
|
newWithdrawal := &enginev1.Withdrawal{
|
|
WithdrawalIndex: 2,
|
|
ExecutionAddress: []byte("address3"),
|
|
Amount: 3,
|
|
}
|
|
err := s.AppendWithdrawal(newWithdrawal)
|
|
require.NoError(t, err)
|
|
expectedQ := []*enginev1.Withdrawal{oldWithdrawal1, oldWithdrawal2, newWithdrawal}
|
|
assert.DeepEqual(t, expectedQ, s.withdrawalQueue)
|
|
})
|
|
t.Run("version before Capella not supported", func(t *testing.T) {
|
|
s := BeaconState{version: version.Bellatrix}
|
|
err := s.AppendWithdrawal(&enginev1.Withdrawal{})
|
|
assert.ErrorContains(t, "AppendWithdrawal is not supported", err)
|
|
})
|
|
}
|